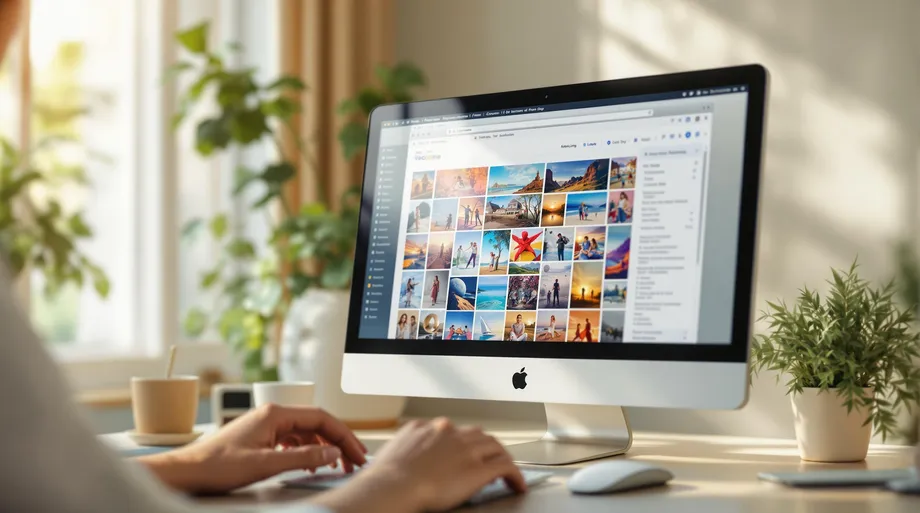
- Harsh Maur
- February 28, 2025
- 12 Mins read
- WebScraping
Everything You Need to Know About Scraping Images from a Website
Want to scrape images from a website but don’t know where to start? Here's a quick guide:
- What is Image Scraping?: It’s the process of automatically extracting images from websites using tools like Python, Beautiful Soup, or Selenium.
- Where is it Used?: E-commerce (product tracking), real estate (property images), and digital asset management (organizing large image libraries).
- Legal Considerations: Always follow copyright laws, terms of service, and data privacy regulations.
- Tools You Need: Python libraries (e.g., Beautiful Soup, Selenium, Pillow), browser automation tools, and proxies for large-scale scraping.
- Challenges: Dynamic content, lazy-loading images, and anti-scraping protections like rate limiting and IP blocking.
Quick Comparison of Tools
Tool | Purpose | Best For | Complexity |
---|---|---|---|
Beautiful Soup | HTML parsing and DOM navigation | Simple websites | Low |
Selenium | Browser automation | JavaScript-heavy content | Medium |
Scrapy | Large-scale scraping | Managing multiple pages | High |
Playwright | Cross-browser automation | Advanced dynamic content | High |
Key Takeaway: Scraping images is a powerful technique, but it requires the right tools, ethical practices, and a structured approach to succeed.
Image Scraping Basics
Reading HTML Image Elements
HTML image elements are a primary source for static files, accessible via unique URLs in img
tags. Key attributes include:
-
src
: Points to the direct image URL. -
alt
: Contains descriptive text for the image. -
srcset
: Lists multiple resolutions for responsive design.
These attributes are crucial for identifying and extracting images during web scraping.
"So, when web scraping for images, we'll mostly be looking for
img
tags and theirsrc
orsrcset
attributes." - Mazen Ramadan, Scrapfly.io
For example, on eBay product listings, images are embedded within div
elements with the class s-item__image-wrapper image-treatment
. The actual image URLs are stored in the src
attributes of img
tags.
Image File Types and Links
Understanding image formats helps you choose the right type for your scraping needs. Here's a quick breakdown:
Format | Best Use Case | Compression | Key Features |
---|---|---|---|
JPG/JPEG | Photographs | Lossy | Smaller sizes, faster loading |
PNG | Graphics | Lossless | Supports transparency |
SVG | Vector graphics | Vector-based | Scales infinitely |
GIF | Animations | Lossless | Limited color range |
For instance, JPGs work well for product photos due to their compact size, while SVGs are ideal for logos since they maintain quality at any scale.
Python Tools for Scraping Images from a Website
- Requests: Great for handling HTTP requests but lacks JavaScript support.
- Beautiful Soup: Simplifies HTML parsing and DOM navigation.
- Scrapy: Perfect for managing large-scale crawling projects.
Handling Dynamic Content
- Selenium: Offers full browser automation for JavaScript-heavy sites.
- Playwright: Provides robust cross-browser support and handles JavaScript seamlessly.
Tools like gImageGrabber (for Google Images) and PicoScrape (for Unsplash) can save time for specific tasks. The choice of tool depends on the complexity of the website and the scope of your project.
Requests and Beautiful Soup may not suffice for modern, JavaScript-reliant websites. In these cases, browser automation tools like Selenium or Playwright are essential, even though they require more resources and a steeper learning curve.
Next, we’ll walk through a step-by-step guide on deploying these tools effectively.
How to Scrape Images: Step by Step
Python Setup Guide
To get started, you'll need to set up your Python environment with the following libraries. Install them by running:
pip install beautifulsoup4 selenium pandas pyarrow Pillow requests
Here’s what each library does:
- Beautiful Soup: Parses HTML content.
- Selenium: Manages interactions with dynamic websites.
- Pillow: Handles image processing.
- Requests: Sends HTTP requests.
Once these are installed, you’ll have the essential tools to build your image scraper.
Creating a Simple Scraper
Here’s how to combine these tools to create a basic image scraper:
import requests
from selenium import webdriver
from selenium.webdriver.common.by import By
import urllib
import time
driver = webdriver.Chrome('chromedriver', chrome_options=chrome_options)
driver.get(target_url)
This script sets up a web driver and begins extracting images. Selenium is especially useful for handling JavaScript-rendered content, which simpler tools might miss.
Once you’ve retrieved the images, you’ll need a structured way to store them.
Image Storage Methods
To keep your scraped images organized, consider this storage structure:
Storage Level | Purpose | Example Structure |
---|---|---|
Root Directory | Main project folder | /scraped_images/ |
Category Folders | Organize by content type | /scraped_images/products/ |
Date-based Subfolders | Organize by date | /scraped_images/products/2025-02/ |
Resolution Folders | Manage image quality | /scraped_images/products/2025-02/high_res/ |
For better efficiency, you can also use metadata tagging, cloud storage, and asset classification. Consider creating a database to link images with their source URLs, scrape dates, resolutions, and formats. Cloud storage allows for scalability and easy team collaboration, while metadata tagging ensures quick retrieval and categorization.
Scraping Complex Image Types
Modern websites often use advanced techniques to load images, making it necessary to apply specific methods for effective scraping.
JavaScript Image Extraction
Images rendered by JavaScript don’t appear in the initial HTML source, which can complicate the scraping process. To handle this, Selenium’s dynamic capabilities come in handy. Use explicit waits to extract these images:
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
wait = WebDriverWait(driver, 10)
images = wait.until(EC.presence_of_all_elements_located((By.CSS_SELECTOR, "img.dynamic-class")))
image_urls = [img.get_attribute('src') for img in images]
For lazy-loading images, you’ll need to simulate scrolling to trigger the loading process.
Lazy-Loading Image Solutions
Lazy-loading images only load when they come into view. To scrape these, simulate scrolling to ensure all images are loaded:
def scroll_and_extract(driver):
last_height = driver.execute_script("return document.body.scrollHeight")
while True:
# Scroll down
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
# Wait for new images to load
time.sleep(2)
# Calculate new scroll height
new_height = driver.execute_script("return document.body.scrollHeight")
# Break if no more content loads
if new_height == last_height:
break
last_height = new_height
This method is also useful for handling infinite scroll pages, which require continuous loading of new content.
Infinite Scroll and CDN Images
Expanding on lazy-loading techniques, infinite scroll pages and images hosted on CDNs (Content Delivery Networks) add another layer of complexity. These challenges often require a mix of strategies:
Scenario | Challenge | Method | Solution |
---|---|---|---|
Infinite Scroll | Dynamic content loading | XHR request analysis | Monitor network requests to find content API endpoints |
CDN Images | Request validation | Proxy rotation | Use residential proxies with varied User-Agent headers |
Rate Limiting | Server blocks | Delay implementation | Add random delays (1–3 seconds) between requests to avoid detection |
For CDN-hosted images, headers play a key role in bypassing restrictions. Use proper header configurations as shown below:
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36',
'Referer': 'https://example.com',
'Accept': 'image/webp,image/apng,image/*,*/*;q=0.8'
}
response = requests.get(image_url, headers=headers)
"Lazy loading is a design pattern commonly used in computer programming and mostly in web design and development to defer initialization of an object until the point at which it is needed. It can contribute to efficiency in the program's operation if properly and appropriately used." - Wikipedia
For high-volume tasks, such as scraping images protected by Cloudflare, Scrapfly has demonstrated success. On August 22, 2024, their API with the asp=True
option effectively bypassed "403 Forbidden" errors, making it a reliable choice for large-scale image scraping.
sbb-itb-65bdb53
Guidelines of Scraping Images from a Website
Before starting any scraping project, always review the website's robots.txt file. This file specifies which areas of the site are open for automated access. Additionally, check the Terms of Service (ToS) to understand the rules about data usage and access. Following these guidelines helps you avoid potential legal troubles and prevents your access from being blocked.
Here are some key areas to keep in mind:
Requirement | Implementation | Impact |
---|---|---|
Terms of Service | Review the website's ToS for scraping rules | Helps avoid legal complications and service restrictions |
HTTP Headers | Check for directives like X-Robots-Tag | Ensures compliance with site-specific rules |
Copyright Rules | Confirm image usage rights and restrictions | Avoids intellectual property violations |
Server Load | Monitor response times and adjust request rates | Prevents overwhelming the website's servers |
Managing your request speed is another critical step to ensure compliance with these rules.
Speed Limits and Proxy Use
Controlling the speed of your requests is essential for maintaining access. A good practice is to send one request every 10 to 15 seconds, which reduces the risk of IP blocks.
For larger-scale scraping projects, consider these techniques:
- Rate Limiting: Use strategies like exponential backoff to adjust the frequency of requests.
- User-Agent Rotation: Periodically change the User-Agent string to mimic different browsers.
- Proxy Rotation: Distribute traffic across multiple IP addresses to avoid detection and maintain access.
Proxy rotation, in particular, helps simulate legitimate user behavior while staying within a site's allowed limits.
async def fetch_with_backoff(url, max_retries=3):
for attempt in range(max_retries):
try:
await asyncio.sleep(10 + (2 ** attempt))
async with session.get(url) as response:
return await response.read()
except Exception as e:
if attempt == max_retries - 1:
raise e
Once you've set up these systems, it's equally important to handle errors effectively.
Error Management
Even with careful speed management, errors can occur. Use the following strategies to handle them:
- Response Validation: Check HTTP status codes and respond accordingly.
- Connection Issues: Implement retry logic with exponential backoff to manage temporary failures.
- Data Integrity: Ensure that downloaded images are complete and properly formatted.
Here’s how to address specific error types:
Error Type | Initial Response | Escalation Strategy |
---|---|---|
429 (Too Many Requests) | Pause for 30 seconds | Switch to a backup proxy pool |
403 (Forbidden) | Rotate the User-Agent | Change browser fingerprints |
500x (Server Errors) | Wait 60 seconds | Reduce the number of simultaneous connections |
Fixing Common Scraping Problems
This section dives into some of the technical challenges often faced during image scraping and how to address them. It focuses on three key areas: bypassing anti-scraping measures, preserving image quality, and cleaning up image data.
Anti-Scraping Protection
Websites today use advanced tools to block automated scraping. Getting past these defenses requires mimicking human behavior while respecting the site's usage policies. Here's a quick breakdown of common anti-scraping methods and how to handle them:
Protection Type | Solution | Implementation |
---|---|---|
IP Blocking | Rotating proxies | Spread requests across multiple IP addresses |
Rate Limiting | Throttle requests | Slow down the frequency of requests |
JavaScript Challenges | Headless browsers | Use tools like Selenium for rendering |
User-Agent Detection | Rotate fingerprints | Switch between common browser user agents |
"Anti-scraping refers to all techniques, tools, and approaches to protect online data against scraping. In other words, anti-scraping makes it more difficult to automatically extract data from a web page. Specifically, it's about identifying and blocking requests from bots or malicious users." - ZenRows
Once you've navigated these barriers, the next step is ensuring the images you scrape meet quality standards.
Image Quality Issues
To maintain high-quality scraped images, you’ll need to focus on three main aspects:
- Format Validation: Check that the images meet required formats and dimensions before downloading.
- Resolution Management: Set up your scraper to handle images of varying resolutions effectively.
- Quality Preservation: Enforce minimum resolution and format standards to ensure only usable images are saved.
Image Data Cleanup
A clean, well-organized dataset is essential for any image scraping project. This involves validating data and removing duplicates. Here are some effective methods for detecting duplicates:
Method | Best For | Performance Impact |
---|---|---|
Hash-based Comparison | Large datasets | Fast and uses minimal memory |
Pixel Comparison | Small datasets | Highly accurate but resource-heavy |
Metadata Analysis | Initial filtering | Quick but less precise |
To keep your dataset tidy, follow these strategies:
- Assign unique IDs to each image for easy tracking.
- Use lossy hash algorithms to quickly spot duplicates.
- Schedule regular automated cleanup scans.
- Adjust similarity thresholds based on the type of images you're working with.
Regular monitoring of storage and cleanup processes ensures your dataset remains efficient and manageable. These steps not only improve your data organization but also build on earlier error management practices.
Working with Scraped Images
Once your image dataset is ready, the next step is to refine it through editing, data analysis, and AI-driven processing.
Image Editing Tools
For image editing, the Python Imaging Library (Pillow) is a reliable choice for basic to intermediate tasks. Here’s how it compares to other tools for common image manipulation needs:
Tool | Best Use Case | Processing Speed | Learning Curve |
---|---|---|---|
Pillow | Basic editing & batch processing | Moderate | Low |
OpenCV | Advanced computer vision tasks | Fast | High |
scikit-image | Scientific image analysis | Moderate | Medium |
Pillow’s ImageEnhance module makes it easy to adjust brightness, contrast, and sharpness with minimal code. For effects like blurring or edge detection, the ImageFilter module comes in handy. When combined with NumPy, Pillow can also handle batch processing and complex pixel-level operations efficiently.
After editing, the next step is analyzing the enhanced images to extract meaningful data and improve organization.
Image Data Analysis
Once your images meet quality standards, analyzing the data within them can reveal critical metadata. With around 60% of corporate data now stored in the cloud, effective image analysis ensures your dataset is well-organized and standardized. Focus on these key areas:
- EXIF Data: Extract metadata such as camera settings, timestamps, and locations.
- Format Analysis: Ensure image formats are consistent and optimized for your needs.
- Quality Metrics: Evaluate resolution, color depth, and compression levels to maintain high standards.
AI Image Processing
AI tools take image processing to the next level, offering advanced capabilities for restoration and analysis. Here’s what they bring to the table:
1. Object Detection and Classification
Machine learning models can identify and categorize objects within images with impressive accuracy. This is particularly helpful for organizing large datasets based on content.
2. Image Restoration
AI-powered tools can significantly improve image quality by:
- Removing noise and artifacts
- Upscaling resolution intelligently
- Correcting color imbalances
- Repairing damaged areas
3. Content Analysis
Deep learning models excel in tasks like:
- Semantic segmentation
- Feature extraction
- Pattern recognition
- Categorizing content effectively
By combining Pillow for initial edits with AI tools for deeper analysis and categorization, you can achieve efficient workflows and high-quality results.
Conclusion
The data analytics market, valued at $74.99 billion, is growing fast, with a 25.7% CAGR projected through 2028. This makes mastering image scraping a key skill for businesses aiming to stay competitive.
Key Takeaways
Image scraping success relies on three core elements: technical know-how, ethical practices, and strategic execution. These factors not only improve competitiveness by 74% but also reduce research time by 60%. Here's a quick breakdown:
Aspect | Key Consideration | Impact |
---|---|---|
Technical Setup | Python tools like BeautifulSoup, Selenium, Pillow | Enables smooth extraction and processing |
Legal Compliance | Adherence to Robots.txt, ToS, GDPR | Minimizes risk and ensures compliance |
Performance | Using rate limits (e.g., 1 request every 10-15 seconds) | Reduces server strain and avoids bans |
These elements serve as the foundation for responsible and effective image scraping.
Web scraping now accounts for 25% of all Internet traffic, underscoring the importance of ethical practices. As Zeid Abughazaleh from Proxidize puts it:
"Image scraping automates manual image collection into a quick and streamlined process."
By combining technical expertise with ethical considerations, you can optimize your approach to image scraping. Focus on:
- Request Management: Schedule scraping during off-peak hours and add consistent delays.
- Data Quality: Implement strict validation to ensure accuracy.
- Ethical Practices: Be transparent about how data is collected and used.
As Forage AI aptly states:
"Ethical web data extraction is a powerful technology that comes with important responsibilities."
Balancing technical skills with ethical guidelines is the key to successful image scraping. By following best practices and using the right tools, businesses can streamline their efforts while respecting digital boundaries and legal frameworks.