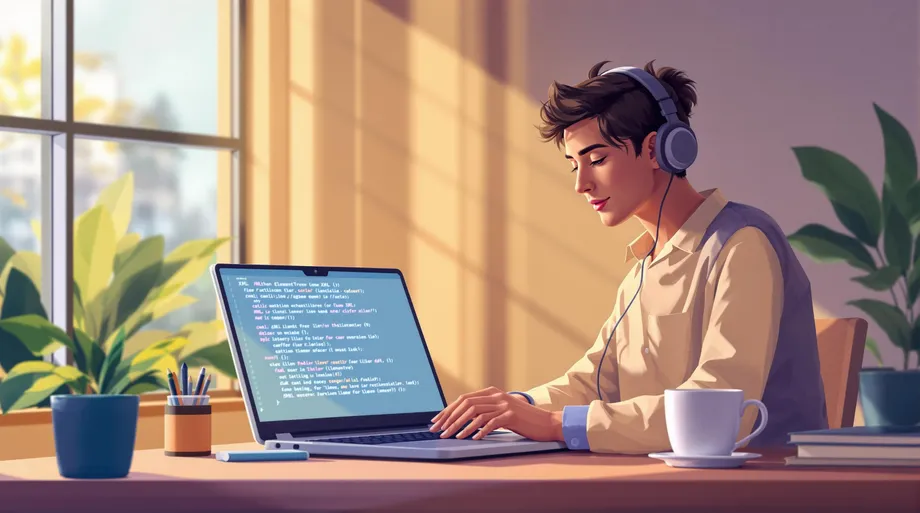
- Harsh Maur
- January 8, 2025
- 8 Mins read
- Scraping
How to Parse XML with Python ElementTree in 2025
Parsing XML in Python is simple and efficient with the built-in ElementTree library. It allows you to navigate, extract, and manipulate XML data easily. Here's you need to know about How to Parse XML with Python:
- Why Parse XML? XML organizes data in a tree-like structure, making it ideal for tasks like handling web services, reading configuration files, or processing datasets.
- Why ElementTree? It's built into Python, lightweight, and mirrors XML's structure for easy navigation.
-
How It Works: Load XML from a file or string, traverse the tree, and extract elements using methods like
find()
,findall()
, andget()
.
Quick Example:
import xml.etree.ElementTree as ET
tree = ET.parse('example.xml')
root = tree.getroot()
for elem in root:
print(elem.tag, elem.attrib, elem.text)
This guide covers everything from basic parsing to handling complex XML structures and optimizing performance for large datasets.
Parse XML Files with Python
Setting Up Python ElementTree
ElementTree is included in Python's standard library, making it a handy tool for parsing XML. Here's how to get started with it.
Installing and Importing ElementTree
Since ElementTree is built into Python, there's no need for additional installation. You can import it directly with this line:
import xml.etree.ElementTree as ET
This gives you access to ElementTree's XML parsing capabilities. Let's move on to loading and parsing XML data.
Loading and Parsing an XML File
ElementTree provides two main ways to handle XML data: loading it from a file or parsing it from a string. Here's how each method works:
# Method 1: Load XML from a file
tree = ET.parse('config.xml')
root = tree.getroot()
# Method 2: Parse XML from a string
root = ET.fromstring('<root><item>Value</item></root>')
Once the XML data is loaded, you can start exploring its structure and extracting elements.
Accessing XML Elements
ElementTree makes navigating XML structures straightforward with its built-in methods. Here's a quick overview:
Method | What It Does |
---|---|
find() |
Retrieves the first matching element, e.g., root.find('element_name') |
findall() |
Retrieves all matching elements, e.g., root.findall('element_name') |
get() |
Fetches the value of an attribute, e.g., element.get('attribute_name') |
Here's an example to show these methods in action:
import xml.etree.ElementTree as ET
tree = ET.parse('products.xml')
root = tree.getroot()
# Find a specific element
first_product = root.find('product')
product_id = first_product.get('id')
# Find all price elements
prices = root.findall('.//price')
for price in prices:
print(price.text)
This approach makes it easy to navigate XML data, whether you're working with simple files or more complex structures. The tree-like layout of ElementTree aligns naturally with XML, allowing you to extract the data you need with minimal effort.
Navigating and Extracting Data from XML
Understanding the XML Tree Structure
ElementTree mirrors XML's parent-child relationships, making it easier to navigate and pull out data. Here's a quick example to illustrate:
import xml.etree.ElementTree as ET
# Sample XML structure
xml_data = '''
<library>
<book category="fiction">
<title>The Great Gatsby</title>
<author>F. Scott Fitzgerald</author>
<year>1925</year>
</book>
<book category="non-fiction">
<title>Clean Code</title>
<author>Robert C. Martin</author>
<year>2008</year>
</book>
</library>
'''
# Parse the XML string
root = ET.fromstring(xml_data)
# Navigate through the tree
for book in root:
print(f"Category: {book.get('category')}")
for element in book:
print(f"{element.tag}: {element.text}")
print("---")
This example shows how to move through the tree structure and access each element.
Extracting Specific Data
Once you're familiar with navigating the XML, you can use methods like .text
(for content), .attrib
(for attributes), and .find()
(for specific elements) to extract the data you need. Here's how it works:
import xml.etree.ElementTree as ET
tree = ET.parse('data.xml')
root = tree.getroot()
# Using XPath to target specific elements
books = root.findall(".//book[@category='fiction']")
for book in books:
title = book.find('title').text
author = book.find('author').text
print(f"Found: {title} by {author}")
# Extracting nested data
for book in root.iter('book'):
# Get all attributes
attributes = book.attrib
# Get nested elements
details = {child.tag: child.text for child in book}
print(f"Book details: {details}")
When working with XML, it's important to handle missing data carefully to avoid errors. These techniques provide a solid starting point for managing more intricate XML structures.
sbb-itb-65bdb53
Handling Complex XML Structures
As XML files become more intricate, knowing how to handle nested and irregular structures is key to efficient data parsing.
Working with Nested Elements
For deeply nested XML structures, Python's ElementTree
module offers tools to navigate multiple hierarchy levels effectively:
import xml.etree.ElementTree as ET
xml_data = '''
<organization>
<department name="engineering">
<team name="backend">
<employee>
<details>
<name>John Smith</name>
<role>Senior Developer</role>
<projects>
<project>Database Migration</project>
<project>API Development</project>
</projects>
</details>
</employee>
</team>
</department>
</organization>
'''
root = ET.fromstring(xml_data)
# Navigate through the nested structure
for employee in root.findall('.//employee'):
name = employee.find('.//name').text
role = employee.find('.//role').text
projects = [p.text for p in employee.findall('.//project')]
print(f"Employee: {name}")
print(f"Role: {role}")
print(f"Projects: {', '.join(projects)}")
Managing Irregular Data
To handle missing or inconsistent XML elements, use a function that extracts data safely:
def safe_extract(element, path, default="Not specified"):
try:
result = element.find(path)
return result.text if result is not None else default
except AttributeError:
return default
# Example usage
team_name = safe_extract(dept, './/team/@name')
This approach ensures your code doesn't break when elements are absent or incorrectly formatted.
Troubleshooting XML Parsing Errors
Parsing errors are common with XML. Here's how to manage them effectively:
try:
tree = ET.parse('complex_data.xml')
except ET.ParseError as e:
print(f"XML Parsing Error: {str(e)}")
error_line = e.position[0]
print(f"Error occurred at line: {error_line}")
For large XML files, use iterparse()
to process data efficiently without overloading memory:
context = ET.iterparse('large_file.xml', events=('start', 'end'))
for event, elem in context:
if event == 'end' and elem.tag == 'target_element':
process_element(elem)
elem.clear() # Free memory by clearing processed elements
These techniques help you manage complex XML structures and large datasets efficiently [1][2]. Next, we'll dive into optimizing XML parsing workflows to improve performance.
Advanced Techniques and Best Practices
Using XPath for Precise Element Selection
XPath is a powerful tool for selecting specific elements in XML documents. Here's an example using Python's ElementTree:
import xml.etree.ElementTree as ET
xml_data = '''
<bookstore>
<book genre="fiction">
<title>The Great Gatsby</title>
<price>9.99</price>
</book>
<book genre="non-fiction">
<title>Data Science Handbook</title>
<price>29.99</price>
</book>
</bookstore>
'''
root = ET.fromstring(xml_data)
# Find books by genre or price
books = root.findall('.//book[@genre="fiction" or price>20]/title')
for book in books:
print(f"Book: {book.text}")
This example demonstrates how to locate specific books based on their genre or price. While XPath provides precision, it's equally important to ensure your XML parsing approach is efficient, especially when working with large datasets.
Improving XML Parsing Efficiency
When dealing with large XML files containing thousands of records, optimizing your parsing process can save memory and improve performance. Here's how:
def optimize_xml_parsing(filename):
context = ET.iterparse(filename, events=('start', 'end'))
for event, elem in context:
if event == 'end' and elem.tag == 'target_element':
process_data(elem)
elem.clear() # Free memory
This method processes elements as they are parsed, clearing them from memory once handled. It's particularly useful for applications requiring high performance and minimal resource usage.
XML Parsing in Business Applications
Combining XPath's targeting capabilities with efficient parsing methods allows businesses to process XML feeds for applications like e-commerce or real estate. Here's an example for parsing product feeds:
def parse_product_feed(xml_file):
tree = ET.parse(xml_file)
root = tree.getroot()
return [
{
'sku': product.find('.//sku').text,
'name': product.find('.//name').text,
'price': product.find('.//price').text,
'stock': product.find('.//inventory/quantity').text
}
for product in root.findall('.//product')
]
Using streaming techniques in scenarios like this can significantly reduce memory usage - by as much as 90% - compared to loading the entire XML file into memory [1][2]. This ensures efficient and reliable data processing without compromising performance or code quality [4].
Conclusion and Key Takeaways
Key Steps on How to Parse XML with Python
Python's ElementTree library offers a clear and structured way to handle XML parsing. The process involves importing the library, loading your XML data, and navigating through the tree structure to retrieve the information you need. Here’s why it stands out:
- It creates an easy-to-understand tree representation of XML documents, simplifying navigation and supporting both file-based and string-based parsing.
- Since it's part of Python's standard library, it provides dependable performance without requiring additional dependencies.
With these basics in place, you can see how XML parsing can be applied across a range of industries.
Practical Uses of XML Parsing
XML parsing with ElementTree plays a significant role in various business scenarios. Here are some common applications:
Application Area | Example | Benefit |
---|---|---|
E-commerce | Automating product feed updates | Streamlined inventory management |
Digital Libraries | Processing XML archives | Improved metadata categorization |
API Integration | Handling XML responses | Real-time data updates |
Scientific Research | Transforming XML datasets | Automated analysis |
These examples highlight how XML parsing can address diverse needs, but ensuring efficient performance is key when dealing with larger datasets.
Next Steps
To build on the techniques discussed earlier, focus on these advanced strategies and performance tips:
Advanced Techniques:
- Learn to use XPath for precise element selection.
- Apply streaming methods for handling large XML files.
- Develop strong error-handling mechanisms to manage unexpected issues.
Performance Tips:
- Opt for iterative parsing to save memory when processing large files.
- Use element clearing to avoid excessive resource consumption.
- Write clean, maintainable code to simplify long-term updates and scaling.
For businesses working with multiple data formats beyond XML, platforms like Web Scraping HQ offer specialized tools to tackle even the most complex data extraction challenges.
FAQs on How to Parse XML with Python
Here are answers to some common questions about using Python's ElementTree for XML parsing, based on the techniques covered earlier.
Which Python module is best for parsing XML documents?
ElementTree is a built-in library in Python that makes XML parsing straightforward. It converts XML documents into a tree structure that's easy to navigate. Since it's part of Python's standard library, there's no need for extra installations. ElementTree is a great choice for handling XML parsing tasks right away [3].
How to Parse XML with Python ElementTree?
To parse XML files or strings, you can use parse()
for files or fromstring()
for strings. Here's an example:
import xml.etree.ElementTree as ET
# Parsing an XML file
tree = ET.parse('example.xml')
# Parsing an XML string
root = ET.fromstring(xml_string)
Both methods load the XML data into a tree structure for further processing [1][2].
How can you read a specific tag in an XML file with Python?
Here's a quick example:
import xml.etree.ElementTree as ET
# Load the XML file
tree = ET.parse('example.xml')
root = tree.getroot()
# Find specific elements
elements = root.findall('.//desired_tag')
This allows you to extract data from specific tags within the XML file [2].
What’s the difference between ElementTree and Element in Python XML?
ElementTree represents the entire XML document, while Element refers to individual nodes within that document. Use ElementTree for operations on the whole document and Element for tasks focused on specific nodes. Understanding this distinction helps you navigate and manipulate XML data more effectively.
How do you find an element in XML using Python?
To locate elements by tag or attribute, you can use the findall()
method with XPath. For example:
root.findall('.//tag[@attribute="value"]')
This approach helps you pinpoint elements based on specific tags or attributes [2][4].