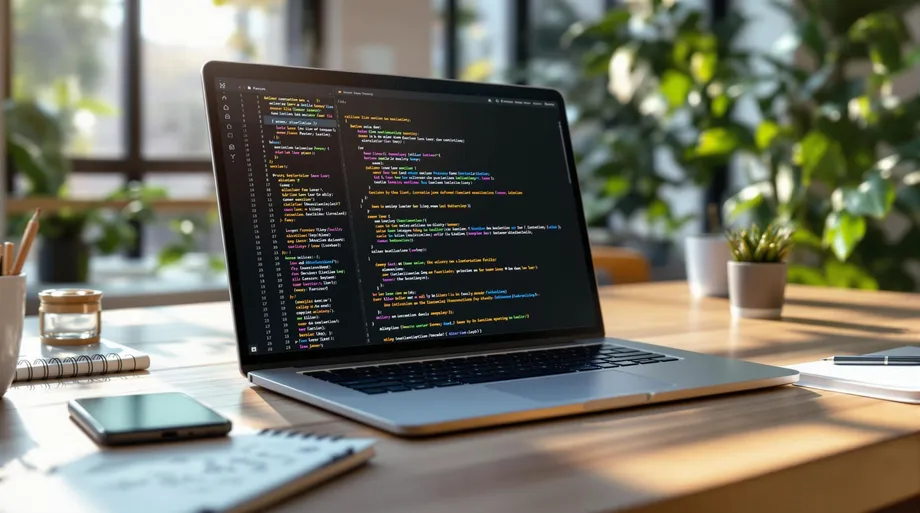
- Harsh Maur
- November 22, 2024
- 9 Mins read
- Scraping
How to bypass Cloudflare for web scraping
Bypassing Cloudflare's advanced anti-bot protections can be challenging but is possible with the right tools and ethical practices. Here's a quick summary of what you need to know:
- Why It's Hard: Cloudflare uses methods like IP blocking, browser fingerprinting, and CAPTCHA challenges to detect bots.
-
Ethical Rules: Always follow data protection laws, respect a site
robots.txt
, and avoid overloading servers with too many requests. - Common Issues: Expect errors like 403 (Access Denied) or 1020 (Rate Limiting) if your bot doesn't mimic real user behavior.
-
Tools to Use:
- Proxies: Rotate residential proxies to avoid IP bans.
- Browser Automation: Tools like Puppeteer or Playwright can simulate human browsing.
- CAPTCHA Solvers: Use tools like FlareSolverr to handle CAPTCHA challenges.
-
Advanced Tactics:
- Mimic human behavior with randomized mouse movements and delays.
- Rotate HTTP headers and user-agent strings to appear more authentic.
- Render JavaScript properly using tools like Puppeteer or FlareSolverr.
Quick Comparison of Tools
Tool/Service | Features | Best For | Limitations | Cost |
---|---|---|---|---|
FlareSolverr | Handles JavaScript & CAPTCHA challenges | Small projects | Limited scalability | Free/Open-source |
Puppeteer | Browser automation with stealth plugins | JavaScript developers | High resource usage | Free/Open-source |
Cloudscraper | Solves basic challenges in Python | Simple scraping tasks | Less reliable for complex | Free/Open-source |
Web Scraping HQ | Managed service with compliance | Enterprise solutions | Expensive | Starts at $449/mo |
Related video from YouTube
How Cloudflare Blocks Bots
To effectively scrape websites protected by Cloudflare, it's key to understand how their bot detection works. By learning the details of these systems, scrapers can craft approaches that better mimic real user behavior.
Cloudflare's Security Features
Cloudflare uses a layered security system with advanced methods to spot and block bots. One of its main tactics is TLS fingerprinting, which examines the technical details of incoming connections. This helps Cloudflare identify the true client-making requests, even when bots attempt to disguise themselves as regular browsers.
Here are some of the platform’s key defenses:
- IP-based Detection: Tracks traffic patterns and flags suspicious activity from specific IPs.
- Browser Fingerprinting and Behavioral Analysis: Looks at browser details, JavaScript execution, and user actions to tell humans apart from bots.
- TLS Fingerprinting: Examines connection details to verify if the client is genuine.
Common Errors When Scraping
These advanced protections often result in specific error codes when bots are detected. Below are the most common errors scrapers face:
Error Code | Description | Common Trigger |
---|---|---|
403 | Access Denied | Using non-browser user agents or mismatched HTTP headers |
1020 | Rate Limiting | Sending too many requests from the same IP |
1015 | Too Many Requests | Exceeding request limits set by the website |
Cloudflare’s detection system often flags automation through irregular HTTP headers. For example, if headers don’t align with standard browser behavior or if the TLS fingerprint doesn’t match the declared user agent, the traffic is marked as suspicious.
To navigate these defenses, tools like FlareSolverr can be used. They mimic browser activity to handle JavaScript challenges and bypass other Cloudflare protections.
Ways for how to Bypass Cloudflare
Before diving into technical methods, it's crucial to stick to ethical scraping practices. This means:
- Following robots.txt directives
- Adding reasonable delays between requests
- Using proper user-agent strings that reflect legitimate usage
For more details on ethical considerations, check out the "Ethical and Legal Rules to Follow" section mentioned earlier.
Using Proxies to Avoid IP Blocks
Rotating residential proxies is a key tool for getting around Cloudflare's defenses. Unlike data center proxies, residential IPs are tied to real internet service providers, making your traffic look more like that of a regular user. Here’s a Python example of how to use a proxy rotation system:
import requests
from proxy_rotation import ProxyRotator
proxy_pool = ProxyRotator(residential_ips=True)
session = requests.Session()
def make_request(url):
proxy = proxy_pool.get_next_proxy()
session.proxies = {
'http': f'http://{proxy}',
'https': f'https://{proxy}'
}
return session.get(url)
While proxies are vital, combining them with browser automation tools can make your approach even more effective.
Using Browser Automation Tools
Browser automation tools simulate human browsing patterns, helping you navigate Cloudflare's protections. One reliable option is Playwright, which can handle these challenges effectively. Here’s a basic setup:
const { chromium } = require('playwright');
async function scrapePage(url) {
const browser = await chromium.launch();
const context = await browser.newContext({
userAgent: 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) Chrome/118.0.0.0'
});
const page = await context.newPage();
await page.goto(url);
}
This approach helps mimic real user behavior, reducing the chances of being flagged.
Solving CAPTCHA Challenges
CAPTCHA challenges are a common hurdle when dealing with Cloudflare. Tools like FlareSolverr can help you tackle these effectively. Below is a simple Python implementation:
import requests
def solve_cloudflare(url):
flaresolverr_url = 'http://localhost:8191/v1'
payload = {
'cmd': 'request.get',
'url': url,
'maxTimeout': 60000
}
response = requests.post(flaresolverr_url, json=payload)
return response.json()
While solving CAPTCHAs is often necessary, maintaining ethical scraping practices should always remain a priority. Using these methods responsibly ensures you stay within acceptable boundaries.
sbb-itb-65bdb53
Advanced Methods to Avoid Detection
"Cloudflare's antibot protection system is sophisticated, but it is set up by humans who might not fully understand Cloudflare, might cut corners, or make mistakes when setting up their website on Cloudflare."
Mimicking Human Behavior
Cloudflare uses behavioral analysis to monitor patterns like mouse movements, timing between actions, and randomness in interactions. To bypass detection, it's crucial to mimic these human-like behaviors. The Puppeteer-extra library, paired with its stealth plugin, is a great tool for this purpose. Here's an example:
const puppeteer = require('puppeteer-extra')
const StealthPlugin = require('puppeteer-extra-plugin-stealth')
puppeteer.use(StealthPlugin())
async function scrapeStealth(url) {
const browser = await puppeteer.launch({ headless: true })
const page = await browser.newPage()
// Add random delays between actions
await page.setDefaultNavigationTimeout(30000)
await page.goto(url)
// Simulate natural mouse movements
await page.mouse.move(100, 200, { steps: 10 })
await page.waitForTimeout(Math.random() * 1000 + 500)
return await page.content()
}
By adding randomness to actions like mouse movements and interaction timings, you can simulate more realistic user behavior. However, this approach works best when combined with other techniques, such as customizing HTTP headers.
Changing HTTP Headers and User-Agent
Randomizing HTTP headers is another way to make your traffic appear more authentic. Here's an example in Python to rotate headers dynamically:
headers_pool = [
{
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) Chrome/118.0.0.0',
'Accept': 'text/html,application/xhtml+xml',
'Accept-Language': 'en-US,en;q=0.9',
'Accept-Encoding': 'gzip, deflate, br',
'Connection': 'keep-alive'
},
# Add more header combinations
]
def get_random_headers():
return random.choice(headers_pool)
Using a pool of headers and switching between them can help mimic diverse user traffic, making detection more challenging.
Using JavaScript Rendering
Cloudflare often relies on detecting incomplete or incorrect JavaScript execution to flag bots. Tools like FlareSolverr can help handle this by rendering JavaScript properly. Here's a simple implementation:
import requests
def render_with_flaresolverr(url):
solver_api = 'http://localhost:8191/v1'
payload = {
'cmd': 'request.get',
'url': url,
'maxTimeout': 60000
}
response = requests.post(solver_api, json=payload)
return response.json()['solution']
This method ensures JavaScript is fully executed, making it harder for Cloudflare to detect automated requests. Combining this with behavioral simulation and header randomization creates a more comprehensive approach to avoiding detection.
Comparing Tools and Services
Picking the right tool to bypass Cloudflare depends on your project's needs, complexity, and budget. Here's a breakdown of some popular tools and services, outlining their features, ideal use cases, drawbacks, and costs.
Tool and Service Comparison Table
Tool/Service | Key Features | Best For | Limitations | Relative Cost |
---|---|---|---|---|
FlareSolverr | • Docker support • Proxy server integration • Automates challenges |
Small to medium projects | Limited scalability | Free/Open-source |
Cloudscraper | • Python-native • Handles cookies • Solves basic challenges |
Python developers | Less reliable for complex sites | Free/Open-source |
Puppeteer | • Chrome DevTools Protocol • Stealth plugins • Lightweight |
JavaScript developers | Higher resource usage | Free/Open-source |
Selenium | • Multi-browser support • Extensive ecosystem • Language flexibility |
Large-scale operations | Slower than Puppeteer | Free/Open-source |
Web Scraping HQ | • Managed service • Enterprise-grade features • Legal compliance |
Enterprise solutions | Starts at $449/month | Premium |
Choosing the right option depends on the size and complexity of your project. For smaller projects, open-source tools like Cloudscraper or Puppeteer (especially with stealth plugins) are great choices. On the other hand, enterprise solutions like Web Scraping HQ offer managed services and advanced features for larger-scale operations.
When evaluating tools, think about how they integrate with your existing scraping setup and whether they support legal compliance. It's also important to look at how often the tool is updated and the level of community support available. Frequent updates help maintain compatibility with Cloudflare's evolving security, while active communities can provide tips, troubleshooting help, and solutions to common issues.
"Cloudflare's detection methods have evolved to include sophisticated IP blocking, user-agent detection, and TLS fingerprinting, making it essential to choose tools that can handle these challenges effectively."
Conclusion on how to bypass Cloudflare
"Bypassing Cloudflare's anti-bot protection is possible. However, it isn't an easy task." - ScrapeOps, "How To Bypass Cloudflare in 2024"
Web scraping has become more challenging with Cloudflare's robust security features, but it’s not impossible. Tools like Puppeteer and Selenium, when used correctly, can help you navigate these barriers and maintain access to protected websites. How to bypass Cloudflare is a significant problem while doing web scraping so it’s better to use Web Scraping Tools.
Key Takeaways
-
Infrastructure and Requests
- Use rotating proxies and vary request timing to mimic human behavior.
- Distribute requests intelligently to stay under the radar.
-
Automation Tools
- Leverage browser emulation tools to handle JavaScript-based challenges.
- Regularly update headers and user-agent strings.
-
Human-Like Interactions
- Simulate natural scrolling and realistic mouse movements.
- Ensure consistent patterns that resemble genuine user activity.
By applying these methods carefully, you can improve your chances of success while staying within ethical and legal boundaries. As Cloudflare continues to refine its systems, staying updated on new techniques is critical.
Balancing technical expertise with ethical practices ensures you can collect data effectively without causing harm to the websites or violating their policies. Adapting your approach over time keeps your scraping efforts efficient and compliant, allowing for reliable, long-term data access.
FAQs
How do I access a website without Cloudflare?
When trying to access a website protected by Cloudflare, different techniques can be used to improve success rates. Here's a quick comparison of some common approaches:
Method | Key Advantage | Success Rate |
---|---|---|
Rotating Proxies | Hides your IP address | 75-85% |
Browser Automation | Mimics human browsing behavior | 80-90% |
CAPTCHA Services | Solves challenges using AI or humans | 70-80% |
Combining these methods can greatly improve your ability to bypass Cloudflare's protections while sticking to ethical practices. Since Cloudflare updates its detection strategies frequently, it's important to stay informed about the latest tools and techniques.
Using Web Scraping APIs
Services like FlareSolverr make bypassing Cloudflare easier by automating the process. They use Puppeteer with stealth plugins to navigate Cloudflare's security layers, letting developers focus on building their scraping logic rather than solving security challenges manually.
It's crucial to use these methods responsibly, respecting the website's terms of service and legal guidelines. As Cloudflare continues to refine its defenses, keeping your tools up-to-date is key to maintaining effectiveness.
How do I get unblocked from Cloudflare?
Using Web Scraping APIs
Services like FlareSolverr make bypassing Cloudflare easier by automating the process. This is the best solution for how to bypass Cloudflare while doing webscraping.