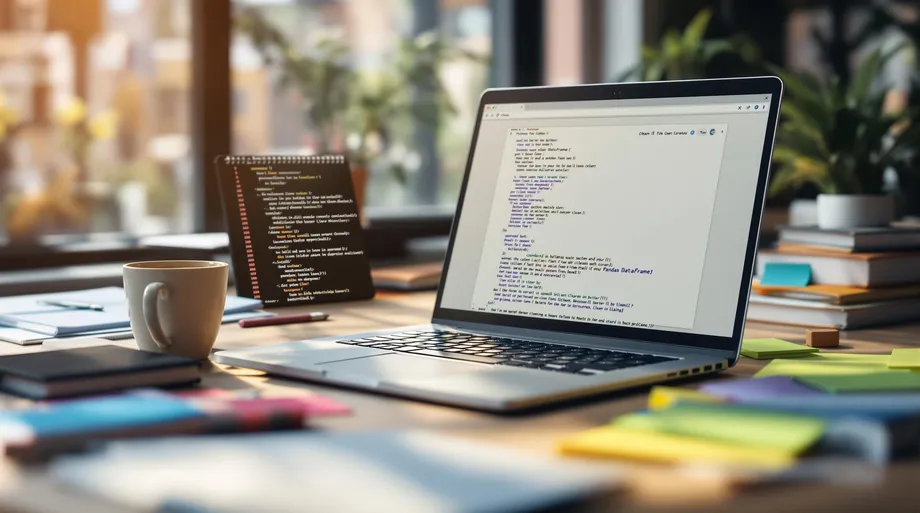
- Harsh Maur
- December 30, 2024
- 6 Mins read
- Scraping
Cleaning Web-Scraped Data with Python and Pandas
Want to make messy web-scraped data useful? Python and Pandas can help you clean and structure it for analysis. Here's how:
- Common Problems with Web-Scraped Data: Inconsistent formats, missing values, duplicates, and mixed data types.
- Key Cleaning Techniques: Use Pandas functions like
fillna()
,drop_duplicates()
, andto_datetime()
to fix issues efficiently. - Setup Essentials: Install libraries like Pandas, BeautifulSoup, and NumPy in a virtual environment for a clean workspace.
- Advanced Tools: Merge datasets, split fields, and normalize data for deeper analysis.
With these steps, you’ll transform raw data into reliable insights, ready for decision-making. Let’s dive into the details.
Data Cleaning in Pandas
Setting Up the Python Environment
Getting your Python environment ready is the first step to turning raw web-scraped data into structured insights. A properly set up environment ensures smooth data handling and minimizes technical hiccups.
Installing Libraries
Every data cleaning project begins with the right tools. Below are the key libraries you'll need:
Library | Purpose | Installation Command |
---|---|---|
Pandas | For data manipulation and analysis | pip install pandas |
NumPy | Handles large arrays and matrices | pip install numpy |
BeautifulSoup | Extracts data from HTML or XML documents | pip install beautifulsoup4 |
It's a good idea to use a virtual environment to keep your project dependencies separate and avoid version conflicts. You can set one up with:
python -m venv scraping_env
Activate it using:
- Unix/Linux:
source scraping_env/bin/activate
- Windows:
scraping_env\Scripts\activate
"Using a virtual environment like
venv
can help isolate your project dependencies and avoid version conflicts" [1].
Importing Libraries
After installation, import the libraries at the start of your script. A typical setup might look like this:
import pandas as pd
import numpy as np
from bs4 import BeautifulSoup
To ensure everything is installed correctly, run pip list
to check the installed packages and confirm compatibility with your Python version.
Managing Library Versions
Keep track of your library versions by saving them to a file with:
pip freeze > requirements.txt
This makes it easier to replicate the environment later.
Now that your Python environment is configured, you're ready to dive into your web-scraped data.
Loading and Exploring Web-Scraped Data
Once your Python environment is ready, the next step is getting your web-scraped data into a format that’s easy to analyze.
Loading Data into a DataFrame
Pandas makes it easy to load web-scraped data into a DataFrame. Since scraped data often comes in formats like JSON or HTML, functions such as read_html()
and read_json()
can be particularly handy.
# Loading CSV data
df = pd.read_csv('scraped_data.csv')
# Loading HTML tables directly from a webpage
tables = pd.read_html('https://example.com/table')
df = tables[0] # Use the first table if there are multiple
Here’s a quick guide to some common Pandas functions for loading data:
Data Format | Pandas Function |
---|---|
CSV | read_csv() |
HTML | read_html() |
JSON | read_json() |
Excel | read_excel() |
Inspecting Data
Once your data is loaded, it’s time to check its structure and quality. Functions like df.head()
, df.info()
, and df.describe()
are great for getting a quick overview.
Key areas to focus on:
- Data Types: Use
df.dtypes
to confirm that columns have the correct data types. - Missing Values: Identify null values with
df.isnull().sum()
. - Unique Values: Check distinct entries in a column using
df['column_name'].unique()
.
If your dataset includes dates, you can format them properly with pd.to_datetime()
.
For large datasets, df.describe(include='all')
provides a detailed summary, helping you spot potential issues quickly.
If your project involves handling large or messy datasets, consider using services like Web Scraping HQ. They offer pre-cleaned datasets, saving you time on initial preparation and ensuring high-quality data.
Now that your data is loaded and inspected, you’re ready to tackle common cleanup tasks like handling missing values, duplicates, or inconsistent formats.
sbb-itb-65bdb53
Data Cleaning Techniques with Pandas
After inspecting your web-scraped data, it's time to transform it into a clean, analysis-ready format. Pandas provides a range of tools to make this process efficient and straightforward.
Handling Missing Data
Pandas makes it easy to spot and handle missing values:
# Check for missing values
missing_values = df.isnull().sum()
# Remove rows with missing values
df_clean = df.dropna()
# Fill missing values with specific strategies
df['price'].fillna(df['price'].mean(), inplace=True)
df['category'].fillna('Unknown', inplace=True)
You can replace missing numerical values with the mean or median, fill missing categories with placeholders like 'Unknown,' or use forward/backward filling for time series data. Once you've dealt with missing entries, the next step is to check for duplicates.
Removing Duplicates
Duplicate data can skew your analysis, but Pandas makes it simple to identify and remove them:
# Check for duplicate rows
duplicate_count = df.duplicated().sum()
# Remove duplicates, keeping the first occurrence
df_unique = df.drop_duplicates(subset=['url', 'title'], keep='first')
When working with scraped data, decide which columns define uniqueness to avoid deleting valid variations. After removing duplicates, you’ll want to focus on making your dataset consistent.
Standardizing Data
Standardizing ensures your data is uniform and ready for analysis:
# Standardize data formats
df['date'] = pd.to_datetime(df['date']) # Convert to datetime
df['category'] = df['category'].str.lower() # Lowercase text
df['description'] = df['description'].str.strip() # Remove extra spaces
For tasks like scaling numerical data, you can use tools like MinMaxScaler:
# Normalize numerical columns
from sklearn.preprocessing import MinMaxScaler
scaler = MinMaxScaler()
df['price_normalized'] = scaler.fit_transform(df[['price']])
These steps ensure your data is clean, consistent, and ready for deeper analysis.
Advanced Data Manipulation: Merging and Splitting
When dealing with web-scraped data, you often need to combine datasets or break down complex fields into simpler components. Here's how Pandas can help streamline these tasks.
Merging Datasets
Pandas offers powerful tools to combine data efficiently:
# Merge datasets or concatenate vertically
merged_data = pd.merge(scraped_profiles, purchase_history, on='customer_id', how='outer')
combined_data = pd.concat([dataset1, dataset2], ignore_index=True)
# Group and summarize data
summary = customer_data.groupby('country').agg({
'purchase_amount': 'mean',
'customer_id': 'count'
}).reset_index()
You can use the indicator=True
option during a merge to flag unmatched records. This is handy for spotting data quality issues.
Splitting and Reformatting
Web-scraped data often contains fields that need to be split or reformatted for better usability:
# Split full names into first and last names
df[['first_name', 'last_name']] = df['full_name'].str.split(' ', expand=True)
# Extract numeric values from text
df['price_clean'] = df['price_text'].str.extract(r'(\d+\.?\d*)').astype(float)
# Convert date strings to datetime objects
df['date_posted'] = pd.to_datetime(df['date_string'], format='%Y-%m-%d')
# Standardize and clean address fields
df['address_clean'] = df['address'].str.replace('[^\w\s]', '').str.title()
"Data cleaning is the process of identifying and correcting errors in a dataset." - W3Schools, Pandas - Cleaning Data [2]
Data cleaning is a critical step, often taking up to 80% of your analysis time [3]. To handle large datasets more effectively:
- Use vectorized operations instead of loops for faster processing
- Drop unnecessary columns before merging to save memory
These methods can transform messy, unstructured web-scraped data into a clean, usable format, setting the stage for deeper analysis or seamless integration into your workflows.
Conclusion and Next Steps
Key Points Summary
Cleaning your data is a crucial step in any web scraping project. This guide covered essential Pandas techniques to help you turn raw data into reliable, analysis-ready datasets. The success of your data cleaning efforts comes down to knowing your dataset and using the right tools effectively.
Here are some key practices to keep in mind:
- Use vectorized operations and standardize data early to streamline workflows.
- Validate your cleaned data by profiling it (like spotting outliers) and leveraging visualizations.
- Keep a record of your cleaning steps to ensure reproducibility.
- Perform regular quality checks to maintain consistency throughout the process.
Additional Resources
If you’re looking to expand your knowledge and sharpen your skills, these resources are great starting points:
Resource Type | Description | Best For |
---|---|---|
DataHeadhunters Academy | Detailed Python data cleaning guides | Tutorials and hands-on learning |
Web Scraping HQ | Managed scraping services and custom data solutions | Large-scale data extraction |
Pandas Documentation | Official guide for data manipulation functions | In-depth technical reference |
For large-scale projects, Web Scraping HQ offers automated solutions that handle both data extraction and cleaning. Their services support a variety of data types, like company profiles and real estate listings, with built-in quality assurance to ensure accuracy.
Start with the basics, and as you gain confidence, incorporate advanced techniques. Working with real datasets will help you develop the intuition needed to tackle data quality challenges. With these skills, you’ll be ready to extract meaningful insights from even the messiest raw data. </