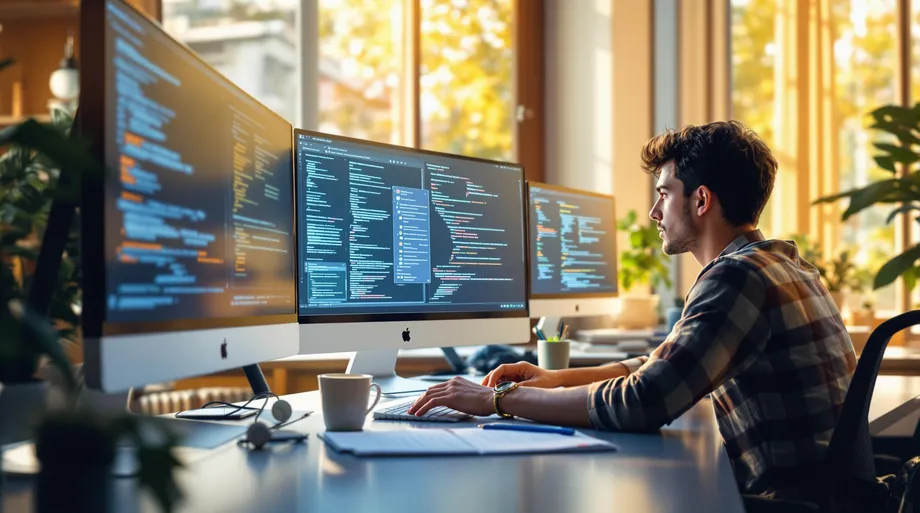
- Harsh Maur
- November 17, 2024
- 13 Mins read
- Scraping
7 Common Web Scraping Errors and Their Solutions
Web scraping can be tricky, but knowing how to handle common errors keeps your data flowing. Here's a quick guide to 7 major scraping pitfalls and how to fix them:
- HTTP 403 Forbidden: Use rotating IPs and varied user agents
- HTTP 429 Too Many Requests: Add delays between requests
- Network Timeouts: Set longer timeouts, retry with backoff
- CAPTCHA Challenges: Use CAPTCHA solvers or mimic human behavior
- Dynamic Content Issues: Switch to headless browsers like Puppeteer
- Data Extraction Errors: Update scripts often, use robust parsing
- Login Problems: Handle cookies and CSRF tokens properly
The key is to make your scraper act human. Rotate IPs, add random delays, and use realistic user agents. For tough cases, tools like Bright Data's Web Unlocker can help bypass blocks.
Related video from YouTube
HTTP Status Code Errors
Web scraping often hits snags with HTTP status code errors. Let's focus on two common ones: 403 Forbidden and 429 Too Many Requests.
Fixing 403 Forbidden Errors
A 403 Forbidden error is like a digital "Keep Out" sign. Websites throw this up when they think you're not a real user.
Here's how to sneak past:
- Switch up your user agents: Make your scraper look like different browsers.
- Use rotating proxies: Change your IP address often.
- Tweak your headers: Add headers a real browser would use.
- Deal with Cloudflare: Use tools like ScrapeOps' Cloudflare Bypass if needed.
"Rotating IP addresses and setting proper HTTP headers (especially User-Agents) can help you dodge detection on most websites." - Web Scraping Expert
Handling 429 Request Limits
A 429 Too Many Requests error means "Slow down!" You've made too many requests too quickly.
To get around this:
- Add delays: Put pauses between requests.
- Use exponential backoff: Wait longer between retries if you hit a 429.
- Watch rate limits: Some APIs tell you how many requests you have left.
- Spread out requests: Use multiple IPs or time periods.
Here's a Python example for handling 429 errors:
import httpx
import asyncio
async def fetch_with_retry(url, retries=3):
async with httpx.AsyncClient() as client:
for attempt in range(retries):
response = await client.get(url)
if response.status_code == 200:
return response.json()
if response.status_code == 429:
retry_after = int(response.headers.get("Retry-After", 5))
print(f"Rate limited. Waiting {retry_after} seconds.")
await asyncio.sleep(retry_after)
else:
response.raise_for_status()
raise Exception("Max retries reached")
async def main():
url = "https://api.example.com/data"
try:
result = await fetch_with_retry(url)
print("Data fetched successfully:", result)
except Exception as e:
print(f"Failed to fetch data: {e}")
asyncio.run(main())
This script catches 429 errors, respects the Retry-After
header, and retries.
"Your scraper should act like a regular user browsing the website." - ZenRows
The key? Make your bot act human. Slow down, mix things up, and you'll have better luck scraping.
Network Problems
Web scraping can hit some bumps in the road. Let's look at two common issues: timeouts and DNS errors, and how to keep your data collection on track.
Fixing Timeouts and DNS Errors
Timeouts happen when servers take too long to respond. DNS errors? That's when your scraper can't find the right address for a website.
Here's how to deal with these:
1. Retry, retry, retry
Don't give up after one failed attempt. Set up your scraper to try again. Here's a simple Python example:
def initialScrape(link):
try:
scrape(link)
except:
return initialScrape(link)
This code keeps trying until it works. But be careful - you don't want to accidentally overwhelm the target site!
2. Have backup servers ready
If one server's acting up, switch to another. This works great for big scraping jobs.
3. Tweak your timeout settings
Sometimes servers are just slow. Give them more time to respond:
resp = requests.get("https://example.com", timeout=10)
This code waits 10 seconds before giving up.
4. Check DNS manually
If you think DNS is the problem, use tools like nslookup
or dig
to check the IP address yourself. If the DNS is wrong, you can use the IP directly.
Solving Proxy Issues
Proxies hide your scraper's identity, but they can cause problems too. Here's how to use them well:
1. Switch proxies often
Don't stick with one proxy for too long. As Raluca Penciuc from WebScrapingAPI says:
"By rotating proxies, we mean the process of automatically switching from one IP to another with each request sent."
This helps you avoid getting caught and access content from different locations.
2. Use a proxy rotation system
Keep track of how well each proxy is working. If one starts to slow down, stop using it until it's back to normal. This keeps your scraping smooth and fast.
3. Pick the right proxy for the job
Different scraping tasks need different proxies:
- Residential proxies: Good for looking like a real user
- Datacenter proxies: Faster, but easier to spot
- Mobile proxies: Great for mobile-specific content
4. Keep an eye on your proxies
Check your proxies regularly. A slow proxy can be worse than no proxy at all.
Data Extraction Errors
Web scraping isn't always smooth sailing. Dynamic pages and missing elements can throw a wrench in your plans. Let's look at how to handle these tricky situations.
Getting Data from Dynamic Pages
Dynamic pages load content on the fly, often using JavaScript. This can leave traditional scrapers scratching their heads.
Tools like Selenium and Puppeteer are your go-to for scraping dynamic content. They interact with web pages just like a real user would.
Let's say you're scraping an e-commerce site that loads product listings as you scroll. Here's how you might do it with Selenium:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
driver = webdriver.Chrome()
driver.get("https://dynamic-ecommerce-site.com")
# Wait for product elements to load
products = WebDriverWait(driver, 10).until(
EC.presence_of_all_elements_located((By.CLASS_NAME, "product-item"))
)
# Scroll to load more products
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
# Extract product data
for product in products:
name = product.find_element(By.CLASS_NAME, "product-name").text
price = product.find_element(By.CLASS_NAME, "product-price").text
print(f"Product: {name}, Price: {price}")
driver.quit()
This script waits for products to load, scrolls to trigger more loading, and then grabs the data. It's like having a robot do your shopping!
Pro tip: Always use WebDriverWait
to make sure elements are loaded before you try to grab them. It's the difference between getting data and getting errors.
Dealing with Missing Content
Websites change. One day your scraper is humming along, the next it's throwing errors because that crucial div you were targeting has vanished.
Here's how to roll with the punches:
- Use flexible selectors: Instead of specific IDs that might change, use more general class names or data attributes.
- Implement error handling: Wrap your extraction code in try-except blocks to handle missing elements gracefully.
- Regular audits: Set up automated checks to alert you when your scraper starts returning weird results.
Here's a practical example of handling missing content:
def extract_product_info(product_element):
try:
name = product_element.find_element(By.CLASS_NAME, "product-name").text
except:
name = "N/A"
try:
price = product_element.find_element(By.CLASS_NAME, "product-price").text
except:
price = "Price not available"
return {"name": name, "price": price}
# Use this function in your main scraping loop
products_info = [extract_product_info(product) for product in products]
This approach ensures you get some data even if certain elements are missing, rather than crashing your entire scraping operation.
Web scraping is often a game of adaptation. As Jose Luis Fernández, a Data Scientist, puts it:
"In this article, we have learned to deal with the values lost in the extraction of data from an html code due to a lack of tags. Something relatively easy to solve, but that took me a while to solve, so, if readers can save a while, it will be great!!!"
Keep these tips in mind, and you'll be scraping like a pro in no time!
sbb-itb-65bdb53
CAPTCHA Problems
CAPTCHAs are like digital bouncers, keeping bots out and letting humans in. For web scrapers, they're a major headache. Let's look at these puzzles and how to crack them.
Types of CAPTCHAs
CAPTCHAs come in various forms:
- Text-based CAPTCHAs: Squiggly letters and numbers.
- Image-based CAPTCHAs: Pick specific objects from a grid of images.
- reCAPTCHA v2: Google's "I'm not a robot" checkbox.
- hCaptcha: Similar to reCAPTCHA, but with its own flavor.
- Audio CAPTCHAs: Listen and type out numbers or letters.
These are meant to be easy for humans but tough for bots. When you're scraping at scale, even "easy" becomes a big problem.
Getting Past CAPTCHAs
How can we outsmart these digital gatekeepers? Here are some tactics:
1. CAPTCHA Solving Services
These services use real people to solve CAPTCHAs for you. 2Captcha and AntiCaptcha are popular options.
"CAPTCHA solvers are key tools for web scraping, boosting automation and efficiency of your projects."
But they're not free. Most charge between $0.5 to $3 per 1,000 CAPTCHAs solved. You'll need to weigh the costs and benefits for your project.
2. Smart Proxies and IP Rotation
Websites often show CAPTCHAs when they see too many requests from one IP. Rotating IP addresses can help you stay under the radar.
Bright Data's Web Unlocker, for example, offers a proxy setup that can bypass various CAPTCHA types with a 99.9% success rate.
3. Mimic Human Behavior
CAPTCHAs pop up when they spot bot-like behavior. So, act human:
- Add random delays between requests
- Simulate mouse movements
- Follow natural browsing patterns
4. Use Headless Browsers
Tools like Selenium or Puppeteer can interact with websites like a real browser, making it harder for sites to spot your scraper.
5. Machine Learning Approaches
For image-based CAPTCHAs, you can train ML models to recognize and solve them. It's not perfect, but it can work for some types.
The main goal is to stop CAPTCHAs from showing up in the first place. As one expert says:
"The best approach is to prevent CAPTCHAs from appearing initially and, if blocked, to retry the request."
By mixing these methods, you can cut down on CAPTCHAs and make your scraping smoother.
Keep in mind that CAPTCHA tech is always changing, so your bypass strategies need to evolve too. Stay updated, get creative, and happy scraping!
Request Limit Problems
Web scraping at scale can hit request limit snags. Websites set these limits to guard their servers and data. Here's how to dodge blocks and keep scraping:
Avoiding IP Blocks
Make your scraper act human, not bot-like. Try these:
1. IP rotation
Don't use one IP for all requests. That's a block magnet. Instead, use an IP pool and rotate. It spreads out requests, making them look like they're from different sources.
Bright Data's Web Unlocker, for instance, offers a proxy setup that sidesteps various blocks with a 99.9% success rate. It auto-rotates IPs, making your scraper harder to spot.
2. Human-like behavior
Websites are getting better at sniffing out bots. To fly under the radar:
- Add random delays between requests
- Mix up your request patterns
- Use realistic user agents
Here's a quick Python example for random delays:
import time
import random
def make_request(url):
# Your request code here
time.sleep(random.uniform(1, 5)) # Random 1-5 second delay
3. Mind the robots.txt
Don't ignore the robots.txt file. It's a rookie mistake. This file lays out scraping dos and don'ts. Following it helps you avoid looking like a bad bot.
4. Keep sessions consistent
Stick to the same session when interacting with a site. Use the same cookies and headers throughout, just like a real user would.
5. Smart retries
Hit a rate limit? Don't jump right back in. Use an exponential backoff. Here's a Python example:
import time
def make_request_with_backoff(url, max_retries=5):
for attempt in range(max_retries):
try:
# Your request code here
return response
except RateLimitException:
sleep_time = 2 ** attempt # Exponential backoff
time.sleep(sleep_time)
raise Exception("Max retries reached")
6. Watch and tweak
Keep an eye on your success rates. Seeing more blocks? Time to adjust. Tools like Web Scraping HQ offer auto-monitoring and tweaking to help you stay ahead of blocks.
These strategies can seriously cut your block risk during big scraping jobs. Remember, the goal is to be as invisible as possible while still getting your data.
"To avoid requests throttling, respect the website, and reduce your scraping speed." - Adelina Kiskyte, former Senior Content Manager at Oxylabs
This simple advice can go a long way in keeping your access to the data you need.
Login Problems
Password-protected content can be a real pain for web scrapers. But don't worry - we've got some tricks up our sleeve to get past those login screens.
Managing Logins and Cookies
The secret? Act like a real user. Here's how:
1. Use session objects
Python's requests.Session()
is your new best friend. It handles cookies for you, keeping you logged in. Check it out:
import requests
session = requests.Session()
login_url = "https://example.com/login"
data = {"username": "your_username", "password": "your_password"}
response = session.post(login_url, data=data)
# You're in! Now grab that protected content:
response = session.get("https://example.com/protected_page")
2. Handle CSRF tokens
Some sites use these to keep the bad guys out. You'll need to grab the token and include it in your login. Like this:
from bs4 import BeautifulSoup
login_page = session.get(login_url)
soup = BeautifulSoup(login_page.content, 'html.parser')
csrf_token = soup.find("input", {"name": "_token"})["value"]
data["_token"] = csrf_token
response = session.post(login_url, data=data)
3. Mix up your user agents
Websites can spot patterns. Keep 'em guessing by using different user agents.
4. Don't be a speed demon
Slow down, hotshot. Add some random delays between requests. time.sleep()
is your friend here.
5. Plan for failure
Sometimes logins fail. No biggie. Just try again:
import time
def login_with_retry(max_retries=3):
for attempt in range(max_retries):
try:
# Your login code here
return True
except Exception as e:
print(f"Login failed: {e}")
time.sleep(2 ** attempt) # Back off exponentially
return False
6. Break out the big guns for tough logins
Some sites use fancy JavaScript login forms. Time to call in Selenium or Puppeteer. They can click and type just like a real person.
Just remember: while you CAN scrape behind logins, it doesn't mean you SHOULD. Always check the site's terms of service and data rules. Get permission before you start grabbing data.
"When scraping a login page, always handle cookies and sessions securely. Use Python's requests.Session() to manage these automatically, ensuring your session stays active across requests." - Web Scraping Expert
Stick to these tips, and you'll be slipping past login screens like a pro in no time.
Key Tips and Tools
Web scraping can be tricky. But with the right approach and tools, you can overcome common hurdles. Let's look at some key strategies and resources to keep your scraping projects running smoothly.
Error Types and Solutions
Here's a quick guide to common errors and how to fix them:
Error Type | Common Causes | How to Fix |
---|---|---|
HTTP 403 Forbidden | IP blocking, User-Agent restrictions | Rotate IPs, use different User-Agents |
HTTP 429 Too Many Requests | Hitting rate limits | Add delays, rotate proxies |
Network Timeouts | Slow server, poor connection | Set longer timeouts, retry with backoff |
CAPTCHA Challenges | Anti-bot measures | Use CAPTCHA solvers, act more human-like |
Dynamic Content Issues | JavaScript-rendered pages | Use headless browsers (Puppeteer, Selenium) |
Data Extraction Errors | Website structure changes | Update scripts often, use robust parsing |
Login Problems | Authentication needed | Handle cookies and CSRF tokens |
Now, let's break down some key tips and tools:
1. Smart Proxy Management
Bright Data's Web Unlocker helps avoid IP blocks. It has a 99.9% success rate in bypassing anti-scraping measures. It rotates IPs to make your scraper look like normal traffic.
2. Act Like a Human
Web Scraping HQ says acting like a real user is key. Their tools add random delays between requests and copy normal browsing patterns. This helps avoid triggering anti-bot systems.
3. Handle Errors Well
Use try-except blocks in Python to deal with errors smoothly. Here's an example:
import requests
from requests.exceptions import RequestException
def scrape_with_retry(url, max_retries=3):
for attempt in range(max_retries):
try:
response = requests.get(url, timeout=10)
response.raise_for_status()
return response.text
except RequestException as e:
print(f"Attempt {attempt + 1} failed: {e}")
if attempt == max_retries - 1:
raise
return None
This function tries to scrape a URL multiple times, handling network errors and retrying when needed.
4. Use Headless Browsers
For sites with lots of JavaScript, tools like Puppeteer and Selenium are great. They can interact with web pages like a real browser, handling dynamic content and complex user actions.
5. Log and Monitor
Use Python's logging module or tools like Loguru to track what your scraper is doing. This helps find and fix issues quickly.
import logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
def scrape_page(url):
logger.info(f"Scraping {url}")
# Scraping logic here
logger.info(f"Completed scraping {url}")
6. Follow the Rules
Always check the robots.txt file of the website you're scraping. Following these rules helps avoid blocks and keeps your scraping ethical.
7. Use Scraping Frameworks
Consider frameworks like Scrapy. They have built-in features for handling common scraping issues. Scrapy offers good error handling, follows robots.txt rules, and works well with proxy services.
"The best approach is to prevent CAPTCHAs from appearing initially and, if blocked, to retry the request." - Web Scraping Expert
FAQs
What are the problems with the web scraping solution for integration?
Web scraping is powerful, but it's not without its challenges. Here are the main issues you'll face when integrating web scraping into your business:
1. Getting blocked
Websites are getting better at spotting and blocking scrapers. Cloudflare saw a 35% jump in bot detection across their network in 2022. But there's hope: Bright Data's rotating IP solutions have a 99.9% success rate in getting past these blocks.
2. Scaling up
Growing your scraping operation isn't easy. ScrapingBee found that their big clients often hit a wall when trying to go from 1,000 pages a day to 1 million without major changes to their setup.
3. Dealing with dynamic content
JavaScript is everywhere. The 2023 Web Almanac report says 98% of websites use it, which makes old-school scraping tough. You'll need tools like Puppeteer and Selenium to handle these tricky pages.
4. Keeping up with website changes
Websites change, and your scraper needs to keep up. Octoparse estimates that 30% of scraping projects need updates within just three months because of site tweaks.
5. Maintaining your setup
Running a scraping operation takes work. Zyte's survey found that companies spend 25-30% of their web scraping budget just keeping their infrastructure running smoothly.
A web scraping expert sums it up:
"Web scraping, a popular phrase being talked about, remains a mystery to many professionals. The key is to anticipate these challenges and build robust, adaptable systems that can evolve with the ever-changing web landscape."
In short: web scraping is powerful, but it's not a set-it-and-forget-it solution. You need to be ready to adapt and overcome these hurdles to make it work for your business.