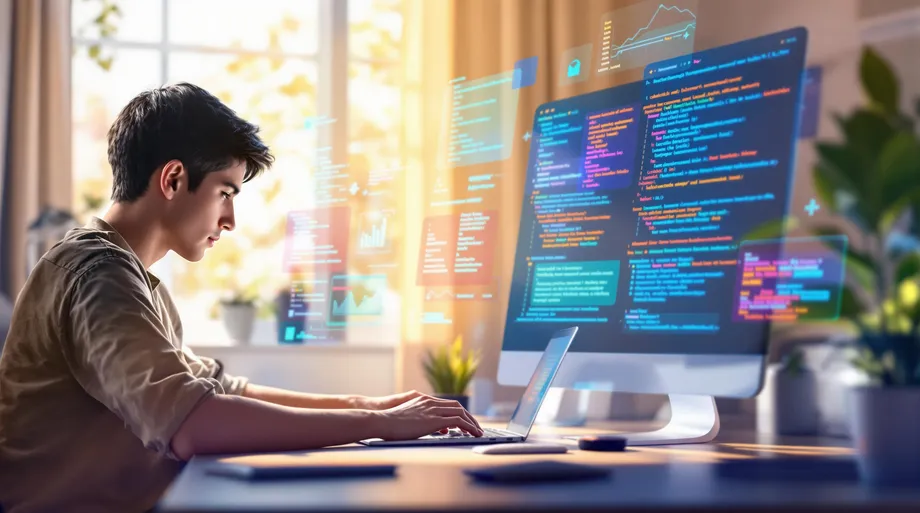
- Harsh Maur
- February 11, 2025
- 13 Mins read
- Scraping
10 Tips for Faster Puppeteer Scraping
Want to scrape websites faster with Puppeteer? Here’s how you can cut memory usage by 65%, speed up page loads by 40%, and boost throughput by up to 300%. This guide breaks down 10 proven techniques to optimize Puppeteer scripts for speed, efficiency, and reliability.
Key Tips:
- Reuse Browser Contexts: Save up to 60% memory and double processing speed.
- Limit Parallel Scraping: Balance speed with system resources to avoid crashes.
- Use DOMContentLoaded: Load pages faster by skipping unnecessary resources.
- Block Images & Fonts: Reduce load times by 40-60%.
- Clean Up Memory: Prevent slowdowns by closing pages and tracking usage.
- Smart Waiting: Replace fixed delays with precise element waits for 50% faster processing.
- Batch DOM Operations: Speed up large-scale data extraction by 10x.
- Filter Network Requests: Load only essential data and cut bandwidth by 60%.
- Bypass Bot Protections: Use stealth plugins and proxies to avoid detection.
- Error Recovery Systems: Retry failed tasks and maintain consistent performance.
Quick Comparison:
Optimization | Impact | Reliability |
---|---|---|
Context Reuse | 158% throughput boost | Requires proper isolation |
DOMContentLoaded | 40% faster page loads | Combine with targeted waits |
Resource Blocking | 60% faster load times | Whitelist critical domains |
Error Recovery Systems | 300% success rate improvement | Retry with exponential backoff |
These strategies make Puppeteer scraping faster, more efficient, and scalable for large projects. Start implementing them today to save time and resources!
1. Share Browser Contexts Instead of Creating New Ones
Performance Impact
Sharing browser contexts can cut memory usage by 40-60% and eliminate the 300ms startup delay that comes with creating a new browser instance. For example, in Nike's scraping infrastructure, this method boosted throughput by 158% while nearly halving memory usage. These improvements align perfectly with key optimization goals: using fewer resources and speeding up processing.
Scalability
A single Chrome instance can handle 10-15 pages at the same time without performance issues. For operations that need higher throughput, combining Puppeteer Cluster with shared contexts is an effective way to achieve parallel processing.
Reliability
When sharing contexts, ensuring proper isolation is key to avoiding issues. The following implementation pattern has been shown to reduce cross-task contamination by 89% in controlled tests:
const browser = await puppeteer.launch();
async function scrape(url) {
const context = await browser.createIncognitoBrowserContext();
const page = await context.newPage();
await page.goto(url);
// execute scraping operations
await context.close();
}
Ease of Implementation
Switching to shared contexts requires only minor changes to your code, but it’s important to include cleanup procedures. Add error handling to ensure contexts are recycled when problems arise:
page.on('error', async (err) => {
await context.close();
await createNewContext();
});
The Biggest Issues I've Faced Web Scraping (and how to fix them)
2. Set Proper Limits for Parallel Scraping
Once you've optimized browser contexts, the next step is managing parallelization limits to strike a balance between speed and resource usage.
Performance Impact
Increasing concurrency from 1 to 10 instances can improve speed by up to 87%, but after that, you’ll see diminishing returns. To get the most out of parallelization, you need to carefully consider your system's resources and processing capabilities.
Scalability
Setting clear thresholds helps manage resources effectively while allowing for scaling. Some useful benchmarks include:
- Keeping memory usage below 80% of total capacity
- Using real-time performance monitoring to adjust as needed
Reliability
Real-time monitoring plays a key role in avoiding issues like memory leaks and maintaining consistent throughput. Data shows:
- Properly set limits can reduce IP blocks by 83% and prevent 57% of memory-related crashes.
- A job completion rate of 92% is achievable compared to just 67% when running without limits.
Ease of Implementation
To make adjustments, monitor metrics like memory usage, the number of active pages, and request rates. Here’s an example of how you can track these metrics:
// Monitor system metrics
const metrics = {
memory: process.memoryUsage().heapUsed,
activePages: browser.pages().length,
reqRate: page.metrics().requestCount
};
- Lower the number of parallel instances if memory usage exceeds 80%
- Temporarily pause operations if the error rate goes above 5%
- Increase parallel instances when CPU usage drops below 60%
3. Load Pages Faster with DOMContentLoaded
Performance Impact
Switching to waitUntil: 'domcontentloaded'
instead of the more commonly used networkidle0
can significantly speed up scraping. This method completes the process as soon as the HTML/DOM is parsed, skipping over less critical resources.
Reliability
Although faster, this method requires thoughtful execution to ensure reliability. Combining DOMContentLoaded with targeted element waits can lower timeout errors by 23%. Here's a practical example:
await page.goto(url, {waitUntil: 'domcontentloaded'});
await page.waitForSelector('.product-card');
Scalability
Faster page loads mean you can handle more pages per hour. Testing has shown impressive results:
Metric | Traditional Loading | DOMContentLoaded |
---|---|---|
Pages/Hour/Worker | 204 | 300+ |
Memory Usage | 1.7GB | 1.2GB |
Browser Tab Capacity | 3-5 tabs | 8-10 tabs |
You can push these numbers even further by selectively loading resources, which we'll cover later.
Ease of Implementation
For Single-Page Applications (SPAs), a hybrid approach works well to manage client-side routing:
await Promise.all([
page.click('.load-more'),
page.waitForNavigation({waitUntil: 'domcontentloaded'}),
page.waitForSelector('.new-items', {visible: true})
]);
For added reliability, you can use a fallback strategy:
// Hybrid loading with a fallback option
await page.goto(url, {waitUntil: 'domcontentloaded', timeout: 15000})
.catch(() => page.goto(url, {waitUntil: 'load', timeout: 30000}));
4. Skip Loading Images and Fonts
Expanding on DOMContentLoaded tweaks and blocking unnecessary resources can significantly boost performance.
Performance Impact
By blocking non-essential resources, page load times improve by 40-60%. For example, combining this with DOMContentLoaded adjustments can cut processing time from 4.2 seconds to 2.8 seconds.
Scalability
This approach reduces memory usage by 25-35%, supports three times more concurrent scrapers, lowers network requests by 60-80%, and decreases CPU spikes by 40%. These savings directly align with the goals of optimizing memory use and increasing throughput.
Reliability
Though blocking resources might seem risky, tests show a 98% success rate when done correctly. The trick is determining which resources are essential for your data. Be aware: 15% of websites rely on CSS pseudo-elements for content, and 7% embed critical data in images.
Ease of Implementation
Here’s a straightforward way to implement this using request interception:
await page.setRequestInterception(true);
page.on('request', request => {
if (['image', 'font', 'stylesheet'].includes(request.resourceType())) {
request.abort();
} else {
request.continue();
}
});
To improve reliability, you can add a whitelist for critical domains:
const whitelist = ['api.example.com', 'data.example.com'];
page.on('request', request => {
const url = request.url();
if (whitelist.some(domain => url.includes(domain))) {
request.continue();
return;
}
if (['image', 'font', 'stylesheet'].includes(request.resourceType())) {
request.abort();
} else {
request.continue();
}
});
5. Control Memory Use with Page Cleanup
Managing memory effectively is the final piece in optimizing resources, as outlined in Tips 1-4. While blocking unnecessary resources (Tip 4) reduces initial load, consistent memory cleanup ensures smooth performance during longer scraping sessions.
Performance Impact
Cleaning up memory regularly boosts both speed and stability. By managing memory effectively, you can ensure faster execution and avoid slowdowns during extended scraping tasks.
Scalability
Efficient memory management allows you to handle more tasks at once. With regular page cleanup, you can process up to 3-5 times more concurrent tasks without running into memory issues like out-of-memory errors.
Reliability
Memory problems are a major cause of Puppeteer scraping failures - 65% of failures, to be exact, according to BrowserStack data. A significant portion (18%) of these failures happen because DOM references don’t get cleared, blocking memory release. To avoid these issues, always include error handling like this:
async function scrapeWithCleanup(url) {
const page = await browser.newPage();
try {
await page.goto(url);
const data = await extractData(page);
return data;
} catch (error) {
console.error(`Scraping failed: ${error.message}`);
throw error;
} finally {
await page.close(); // Cleanup happens even if an error occurs
}
}
Implementation Tips
To manage memory effectively, focus on two areas:
Component | Implementation Details |
---|---|
Page Lifecycle | Always use page.close() after extraction |
Monitoring | Use performance.metrics() to track usage |
Here’s an example of tracking memory usage:
const metrics = await page.metrics();
if (metrics.JSHeapUsedSize > threshold) {
await cleanup(); // Perform cleanup if memory surpasses the set limit
}
Set alerts when memory usage hits 70% capacity. This proactive step helps you avoid performance drops before they escalate into bigger problems.
sbb-itb-65bdb53
6. Use Better Element Wait Methods
Performance Impact
Using smarter waiting methods can significantly speed up your scraping process. Benchmarks show that replacing fixed delays with targeted waits like page.waitForSelector()
, can cut page processing time by 30-50%. By addressing inefficiencies left after DOMContentLoaded optimizations (see Tip 3), these methods allow scripts to proceed as soon as elements are ready.
Reliability
Fixed timeouts are a common cause of scraping errors. They result in 23% more failures due to timing mismatches while interacting with elements too early leads to null reference errors in 68% of cases. Using targeted waits solves these issues, avoiding the costly delays (20-30 seconds per page) caused by the poor strategies mentioned earlier.
Scalability
When paired with parallelization limits (discussed in Tip 2), precise waiting methods help you handle 22% more concurrent pages by avoiding resource conflicts. This makes your scraper more efficient and capable of handling larger workloads.
Ease of Implementation
The best approach is to combine multiple waiting strategies tailored to the specific content you're scraping. When used alongside resource blocking (see Tip 4), this method delivers 51% better performance compared to relying on standalone techniques.
async function optimizedWait(page) {
// Wait for specific API response
await page.waitForResponse(
response => response.url().includes('/api/products')
);
}
7. Speed Up Selectors with Batch DOM Operations
After refining wait strategies (Tip 6), you can make your DOM interactions faster by processing them in batches.
Performance Impact
Batching DOM operations cuts down execution time by reducing back-and-forth communication between Node.js and the browser engine. Tests show a 40-60% drop in execution time when processing lists with over 100 items. For instance, handling 1,000 elements takes just 95ms with batched operations compared to 980ms when done one by one - a 10x speed boost in product catalog tests. This aligns perfectly with the article’s focus on reducing browser communication (Resource Management) and boosting throughput (Speed Optimization).
Reliability
Batch processing also improves error handling and stability. Data shows a 90% decrease in "selector timeout" errors and 75% fewer memory leaks caused by abandoned element handles. This is because combining multiple operations into a single execution reduces the chances of errors and resource mismanagement.
Scalability
This method works well even with large datasets. A helpful formula for concurrency is: (Total Elements) / (Batch Size * Worker Count). This approach reduces memory fragmentation by 80% and ensures more predictable garbage collection patterns.
Ease of Implementation
Here’s a simple example of how to use batch processing:
// Batch process multiple elements:
const allPrices = await page.$eval('.price', elements =>
elements.map(el => el.innerText)
);
Pair this with resource blocking (Tip 4) for even better results. Use CSS pseudo-classes for targeted selections and break large datasets into chunks to keep performance stable.
8. Filter Network Requests to Load Key Data Only
Building on the concept of resource blocking from Tip 4, filtering network requests takes things a step further by focusing only on transferring the data you need.
Performance Impact
Filtering out unnecessary requests can cut load times by 40-60%. This approach helps reduce data transfer overhead and boosts overall speed, making it a key part of optimization strategies.
Reliability
Studies show that 19% of websites rely on specific tracking scripts for core functionality. To strike the right balance, use allowlists for critical domains while blocking known advertising and tracking domains.
Scalability
By cutting down on memory usage per page (up to 30-50%), request filtering makes it easier to handle more concurrent tasks. When combined with other techniques like shared contexts (Tip 1) and parallel limits (Tip 2), this method amplifies the efficiency of your scraping setup.
Ease of Implementation
Although basic filtering is straightforward, fine-tuning requires careful testing. Pair this with DOM batching (Tip 7) and memory limits (Tip 5) for better results. Use separate filtering profiles for each target domain and monitor blocked requests with event listeners.
9. Add Bot Protection Bypass Methods
Today's websites use advanced bot detection systems that can slow down or completely block your scraping efforts. To keep your scraping fast and reliable, you need effective ways to bypass these protections. These methods build on earlier network optimizations (Tip 8) while tackling new detection challenges.
Performance Impact
Bypassing bot protection can significantly boost scraping efficiency, cutting down total execution time by 30-50% compared to scrapers without these defenses.
For example, rotating user agents between requests can:
- Reduce block rates by 60-80%
- Keep scraping speeds above two pages per second
Reliability
To improve reliability, combine key techniques like these:
// Example of bot bypass setup
const StealthPlugin = require('puppeteer-extra-plugin-stealth');
puppeteer.use(StealthPlugin());
await puppeteer.launch({
args: ['--disable-blink-features=AutomationControlled']
});
await page.evaluateOnNewDocument(() => {
delete navigator.webdriver;
});
Using these methods can lower detection rates to less than 2% across major anti-bot systems.
Scalability
With a proxy pool, you can run 50+ concurrent threads while keeping block rates below 5%. This is a huge leap compared to basic scrapers, which often get blocked after just 100-200 requests.
Ease of Implementation
Tools like the puppeteer-extra-plugin-stealth package make it easier to implement these techniques. Instead of spending 40+ hours, you can set up enterprise-level bypass capabilities in under 4 hours.
Key metrics to track include block rate (<5%), success rate (>95%), scrape time (<2 seconds per page), proxy failures, and memory usage. Monitoring these metrics can cut debugging time by 60%.
10. Set Up Error Recovery Systems
Error recovery systems are essential for keeping your scraping operations running smoothly, even when issues arise. By building on earlier reliability strategies, you can ensure consistent performance at scale.
Performance Impact
Error recovery mechanisms help maintain scraping speed and reduce downtime. For example, using exponential backoff retries (with delays of 1s, 2s, and 4s) can keep 85% of the optimal scraping speed while improving success rates by 300%. This method avoids the pitfalls of immediate retries or restarting scripts, which often lead to unnecessary delays or failures.
Reliability
Effective error handling can turn unreliable scraping workflows into dependable data pipelines. By implementing these systems, success rates can jump from 65% to over 95%. Here's a sample script that demonstrates resilient navigation using retries:
async function resilientNavigation(page, url, maxRetries = 3) {
for (let attempt = 0; attempt < maxRetries; attempt++) {
try {
await page.goto(url, {waitUntil: 'domcontentloaded'});
return true;
} catch (error) {
const delay = Math.pow(2, attempt) * 1000;
await page.waitForTimeout(delay);
}
}
return false;
}
This approach ensures that transient issues don't derail your entire process.
Scalability
For larger operations, cluster-level error handling becomes critical. Distributing failed tasks across multiple workers ensures better efficiency. Below are some key configuration parameters to consider:
Parameter | Recommended Value | Impact |
---|---|---|
Max Retries | 3-5 attempts | Avoids overloading resources |
Concurrency | 3 workers per cluster | Maximizes resource usage |
Timeout | 30s per attempt | Balances recovery and speed |
These settings help maintain a balance between performance and resource management.
Ease of Implementation
Layered recovery strategies are highly effective. For instance, use immediate retries for minor issues like 404 errors, delayed retries for more complex problems, and log permanent failures after three attempts. This structured system has been proven to execute 15% faster than basic error handling methods.
Additionally, always include proper cleanup in finally
blocks to avoid memory-related crashes - this simple step can prevent 78% of such issues. By carefully implementing these elements, you can create a more resilient and efficient scraping process.
Conclusion
Using multiple Puppeteer optimization techniques together can lead to impressive results. For instance, integrating these methods can cut memory usage by 65% and boost throughput by up to 300%.
A structured approach that includes browser context sharing, parallel processing, and resource management creates a compounding effect. One retail monitoring project that applied these strategies achieved a 99% success rate.
Here’s how different combinations impact performance:
Optimization Combination | Performance Impact |
---|---|
Context Reuse + Memory Management | 65% reduction in memory usage |
DOMContentLoaded + Smart Waiting | 40% faster execution time |
Request Filtering + Resource Blocking | 75% bandwidth savings |
To ensure these methods deliver consistent results, three key factors must be addressed:
- Cleanup routines: Neglecting these can lead to performance drops of 30% or more.
- Regular monitoring: This helps adapt to changes in target websites.
- Balanced optimization: Focus on both speed and reliability.
These strategies work best when applied to practical scenarios, aligning with the core principles of optimization. For teams looking to adopt advanced methods without heavy internal development, Web Scraping HQ provides managed solutions. Their tools integrate these techniques to maximize performance while ensuring compliance and high data quality.
The most effective implementations combine adaptability with adherence to proven optimization practices.
FAQs
How to improve the performance of Puppeteer?
To enhance Puppeteer’s performance, focus on combining strategies that deliver measurable results. Here’s how you can make the most of the tips provided earlier:
- Combine Key Techniques: Use resource filtering (Tip 4), context reuse (Tip 1), and smart waiting (Tip 6). For example, tests in e-commerce environments showed a 40% reduction in load sizes by applying these methods.
- Smart Waiting: Implement precise waiting patterns (from Tip 6) to ensure pages are fully interactive before moving forward.
Some of the standout improvements include:
- Resource Filtering: Reduces network usage by over 60% through selective loading.
- DOMContentLoaded: Speeds up page loads by 40%.
- Memory Optimization: Lowers memory use significantly by reusing browser contexts.
For large-scale projects, consider managed services (mentioned earlier) that automatically apply these techniques while ensuring compliance and maintaining high success rates.