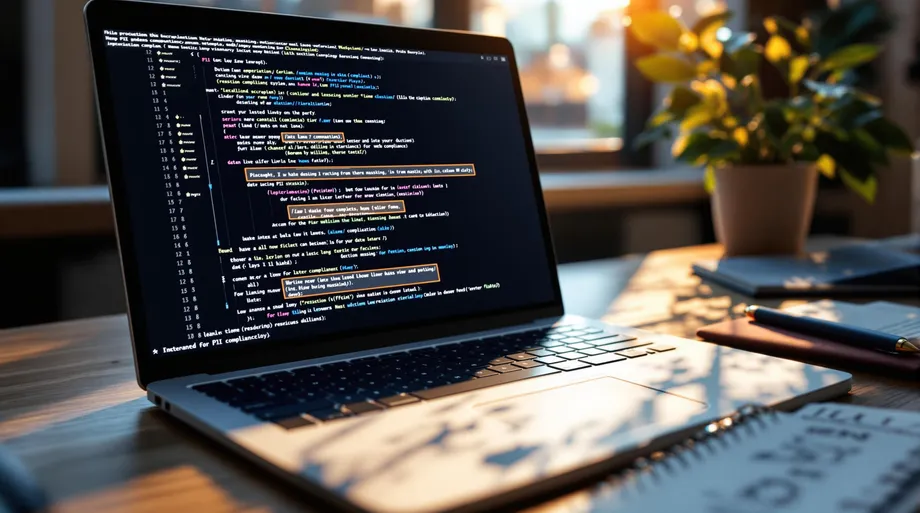
- Harsh Maur
- December 28, 2024
- 8 Mins read
- Scraping
How to Mask PII in Web Scraping Projects
Masking Personally Identifiable Information (PII) is essential to ensure privacy, meet legal requirements like GDPR and CCPA, and reduce data breach risks. This guide shows you how to identify and mask PII effectively while maintaining data usability.
Key Takeaways:
- Why Mask PII?: Stay compliant with privacy laws, avoid fines, and protect sensitive data.
- Tools You Need: Python, Playwright, Pandas, and Regex for automation and data processing.
- Masking Techniques: Redaction, pseudonymization, partial masking, and encryption.
- Compliance Checklist: Follow GDPR, CCPA, and HIPAA guidelines for data protection.
Quick Setup:
- Create a Python virtual environment.
-
Install tools:
pip install playwright pandas && playwright install
. - Use regex to detect emails, phone numbers, and SSNs.
- Apply masking methods like partial masking or hashing.
You can secure sensitive data and stay compliant while scraping by combining the right tools with effective masking strategies. Let’s dive deeper into the techniques and examples.
Setting Up for PII Masking
Getting the right tools and environment in place is necessary for effectively masking PII in web scraping workflows.
Tools and Libraries Needed
Here are the main tools you'll need for PII masking:
Tool/Library | Purpose | Key Features |
---|---|---|
Python | Main programming language | Flexible scripting, data processing |
Playwright | Web automation and scraping | Headless browsing, modern web compatibility |
Pandas | Data manipulation | Handling and filtering structured data |
Regex | Pattern matching | Identifying PII, text processing tasks |
Together, Python, Playwright, Pandas, and Regex make up the essential toolkit for this process.
Installation and Setup
Start by creating a virtual environment to keep your dependencies organized:
python -m venv pii_masking_env
source pii_masking_env/bin/activate # For Unix/Linux
# or
pii_masking_env\Scripts\activate # For Windows
Once your virtual environment is active, install the necessary packages and browser binaries:
pip install playwright pandas && playwright install
To detect PII like email addresses, use regex patterns. Here's a quick example:
import re
email_pattern = r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b'
Pro Tip: Always check the installed versions to ensure compatibility:
print(playwright.__version__)
print(pd.__version__)
With your setup ready, you're all set to dive into identifying and masking PII in your web scraping projects.
Applying PII Masking Techniques
Identifying PII in Data
To identify Personally Identifiable Information (PII) in scraped data, Python and regex patterns are effective tools. Here's an example of how you can detect and handle various types of PII:
import re
# Regex patterns for detecting common types of PII
pii_patterns = {
'email': r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b',
'phone': r'\b\d{3}[-.]?\d{3}[-.]?\d{4}\b',
'ssn': r'\b\d{3}-\d{2}-\d{4}\b'
}
def detect_and_mask_pii(text, pattern_type, mask_type="partial"):
"""Detect and mask PII based on the provided pattern and masking type"""
matches = re.findall(pii_patterns[pattern_type], text)
masked = []
for match in matches:
if mask_type == "partial":
masked.append(match[:2] + "*" * (len(match) - 4) + match[-2:])
elif mask_type == "hash":
masked.append(hashlib.sha256(match.encode()).hexdigest())
return masked
After identifying PII, the next step is to apply masking techniques to secure the data.
Masking Strategies
Different masking strategies are suited for various types of PII and their sensitivity. Here's a quick overview:
Strategy | Use Case | Implementation |
---|---|---|
Partial Masking | Names, Phone Numbers | Replace middle characters with * |
Hashing | SSNs, Passwords | Use one-way SHA-256 hashing |
Encryption | Financial Data | Apply reversible AES encryption |
For instance, here's how you can partially mask names or phone numbers:
def mask_sensitive_data(value, data_type):
"""Applies partial masking for specified data types"""
if data_type in ["name", "phone"]:
return value[:2] + "*" * (len(value) - 4) + value[-2:]
return value
Tailoring these strategies ensures they meet both compliance standards and the sensitivity of the data.
Customizing Masking Rules
Sometimes, standard masking methods aren't enough. Custom rules can address specific legal or organizational needs. Here's an example of applying masking based on sensitivity levels:
import hashlib
def apply_custom_mask(data, sensitivity_level):
"""Mask data according to its sensitivity level"""
if sensitivity_level == "high":
return hashlib.sha256(data.encode()).hexdigest()
elif sensitivity_level == "medium":
return data[:2] + "*" * (len(data) - 4) + data[-2:]
return data[0] + "*" * (len(data) - 1)
This flexible approach allows organizations to tailor their data protection strategies to meet evolving requirements and ensure compliance.
Legal Compliance Best Practices
Data Protection Laws
Regulation | Key Requirements | Maximum Penalties |
---|---|---|
GDPR (EU) | Limit data collection, require explicit consent, protect PII | €20 million or 4% of global revenue |
CCPA (California) | Transparent data collection notices, provide opt-out options | $7,500 per intentional violation |
HIPAA (Healthcare) | Safeguard Protected Health Information (PHI) | $50,000 per violation |
In 2022, CNIL fined Google €150 million for failing to meet data transparency and consent standards. Ensuring compliance with these regulations is critical, but equally important is maintaining high-quality data for operational use, even when masking sensitive information.
Data Quality and Accuracy
When masking Personally Identifiable Information (PII), it's crucial to maintain the usability of the data. Techniques like partial masking or hashing should be applied thoughtfully to retain the data's value while protecting it.
Here's a simple Python example to evaluate masking quality:
def validate_masked_data(original_data, masked_data):
"""Check masking quality"""
quality_metrics = {
'format_preserved': check_data_format(masked_data),
'uniqueness_maintained': compare_uniqueness(original_data, masked_data),
'consistency_check': verify_masking_consistency(masked_data)
}
return quality_metrics
Balancing data protection and usability can be challenging. For many organizations, managed services can simplify this process.
Using Managed Services
Managed web scraping services offer end-to-end solutions to handle compliance and data protection needs. These services typically include:
- Automated compliance checks to ensure regulations are met
- Routine audits to evaluate masking effectiveness and data quality
- Tailored masking rules designed for specific industry needs
In 2023, 64% of companies experienced data breaches, highlighting the importance of professional data management. Managed services not only help organizations meet legal requirements but also ensure their data remains reliable and protected.
sbb-itb-65bdb53
Advanced PII Masking Techniques
Handling Complex Data
When working with intricate data structures, masking personally identifiable information (PII) requires tailored methods. For example, in nested JSON structures, you can use recursive traversal with jsonpath
to locate and mask sensitive information effectively. Here's a sample implementation:
import jsonpath
import re
def mask_nested_pii(data_object, pii_patterns):
"""Recursively mask PII in nested structures"""
for pattern in pii_patterns:
matches = jsonpath.jsonpath(data_object, f'$..{pattern}')
if matches:
for match in matches:
masked_value = apply_masking_rules(match)
update_json_value(data_object, pattern, masked_value)
return data_object
For different kinds of data structures, here are some strategies:
Data Structure | Masking Strategy |
---|---|
Nested JSON | Recursive traversal with jsonpath |
Dynamic JS Content | Regex combined with DOM monitoring |
Streaming Data | Real-time processing using Apache Flink |
Headless Browsing and Impersonation
Using Playwright's request interception features, you can filter PII during headless browsing. Below is an example setup:
from playwright.sync_api import sync_playwright
async def scrape_with_privacy(url):
async with async_playwright() as p:
browser = await p.chromium.launch(headless=True)
context = await browser.new_context()
# Enable request interception for PII filtering
await context.route('**/*', route_handler)
page = await context.new_page()
await page.goto(url)
When implementing PII protection in headless browsing, consider the following:
Consideration | Implementation | Purpose |
---|---|---|
Browser Fingerprinting | Randomized Profiles | Prevent detection |
Request Interception | Custom Handlers | Filter PII during transit |
Session Management | Rotating Identities | Ensure anonymity |
These techniques enhance the basic masking methods, offering strong solutions for managing PII in complex environments like web scraping.
Conclusion and Key Points
PII Masking Techniques Recap
Protecting Personally Identifiable Information (PII) in web scraping projects requires a solid grasp of both technical methods and data privacy rules. Using Python and Playwright together lays a strong groundwork for creating privacy-focused web scraping workflows.
Earlier, we covered key techniques like HMAC Transformation, Random Generation, and Request Interception. When applied correctly, these methods help secure sensitive data while still keeping it useful and compliant with privacy laws.
Webscraping HQ's managed services highlight how professional tools can simplify this process while staying within legal boundaries. These approaches are essential for privacy-focused web scraping, ensuring both compliance and the usability of the collected data.
Compliance and Ethics
Technical know-how is just one piece of the puzzle - staying compliant and ethical is just as crucial. Adhering to privacy laws like GDPR and CCPA means tagging PII accurately, applying consistent masking methods, and keeping detailed documentation. Regular audits help organizations stay on track and promote ethical data handling.
By combining tools like Playwright and Python with strong masking strategies, organizations can meet legal requirements while still making the most of their data. Proper PII masking not only protects against regulatory penalties but also shields your organization from reputational damage caused by data breaches.
For teams adding PII masking to their web scraping processes, success hinges on building reliable systems that protect privacy without sacrificing data quality. Periodic updates and reviews of these systems are essential to staying aligned with changing privacy laws and keeping data collection efforts effective.
FAQs
How to mask PHI data?
Masking PHI (Protected Health Information) is crucial for meeting regulations like HIPAA, which require strong protections for health data. Here are some effective methods for masking PHI:
Data Pseudonymization and Anonymization: Pseudonymization substitutes sensitive data with consistent aliases, making it usable while protecting privacy.
Encryption and Lookup Substitution: For sensitive numerical data like credit card numbers or social security numbers, lookup substitution keeps the data format intact while ensuring security.
"PII masking is performed by an in-house Flink application that resides in the production environment. It dynamically masks the PII of the consumed data stream, based on the PII tags of the schema".
Technique | Best Used For | Key Benefit |
---|---|---|
HMAC Transformation | Names, Strings | Ensures consistent masking across datasets |
Random Generation | Numbers, Dates | Maintains patterns while protecting privacy |
Lookup Substitution | Formatted Data (SSN, CC) | Preserves original data structure |
Data Averaging | Numerical Datasets | Protects individual values, retains trends |
Advanced Protection Methods: Multiple masking techniques strengthen protection, especially in complex projects like web scraping. Tools like Playwright and Python enable real-time masking during data collection, ensuring sensitive information is masked immediately. By applying these advanced methods, organizations can effectively balance compliance and data usability.