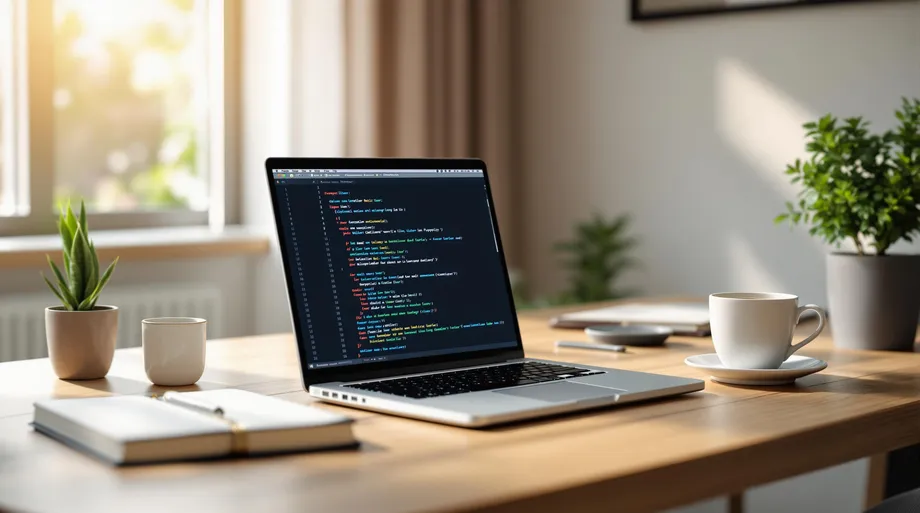
- Harsh Maur
- April 2, 2025
- 10 Mins read
- WebScraping
10 Puppeteer Tips for Efficient Web Scraping
Want to scrape websites faster and more reliably? Puppeteer can help you automate Chrome to extract data efficiently, even from dynamic sites. Here’s what you’ll learn in this guide:
- Optimize performance with browser launch settings and caching.
- Avoid detection using stealth tactics like masking automation traces.
- Speed up scraping by blocking unnecessary resources and running multiple browsers.
- Handle errors with retry mechanisms and smart wait times.
- Improve stability by managing memory and cleaning up browser sessions.
- Track performance to refine your scraping process.
These 10 tips will help you scrape faster, reduce errors, and save resources. Let’s dive into the details.
1. Set Up Browser Launch Options
Fine-tune Puppeteer's launch settings to improve performance and reduce memory usage. Proper configuration ensures smoother operation, especially in resource-constrained environments.
Launch Chrome in headless mode with minimal resource consumption:
const browser = await puppeteer.launch({
headless: true,
args: [
'--no-sandbox',
'--disable-setuid-sandbox',
'--disable-dev-shm-usage',
'--disable-accelerated-2d-canvas',
'--disable-gpu',
'--window-size=1920x1080'
]
});
These options disable features like GPU acceleration and sandboxing, which aren't needed in controlled settings. The --disable-dev-shm-usage
flag is particularly useful for systems with limited shared memory, preventing Chrome from running into memory issues.
You can also add memory-saving configurations for better performance:
const browser = await puppeteer.launch({
defaultViewport: {
width: 1920,
height: 1080
},
ignoreHTTPSErrors: true,
timeout: 30000,
userDataDir: './tmp'
});
The defaultViewport
ensures consistent page rendering dimensions. Enabling ignoreHTTPSErrors
avoids interruptions from SSL certificate errors. A timeout
of 30 seconds helps manage unresponsive connections, while userDataDir
improves cache handling for smoother operation.
For extended or large-scale scraping tasks, enable process management features:
const browser = await puppeteer.launch({
pipe: true,
dumpio: false,
handleSIGINT: true,
handleSIGTERM: true,
handleSIGHUP: true
});
These flags ensure stability by handling system signals and preventing orphan processes during long-running sessions. This setup is ideal for maintaining reliability in demanding scraping workflows.
2. Add Stealth Mode Settings
To enhance your automation's disguise, tweak stealth settings for better concealment.
Here’s how to set them up:
const browser = await puppeteer.launch({
headless: true,
args: [
'--disable-blink-features=AutomationControlled',
'--enable-features=NetworkService',
'--no-first-run',
'--no-default-browser-check',
'--disable-infobars',
'--lang=en-US,en'
]
});
const page = await browser.newPage();
await page.setExtraHTTPHeaders({
'Accept-Language': 'en-US,en;q=0.9',
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/112.0.0.0 Safari/537.36'
});
Randomize actions to appear less automated:
// Add random delays between actions
const randomDelay = (min, max) => {
return Math.floor(Math.random() * (max - min + 1) + min);
};
await page.waitForTimeout(randomDelay(1000, 3000));
// Simulate mouse movements
await page.mouse.move(
Math.random() * page.viewport().width,
Math.random() * page.viewport().height,
{ steps: 10 }
);
Mask automation traces by modifying browser properties:
await page.evaluateOnNewDocument(() => {
delete navigator.__proto__.webdriver;
Object.defineProperty(navigator, 'plugins', {
get: () => [1, 2, 3, 4, 5]
});
Object.defineProperty(navigator, 'languages', {
get: () => ['en-US', 'en']
});
window.chrome = {
runtime: {}
};
});
These adjustments help mimic natural user behavior and eliminate common signs of automation.
3. Control Network Requests
To make your scraping process faster and more efficient, you can manage network requests by intercepting and blocking unnecessary resources. This approach reduces load times and memory usage, complementing optimized browser settings.
Here's how you can block non-essential resources like images, stylesheets, and fonts:
await page.setRequestInterception(true);
page.on('request', (request) => {
// Specify resource types to block
const blockedResources = [
'image',
'stylesheet',
'font',
'media',
'analytics'
];
if (blockedResources.includes(request.resourceType())) {
request.abort(); // Skip loading these resources
} else {
request.continue(); // Allow other requests
}
});
Additionally, you can apply more specific rules to allow critical API calls and JSON responses while blocking analytics, tracking, and third-party resources:
page.on('request', (request) => {
const url = request.url().toLowerCase();
// Allow API calls and JSON responses
if (url.includes('/api/') || url.includes('.json')) {
request.continue();
return;
}
// Block tracking and analytics requests
if (url.includes('google-analytics') ||
url.includes('tracking') ||
url.includes('pixel')) {
request.abort();
return;
}
// Block certain third-party domains
if (url.includes('doubleclick.net') ||
url.includes('facebook.com') ||
url.includes('google-analytics.com')) {
request.abort();
return;
}
// Allow all other requests
request.continue();
});
4. Use Browser Cache
Speed up load times and reduce server requests by making use of browser caching. Here's how you can set up caching with Puppeteer:
const browser = await puppeteer.launch({
userDataDir: './cache-directory',
args: [
'--disk-cache-size=104857600', // Cache size: 100MB
'--disk-cache-dir=./cache'
]
});
Enable caching for pages:
await page.setCacheEnabled(true);
const cacheSettings = {
'document': 3600, // HTML: 1 hour
'script': 86400, // JavaScript: 24 hours
'stylesheet': 86400, // CSS: 24 hours
'image': 604800, // Images: 1 week
'font': 2592000 // Fonts: 30 days
};
// Adjust cache behavior based on resource type
page.on('request', async (request) => {
const url = request.url();
const resourceType = request.resourceType();
const headers = request.headers();
if (url.includes('/api/') || url.includes('/dynamic/')) {
headers['Cache-Control'] = 'no-store';
} else if (cacheSettings[resourceType]) {
headers['Cache-Control'] = `max-age=${cacheSettings[resourceType]}`;
} else {
headers['Cache-Control'] = 'max-age=3600';
}
request.continue({ headers });
});
Keep cache size under control:
const fs = require('fs');
const path = require('path');
function clearCache(directory) {
if (fs.existsSync(directory)) {
fs.rmSync(directory, { recursive: true });
fs.mkdirSync(directory);
}
}
// Clear cache if it exceeds 500MB
const cacheSize = fs.statSync('./cache-directory').size;
if (cacheSize > 524288000) {
clearCache('./cache-directory');
}
With these settings, you can optimize browser performance by reducing both load times and server strain.
5. Run Multiple Browsers
Speed up your scraping process by running multiple browser instances at the same time. The "puppeteer-cluster" library makes it easy to handle several pages simultaneously:
const cluster = require('puppeteer-cluster');
(async () => {
const scrapeCluster = await cluster.launch({
concurrency: cluster.CONCURRENCY_CONTEXT,
maxConcurrency: 4, // Adjust this depending on your system's capacity
monitor: true,
puppeteerOptions: {
headless: true,
args: ['--no-sandbox', '--disable-setuid-sandbox']
}
});
// Define how each URL should be processed
await scrapeCluster.task(async ({ page, data: url }) => {
await page.goto(url);
// Add your scraping logic here
});
// Add URLs to the queue for processing
const urls = ['url1', 'url2', 'url3', 'url4'];
urls.forEach(url => scrapeCluster.queue(url));
await scrapeCluster.idle();
await scrapeCluster.close();
})();
This method allows for parallel processing, improving overall efficiency. Adjust the concurrency level based on your system's memory and processing power. Make sure to close browser instances after each task to manage resources effectively. This approach works well with other performance tweaks you've implemented.
sbb-itb-65bdb53
6. Set Smart Wait Times
Smart wait times help ensure that dynamic content loads properly, avoiding unnecessary delays. Instead of using fixed wait times, these strategies rely on specific page elements or network states to determine when to proceed.
Wait for Key Elements and Network Idle
Use targeted waits to ensure critical elements are ready:
await page.waitForSelector('.product-card', { visible: true, timeout: 5000 });
await page.goto(url, { waitUntil: 'networkidle0', timeout: 30000 });
Custom Wait Function Example
Create a reusable function to handle dynamic content loading:
const waitForDynamicContent = async (page) => {
try {
await page.waitForFunction(() => {
const elements = document.querySelectorAll('.product-card');
return elements.length > 0;
}, { polling: 100, timeout: 10000 });
} catch (error) {
console.error('Content load failed:', error);
}
};
Combine Multiple Wait Conditions
Sometimes, you need to wait for several conditions simultaneously:
async function smartWait(page) {
await Promise.all([
page.waitForSelector('#main-content', { visible: true }),
page.waitForFunction(() => document.readyState === 'complete')
]);
}
Handle Infinite Scrolling
For pages with infinite scrolling, use a loop to load content dynamically:
async function scrollWithSmartWait(page) {
let previousHeight = 0;
while (true) {
await page.evaluate(() => window.scrollTo(0, document.body.scrollHeight));
await page.waitForTimeout(1000);
const currentHeight = await page.evaluate(() => document.body.scrollHeight);
if (currentHeight === previousHeight) {
break;
}
previousHeight = currentHeight;
}
}
These techniques help streamline how you handle dynamic content, ensuring pages load efficiently without unnecessary delays.
7. Write Better CSS Selectors
Good CSS selectors can make Puppeteer more efficient and reliable. Refining your selectors is key to improving scraping performance.
Use Specific Attribute Selectors
Leverage unique data attributes for precise targeting:
await page.$('[data-product-id="123"]');
await page.$('[data-testid="price-value"]');
Adding structure to your selectors can further improve their performance.
Optimize Selector Performance
Here's how different approaches affect performance:
// Slower: full DOM traversal
await page.$('.product-name');
// Faster: scoped search
await page.$('#product-section .product-name');
// Best: direct ID selector
await page.$('#product-title');
Using direct and scoped selectors reduces overhead and speeds up operations.
Handle Dynamic Classes
Dynamic class names can be tricky. Use these strategies to manage them effectively:
// Match partial class names
await page.$('[class*="price-container"]');
// Combine attributes for better accuracy
await page.$('div[class*="product"][data-type="listing"]');
This ensures your selectors remain functional even when class names change.
Implement Smart Fallbacks
Fallbacks can make your selectors more robust:
async function smartSelect(page, selectors) {
for (const selector of selectors) {
const element = await page.$(selector);
if (element) return element;
}
throw new Error('No matching element found');
}
// Usage example
const element = await smartSelect(page, [
'#main-price',
'[data-price]',
'.price-container span'
]);
This method ensures your script can adapt if a primary selector fails.
XPath Alternative
XPath can be a powerful alternative to CSS selectors:
// Find elements with specific text
await page.$x('//div[contains(text(), "In Stock")]');
// Locate elements with multiple conditions
await page.$x('//div[@class="product" and @data-available="true"]');
Validate Selectors Before Deployment
Always test your selectors to confirm they work as expected:
await page.evaluate((selector) => {
const elements = document.querySelectorAll(selector);
console.log(`Found ${elements.length} elements`);
return Array.from(elements).map(el => el.outerHTML);
}, '.product-card');
8. Set Up Error Recovery
To keep your scraping process running smoothly, it's important to handle errors effectively. A retry mechanism can help by automatically attempting failed operations caused by temporary issues.
Here's a retry wrapper you can use to manage these situations:
async function withRetry(fn, maxRetries = 3, delay = 1000) {
let lastError; // Stores the most recent error
for (let attempt = 1; attempt <= maxRetries; attempt++) {
try {
return await fn();
} catch (error) {
lastError = error;
console.log(`Attempt ${attempt} failed: ${error.message}`);
if (attempt < maxRetries) {
await new Promise(r => setTimeout(r, attempt * delay));
}
}
}
throw lastError;
}
// Example usage
await withRetry(async () => {
const element = await page.$('#dynamic-content');
return await element.evaluate(el => el.textContent);
});
This approach helps you handle temporary errors by retrying operations instead of failing outright.
9. Clean Up Browser Memory
Good memory management is key to keeping Puppeteer scrapes stable and running smoothly over time. By using cleanup strategies, you can avoid memory leaks and crashes.
Start by ensuring you close pages properly after each scraping session:
async function scrapePage(url) {
const page = await browser.newPage();
try {
await page.goto(url);
// Your scraping logic here
return data;
} finally {
await page.close();
}
}
Reduce memory usage further by clearing the cache and cookies:
async function clearBrowserData(page) {
const client = await page.target().createCDPSession();
await client.send('Network.clearBrowserCache');
await client.send('Network.clearBrowserCookies');
}
For high-volume scraping, consider recycling the browser instance after processing a set number of pages:
async function recycleBrowser(maxPages = 100) {
let pageCount = 0;
return async function() {
pageCount++;
if (pageCount >= maxPages) {
await browser.close();
browser = await puppeteer.launch(options);
pageCount = 0;
}
return browser;
};
}
Keep an eye on memory usage and restart the browser automatically if it gets too high:
async function checkMemoryUsage(page) {
const metrics = await page.metrics();
const jsHeapSize = metrics.JSHeapUsedSize / 1024 / 1024; // Convert to MB
if (jsHeapSize > 1024) { // Restart if memory exceeds 1GB
await browser.close();
browser = await puppeteer.launch(options);
}
}
Finally, make sure to clean up event listeners and intervals when they’re no longer needed:
// Remove all event listeners
page.removeAllListeners();
// Clear any running intervals
clearInterval(checkMemoryInterval);
10. Track Scraping Speed
Tracking scraping speed is crucial for confirming that your optimizations actually deliver results. By monitoring performance, you can identify bottlenecks and fine-tune your Puppeteer scripts for better efficiency.
Here’s a sample script to measure scraping speed:
async function measureScrapingSpeed() {
const startTime = performance.now();
const metrics = {
pageLoad: 0,
extraction: 0,
processing: 0
};
const page = await browser.newPage();
try {
// Measure page load time
const loadStart = performance.now();
await page.goto(url); // Ensure 'url' is defined
metrics.pageLoad = performance.now() - loadStart;
// Measure data extraction time
const extractStart = performance.now();
const data = await page.evaluate(() => {});
metrics.extraction = performance.now() - extractStart;
// Track processing duration
const processStart = performance.now();
await processData(data);
metrics.processing = performance.now() - processStart;
return {
totalTime: performance.now() - startTime,
metrics
};
} finally {
await page.close();
}
}
In addition to timing, keeping an eye on resource usage can provide deeper insights into performance:
async function monitorResources(page) {
const resources = {
requests: 0,
dataTransferred: 0
};
page.on('request', request => {
resources.requests++;
});
page.on('response', response => {
const length = response.headers()['content-length'];
resources.dataTransferred += parseInt(length || 0);
});
return resources;
}
Logging and Analyzing Metrics
Save performance data to a file for later analysis or visualization on a dashboard:
const fs = require('fs').promises;
async function logPerformance(metrics) {
const timestamp = new Date().toISOString();
const log = {
timestamp,
...metrics,
memoryUsage: process.memoryUsage().heapUsed / 1024 / 1024
};
await fs.appendFile('scraping-metrics.json',
JSON.stringify(log) + '\n'
);
}
Rolling Metrics for Long Tasks
For tasks that run over extended periods, calculating rolling averages can help you monitor trends:
function calculateRollingMetrics(window = 100) {
let times = [];
return (newTime) => {
times.push(newTime);
if (times.length > window) {
times.shift();
}
return {
average: times.reduce((a, b) => a + b) / times.length,
min: Math.min(...times),
max: Math.max(...times)
};
};
}
Conclusion
Effective web scraping with Puppeteer depends on optimizing performance, managing resources wisely, and handling errors effectively. These strategies create a solid base for scalable and reliable scraping operations.
Web Scraping HQ simplifies complex tasks like browser setup, error recovery, HTML parsing, proxy handling, and CAPTCHA bypassing. This allows businesses to focus on using the data rather than worrying about how to collect it. Their automated tools make the entire data extraction process smoother.
"Working with WebScrapingHQ has substantially improved our data operations. Their rigorous QA process ensures that the data we receive meets the highest standards." - Alex Simmons, Data Manager at an e-commerce company
The platform offers flexible data delivery options, including CSV, JSON, JSONLines, and XML formats, along with delivery methods like FTP, SFTP, AWS S3, and Google Cloud Storage. This makes it easy to integrate with existing workflows.
Experts in the field also highlight the platform’s reliability. Research Analyst Priya Nair shares:
"I've found WebScrapingHQ invaluable for real estate data extraction. They are dependable, efficient, and always deliver on time."
Whether you choose to apply these tips yourself or rely on managed scraping services, tracking key metrics like load times, extraction speeds, and resource usage is essential for keeping operations efficient. Success in web scraping requires a balance of technical precision, legal compliance, and quality control. A structured approach - whether handled in-house or outsourced - ensures consistent, long-term results.