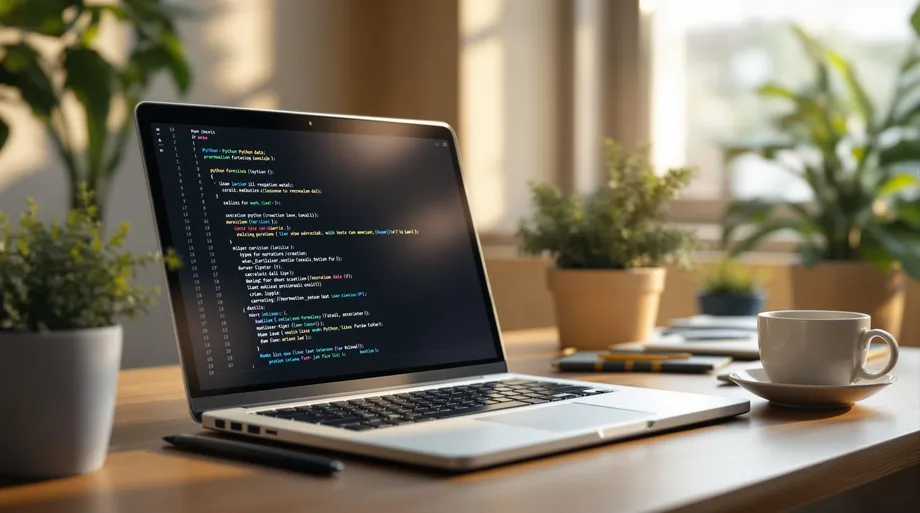
- Harsh Maur
- December 19, 2024
- 6 Mins read
- Scraping
How to Normalize Web Scraped Data with Python
Want to make messy web-scraped data clean and usable? Here's how you can quickly normalize it using Python. Normalization ensures your data is consistent, complete, and ready for analysis. Here's what you'll learn:
- Why normalization matters: Fix mixed formats, handle missing values, and remove duplicates.
- Tools you need: Python libraries like Pandas, NumPy, and scikit-learn.
- Essential steps: Import data, clean missing/duplicate entries, standardize formats, and handle outliers.
- Advanced tips: Merge datasets, validate data quality, and process JSON data.
Normalize JSON Dataset With pandas
Preparing Your Python Environment
Getting your Python environment ready is a key step when working with web scraped data. Here's what you'll need to set everything up.
Installing Required Libraries
Start by installing the core libraries you’ll use with pip:
pip install pandas numpy beautifulsoup4
- Pandas: Great for cleaning and organizing data.
- NumPy: Useful for numerical operations.
- BeautifulSoup4: Extracts information from HTML.
Once installed, test everything by importing the libraries in Python:
import pandas as pd
import numpy as np
from bs4 import BeautifulSoup
Setting Up Pandas for Data Normalization
Pandas is your go-to tool for transforming and cleaning scraped data. It offers features like fillna
to handle missing values, drop_duplicates
to remove repeated entries, and apply
for custom data modifications.
To avoid dependency conflicts, create a virtual environment for your project:
python -m venv scraping_env
source scraping_env/bin/activate # On Windows: scraping_env\Scripts\activate
With your environment set up, you're ready to dive into the process of normalizing your data effectively.
Steps to Normalize Web scraped Data
Importing and Reviewing Scraped Data
Start by loading your scraped data into a Pandas DataFrame. Use methods like head()
, info()
, and describe()
to get an overview of your dataset:
import pandas as pd
# Load data from CSV file
df = pd.read_csv('scraped_data.csv')
# Display the first few rows
print(df.head())
# Get dataset information
print(df.info())
# View statistical summary
print(df.describe())
-
info()
helps identify data types and missing values. -
describe()
provides a statistical summary of numerical columns.
This initial review helps pinpoint problems that need fixing.
Fixing Missing and Duplicate Data
Missing and duplicate entries can distort your analysis. Here's how to address them:
# Check for missing values in each column
missing_values = df.isnull().sum()
# Fill missing numerical values with the column mean
df['price'].fillna(df['price'].mean(), inplace=True)
# Fill missing categorical values with a default label
df['category'].fillna('Unknown', inplace=True)
# Remove duplicate rows based on a unique identifier
df.drop_duplicates(subset=['product_id'], keep='first', inplace=True)
The method you choose for handling missing data depends on the context of your dataset. For instance, numerical columns might use averages, while categorical columns could use default labels.
Standardizing and Transforming Data
Consistency is key when preparing data for analysis. Follow these steps to standardize and transform your dataset:
from sklearn.preprocessing import MinMaxScaler
# Standardize text data by converting to lowercase and stripping whitespace
df['product_name'] = df['product_name'].str.lower().str.strip()
# Convert date strings into datetime objects
df['scrape_date'] = pd.to_datetime(df['scrape_date'])
# Normalize numerical values using Min-Max scaling
scaler = MinMaxScaler()
df['price_normalized'] = scaler.fit_transform(df[['price']])
For text data, cleaning is essential to maintain uniformity:
def clean_text(text):
if isinstance(text, str):
return text.lower().strip().replace('\n', ' ').replace('\r', '')
return text
# Apply cleaning function to text columns
text_columns = ['title', 'description']
for col in text_columns:
df[col] = df[col].apply(clean_text)
These steps ensure your data is consistent, clean, and ready for analysis, making it easier to derive meaningful insights and integrate with other datasets.
sbb-itb-65bdb53
Advanced Techniques for Data Cleaning
Identifying and Handling Outliers
After standardizing your data, it's essential to pinpoint and manage outliers, as they can skew your analysis. Visualization tools like Matplotlib and Seaborn are great for spotting irregularities in your dataset:
import matplotlib.pyplot as plt
import seaborn as sns
# Create a box plot to identify outliers
plt.figure(figsize=(10, 6))
sns.boxplot(data=df['price'])
plt.title('Price Distribution with Outliers')
plt.show()
Once you've identified outliers, you can either remove them or adjust their values. Below are two common approaches:
# Method 1: Remove outliers using the Interquartile Range (IQR)
Q1 = df['price'].quantile(0.25)
Q3 = df['price'].quantile(0.75)
IQR = Q3 - Q1
df_clean = df[~((df['price'] < (Q1 - 1.5 * IQR)) | (df['price'] > (Q3 + 1.5 * IQR)))]
# Method 2: Cap outliers at specific percentile values
df['price_capped'] = df['price'].clip(lower=df['price'].quantile(0.01),
upper=df['price'].quantile(0.99))
Combining Data from Multiple Sources
If you're working with multiple datasets, merging them efficiently is key to maintaining consistency and ensuring completeness. Here's an example of combining datasets:
# Example: Merging product details with pricing data
product_details = pd.read_csv('product_details.csv')
product_prices = pd.read_csv('product_prices.csv')
# Merge datasets
combined_data = pd.merge(
product_details,
product_prices,
on='product_id',
how='left',
# Ensure a one-to-one relationship between datasets
validate='1:1'
)
This approach ensures that all relevant information is consolidated, making the dataset more reliable for analysis.
Checking the Quality of Cleaned Data
Once your data is cleaned and normalized, it's important to validate its quality. This ensures your dataset is accurate and ready for further analysis. Here's a quick function to assess key quality metrics:
def validate_dataset(df):
missing_percentage = (df.isnull().sum() / len(df)) * 100
duplicate_count = df.duplicated().sum()
data_types = df.dtypes
return {
'missing_percentage': missing_percentage,
'data_types': data_types,
'duplicate_count': duplicate_count
}
# Run validation checks
validation_results = validate_dataset(df)
Focus on these core aspects when validating your data:
Validation Type | Purpose | Example Check |
---|---|---|
Completeness | Ensure no key data is missing | Check null value percentages |
Consistency | Verify data follows expected patterns | Validate date formats or ranges |
Accuracy | Confirm data aligns with expected values | Check price ranges and currency formats |
Uniqueness | Detect duplicate records | Ensure unique identifiers are intact |
Conclusion
Key Steps in Data Normalization
The process of data normalization includes importing and reviewing your dataset, addressing missing or duplicate entries, standardizing formats, and verifying data quality. These steps help ensure your data is clean and consistent, making it ready for analysis. Tools like Python's Pandas library, combined with visualization tools such as Matplotlib and Seaborn, streamline this process, making data cleaning and transformation more efficient.
Applications of Normalized Data
Normalized data opens up a range of possibilities for businesses and analysts. It allows for accurate price tracking, detailed trend analysis, and better performance in machine learning models by ensuring data consistency. In price monitoring, for example, standardized data makes it easier to track product price changes across markets. For market research, normalized data helps identify patterns and trends, offering insights into consumer behavior and market shifts.
In machine learning, normalized data enhances model accuracy and interpretability. Scaling variables to a common range avoids issues where certain features dominate others, leading to more reliable and understandable models.
Additional Resources and Tools
For advanced or repetitive normalization tasks, external tools and platforms can be a big help. If you're working on large-scale web scraping projects, services like Webscraping HQ offer managed solutions that handle extraction and normalization efficiently while ensuring compliance and quality.
To deepen your understanding and skills in data normalization, you might want to explore:
- Advanced Pandas methods for managing complex datasets
- Statistical techniques for detecting and managing outliers
- Data validation frameworks to maintain high data quality
- Automated pipelines for recurring data cleaning tasks
Normalization is not a one-time task - it evolves as your data needs grow. The tools and techniques outlined here provide a strong starting point for managing and preparing web-scraped data effectively.
FAQs
How to Normalize JSON Data in Python
Web scraping often results in nested JSON data, which needs to be converted into a tabular format for easier analysis. The Pandas json_normalize
function is perfect for turning nested JSON structures into a DataFrame, simplifying data processing and analysis.
Here’s an example:
from pandas import json_normalize
import json
# Load nested JSON data
data = json.loads('{"product": {"id": 1, "name": "Widget", "price": 19.99}, "category": "tools"}')
# Flatten JSON into a DataFrame
df = json_normalize(data)
print(df)
This function works with nested dictionaries, lists, and arrays, automatically generating column headers based on the JSON structure, Once flattened, the data can be easily used with Pandas for tasks like filtering, grouping, and aggregation.
For web-scraped data, combining json_normalize
with other Pandas tools can create an efficient data-cleaning pipeline. This normalized structure simplifies your analysis and ensures your data is ready to use.