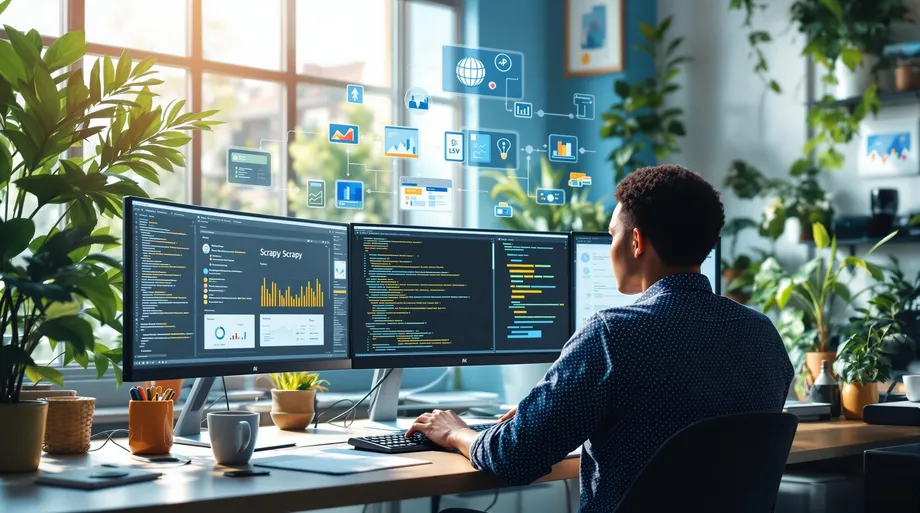
- Harsh Maur
- November 25, 2024
- 6 Mins read
- Scraping
Building Scalable Data Pipelines with Scrapy
Scrapy is a Python framework for building scalable data pipelines. It simplifies web scraping by automating tasks like data extraction, cleaning, and storage. Here's a quick breakdown of what you can do with Scrapy:
- Data Processing: Use
items.py
to define the data you need andpipelines.py
to process it. - Request Management: Middleware helps manage requests and responses, making your scraper act like a browser.
- Database Integration: Store large datasets efficiently with tools like PostgreSQL or MongoDB.
- Scaling: Use Scrapy-Redis to distribute scraping across multiple machines.
- Optimization: Adjust concurrency, enable caching, and use throttling to balance speed and server load.
- Error Handling: Implement error handling and logging to keep your pipeline running smoothly.
Whether you're handling small projects or large-scale operations, Scrapy provides the tools to build efficient, scalable pipelines.
Core Features of Scalable Data Pipelines in Scrapy
Using Item Pipelines for Data Processing
Scrapy's Item Pipelines help you process data smoothly and efficiently. Here's a simple example that handles product prices:
class PriceProcessingPipeline:
def process_item(self, item, spider):
# Convert price string to float
item['price'] = float(item['price'].replace('$', '').strip())
return item
This pipeline turns price text like "$29.99" into numbers that work well with databases. After your data goes through these pipelines, you'll need to handle how you talk to web servers.
Middleware for Managing Requests and Responses
Think of middleware as a traffic controller between Scrapy and websites - it manages how you send requests and handle what comes back.
"Scrapy's middleware architecture allows for sophisticated request handling and response processing, making it possible to handle complex scraping scenarios while maintaining scalability."
Middleware helps you:
- Make your scraper act like a real browser with proper headers and cookies
- Try again when requests fail
- Clean up response data before your spider sees it
After you've got your requests flowing smoothly, you'll want to store all that data properly.
Integrating Databases for Better Storage
When you're scraping lots of data, you need a good place to put it. Scrapy plays nice with many different databases, and you can hook them up through pipeline components.
Here's a smart way to handle big datasets:
class BulkInsertPipeline:
def __init__(self):
self.items = []
self.batch_size = 100
def process_item(self, item, spider):
self.items.append(item)
if len(self.items) >= self.batch_size:
self.bulk_insert()
return item
This pipeline bundles multiple records together before saving them to your database. It's like packing more groceries into each trip - you save time and energy compared to making separate trips for each item. This method works great with PostgreSQL or MongoDB and can handle millions of records without breaking a sweat.
The magic happens when you combine all these pieces: Item Pipelines process your data, Middleware manages your web requests, and smart database integration keeps everything stored neatly. Together, they create a system that can grow with your needs while keeping things quick and reliable.
Related video from YouTube
Ways to Improve Data Pipelines in Scrapy
Let's dive into how you can make your Scrapy pipelines work better and handle more data.
Tuning Concurrency and Delay Settings
Getting the right balance between speed and server load is key. Think of it like adjusting the water flow in a pipe - too much pressure can cause problems, too little slows everything down.
Here's how to control your spider's crawling speed in settings.py
:
# settings.py
CONCURRENT_REQUESTS = 16
DOWNLOAD_DELAY = 2 # seconds between requests
Using Caching and Throttling
Want to make your scraper smarter? Add caching and throttling. Caching works like a memory bank - it stores responses so you don't need to keep asking for the same data. Meanwhile, throttling acts like a speed governor, adjusting how fast your scraper runs based on how the server responds.
Here's the setup for caching:
# settings.py
HTTPCACHE_ENABLED = True
HTTPCACHE_EXPIRATION_SECS = 86400 # Cache for 24 hours
HTTPCACHE_DIR = 'httpcache'
And here's how to enable smart throttling:
# settings.py
AUTOTHROTTLE_ENABLED = True
AUTOTHROTTLE_START_DELAY = 5
AUTOTHROTTLE_TARGET_CONCURRENCY = 1.0
Scaling with Scrapy-Redis for Distributed Scraping
When you need to step up your game and scrape across multiple machines, Scrapy-Redis is your friend. It teams up with Redis (a speedy in-memory database) to coordinate your scraping army.
Set it up like this:
# settings.py for Scrapy-Redis
SCHEDULER = "scrapy_redis.scheduler.Scheduler"
DUPEFILTER_CLASS = "scrapy_redis.dupefilter.RFPDupeFilter"
REDIS_URL = 'redis://localhost:6379'
Redis works as your command center, making sure all your spiders work together without doing the same job twice. This setup lets you scale up your scraping operation when a single machine just won't cut it anymore.
sbb-itb-65bdb53
Best Practices for Scalable Scrapy Pipelines
Implementing Error Handling
Your Scrapy pipeline needs solid error handling to keep running smoothly when things go wrong. Here's how to set up error handling with errback
:
def start_requests(self):
urls = ['http://example.com']
for url in urls:
yield scrapy.Request(url,
callback=self.parse,
errback=self.handle_error,
dont_filter=True)
def handle_error(self, failure):
self.logger.error(f"Request failed: {failure.request.url}")
# Implement retry logic or alternative processing
Using Logging and Debugging Tools
Keep an eye on what's happening in your pipeline with Scrapy's built-in logging system. It's like having a dashboard for your scraper:
LOG_LEVEL = 'DEBUG'
LOG_FORMAT = '%(asctime)s [%(name)s] %(levelname)s: %(message)s'
When you hit a tricky problem, Scrapy Shell is your best friend:
scrapy shell 'http://example.com'
Tracking Performance and Monitoring
Want to know how well your scraper is doing? Scrapy's stats collector shows you the numbers that matter:
# settings.py
STATS_CLASS = 'scrapy.statscollectors.MemoryStatsCollector'
STATS_DUMP = True
# In your spider
def closed(self, reason):
stats = self.crawler.stats.get_stats()
self.logger.info(f"Items scraped: {stats.get('item_scraped_count', 0)}")
self.logger.info(f"Request bytes: {stats.get('downloader/request_bytes', 0)}")
For deep performance analysis, especially in busy pipelines, cProfile helps you spot bottlenecks:
# middleware.py
from cProfile import Profile
class ProfilerMiddleware:
def __init__(self):
self.profiler = Profile()
def process_request(self, request, spider):
self.profiler.enable()
return None
def process_response(self, request, response, spider):
self.profiler.disable()
self.profiler.dump_stats('scrapy_stats.prof')
return response
If you're running large-scale scraping operations and need professional-grade error handling and monitoring, check out Web Scraping HQ's managed solutions.
Conclusion and Further Learning
Summary of Key Techniques
Want to build data pipelines with Scrapy? You'll need to master its core parts. Scrapy gives you the tools to pull and process data in an organized way, plus solid error handling and monitoring features.
To make Scrapy run like a well-oiled machine, focus on three main areas:
- Smart data processing that doesn't waste resources
- Control your scraping speed with caching and throttling
- Scale up using tools like Scrapy-Redis when you need more power
Keep an eye on your scraper's health through logs and debugging tools. Track how well it performs to catch issues early.
Remember: while Scrapy works great for many projects, bigger business operations might need extra tools in their toolkit.
Using Services Like Web Scraping HQ
Sometimes Scrapy alone isn't enough for big business needs. That's where Web Scraping HQ steps in. They fill the gaps with features that make large-scale scraping easier:
- Legal compliance checks
- Auto quality control
- Custom data extraction that works at scale
Web Scraping HQ works with all kinds of data projects. Whether you're researching companies, tracking products, or studying job markets, they add pro-level support and special features that make Scrapy even better at handling big jobs.
FAQs
How to optimize scrapy?
Let's talk about getting the most out of Scrapy when handling big data loads. It's all about finding that sweet spot between speed and system resources.
"Scrapy is designed to handle large volumes of data, but it requires careful tuning to achieve optimal performance."
Here's how to fine-tune Scrapy for better performance:
Getting the CPU-Memory Mix Right Start with lower concurrency settings and work your way up until you hit 80-90% CPU usage - that's your performance sweet spot. Keep an eye on your memory usage though. If it starts climbing too high, dial back those global concurrency limits.
Speed Things Up Want to make Scrapy run smoother? Here's what works:
def process_item(self, item, spider):
try:
# Process item
return item
except Exception as e:
logger.error(f"Processing failed: {e}")
Turn on HTTP caching in settings.py:
- Set HTTPCACHE_ENABLED = True to cut down on server requests
- Enable AutoThrottle with AUTOTHROTTLE_ENABLED = True
- Set AUTOTHROTTLE_TARGET_CONCURRENCY = 1.0 to let Scrapy manage request speeds
Finding Your Speed Limit Begin with 16 concurrent requests. Is your CPU only at 60%? Bump up the number bit by bit until you find that perfect balance - you want maximum speed without overloading your system.
Going Big? For larger projects, try Scrapy-Redis. Just pip install scrapy-redis and switch from Spider to RedisSpider class. This lets you spread your scraping across multiple machines while keeping everything running smoothly.