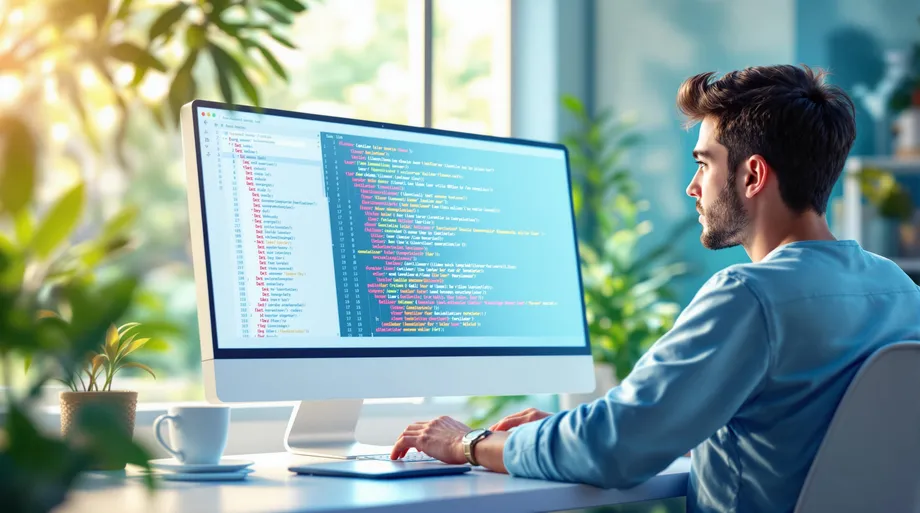
- Harsh Maur
- December 21, 2024
- 7 Mins read
- Scraping
How to Traverse Complex DOM Structures in JavaScript
Want to navigate complex web pages effortlessly?
DOM traversal in JavaScript is the key to working with HTML structures, whether you're dynamically updating content, extracting data, or building interactive features. Here's what you'll learn:
- Why DOM Traversal Matters: Essential for dynamic content updates, data extraction, and creating responsive web apps.
-
Core Methods: Use
parentNode
,childNodes
,firstElementChild
, andnextElementSibling
for precise navigation. - Practical Examples: Handle nested elements, extract product details, and manage dynamic content changes.
-
Advanced Techniques: Combine traversal methods, cache elements for performance, and use
MutationObserver
for real-time updates. - Common Pitfalls: Avoid text node interference, performance issues, and memory leaks with simple strategies.
This guide breaks down everything you need to know about traversing DOM structures efficiently, with clear examples and actionable tips.
Learn JavaScript DOM Traversal In 15 Minutes
Key Methods for DOM Traversal
JavaScript makes it straightforward to navigate through the DOM tree. Here's a breakdown of the key methods you can use to move around the DOM structure.
Navigating Parent and Child Nodes
To move vertically through the DOM tree, you can use parentNode
it to find an element's parent and childNodes
to get all child nodes as a NodeList. For more specific access, firstChild
and lastChild
allow you to pinpoint the first and last child nodes.
// Get the parent of a specific element
const productTitle = document.querySelector('.product-title');
const productContainer = productTitle.parentNode;
// Retrieve all child nodes of a container
const productList = document.querySelector('.product-list');
const allProducts = productList.childNodes;
Navigating Sibling Nodes
To move horizontally, use nextSibling
and previousSibling
. However, keep in mind that these methods include text nodes, which might lead to unexpected results if your HTML contains whitespace.
Element-Specific Methods for Cleaner Navigation
For more precise traversal, JavaScript offers methods that focus only on element nodes, skipping over text nodes entirely:
Method | Description |
---|---|
firstElementChild |
Retrieves the first child element, ignoring text nodes |
lastElementChild |
Retrieves the last child element, ignoring text nodes |
nextElementSibling |
Finds the next sibling element, skipping over whitespace |
previousElementSibling |
Finds the previous sibling element, skipping over whitespace |
const container = document.querySelector('.container');
const firstProduct = container.firstElementChild;
const nextProduct = firstProduct.nextElementSibling;
The structure of your DOM tree will influence which methods work best for your needs. These tools are essential for navigating and manipulating the DOM effectively. Let’s dive into practical examples to see them in action.
Examples of DOM Traversal in Action
Let's dive into how DOM traversal techniques can be applied to handle complex structures and extract data efficiently.
Working with Nested Elements
Here's an example of navigating a nested product catalog using a recursive approach:
function traverseProductCatalog(element) {
// Check if the element exists
if (!element) return;
// Process the current element
if (element.classList.contains('product')) {
const productName = element.querySelector('.product-name')?.textContent;
const productPrice = element.querySelector('.price')?.textContent;
console.log(`Found product: ${productName} - ${productPrice}`);
}
// Recursively process child elements
const children = element.children;
for (let child of children) {
traverseProductCatalog(child);
}
}
// Start traversal from the main catalog container
const catalog = document.querySelector('.product-catalog');
traverseProductCatalog(catalog);
This method ensures that no nested elements are skipped, making it ideal for deeply layered DOM structures. While recursion works well for such scenarios, combining it with other traversal techniques can make data extraction even more effective.
Extracting Specific Data
Using a mix of traversal methods can improve both accuracy and efficiency. Here's an example that extracts product details from a complex e-commerce layout:
function extractProductDetails() {
const productContainer = document.querySelector('.product-grid');
const products = [];
try {
const productElements = productContainer.querySelectorAll('.product-card');
productElements.forEach(product => {
// Access specific child elements
const title = product.firstElementChild?.querySelector('.title')?.textContent;
// Traverse to parent elements for related data
const priceContainer = product.querySelector('.price-tag');
const price = priceContainer?.parentNode?.querySelector('.amount')?.textContent;
// Use sibling traversal for additional details
const description = priceContainer?.nextElementSibling?.textContent;
products.push({ title, price, description });
});
} catch (error) {
console.error('Error extracting product details:', error);
}
return products;
}
This example highlights several useful techniques:
-
Using
querySelector
for precise element selection - Combining parent, child, and sibling traversal methods
- Adding error handling for reliability
-
Using optional chaining (
?.
) to avoid null value issues
For dynamic content, you can use MutationObserver
To track changes in the DOM and trigger data extraction when new elements are added:
const observer = new MutationObserver(mutations => {
mutations.forEach(mutation => {
if (mutation.type === 'childList') {
const newProducts = extractProductDetails();
updateProductDisplay(newProducts);
}
});
});
// Start observing the product container
observer.observe(document.querySelector('.product-grid'), {
childList: true,
subtree: true
});
These examples demonstrate how to efficiently navigate and extract data from complex DOM structures using various traversal techniques.
sbb-itb-65bdb53
Advanced DOM Traversal Techniques
Combining Multiple Methods
Mixing various traversal methods with caching can boost performance when dealing with complex DOM structures. Caching elements you access often helps avoid redundant DOM queries, which is especially useful in large or intricate setups.
function traverseComplexStructure(rootElement) {
const cache = new Map();
function findElementsByAttribute(element, attribute) {
const cacheKey = `${element.id}-${attribute}`;
if (cache.has(cacheKey)) return cache.get(cacheKey);
const results = [];
const candidates = element.querySelectorAll(`[${attribute}]`);
candidates.forEach(candidate => {
if (element.contains(candidate)) results.push(candidate);
});
cache.set(cacheKey, results);
return results;
}
return findElementsByAttribute;
}
This example combines querySelectorAll
with contains
to locate elements efficiently within a specific scope. Adding caching ensures that repeated queries are faster. This method works well for dynamic content where DOM structures are constantly updated.
Handling Dynamic Content
Managing dynamic content requires tracking real-time changes in the DOM and reacting efficiently. Here's how you can do that:
function handleDynamicContent(targetNode, callback) {
const config = {
childList: true,
subtree: true,
attributes: true,
characterData: true
};
const observer = new MutationObserver((mutations) => {
callback(mutations);
});
observer.observe(targetNode, config);
return {
stop: () => observer.disconnect(),
resume: () => observer.observe(targetNode, config)
};
}
This setup uses a MutationObserver
To monitor changes in the DOM, such as added or removed elements, attribute modifications, or text updates. You can pause or resume the observation as needed.
Avoiding Common Issues
When working with DOM traversal, certain pitfalls are common. Addressing these proactively ensures smoother performance and fewer bugs. Here's a quick guide:
Issue | Solution & Implementation |
---|---|
Text Node Interference | Use element-specific methods like firstElementChild instead of firstChild |
Performance Degradation | Cache frequently accessed elements and DOM queries |
Memory Leaks | Disconnect MutationObserver instances when they're no longer needed |
Whitespace Handling | Filter nodes using nodeType === Node.ELEMENT_NODE |
To handle these challenges, you can use defensive programming techniques:
function safeTraversal(element) {
if (!element) return null;
if (element.nodeType !== Node.ELEMENT_NODE) {
return element.parentElement;
}
const tagName = element.tagName.toLowerCase();
const children = Array.from(element.children);
return {
element,
tagName,
children,
hasChildren: children.length > 0
};
}
This function ensures safe traversal by validating the node type and providing structured information about the element and its children. It’s a practical way to avoid unexpected errors while navigating the DOM.
Conclusion and Tips for DOM Traversal
Summary of Key Points
Traversal Need | Recommended Method | Performance Impact |
---|---|---|
Single Element Selection | querySelector() |
High - Direct Targeting |
Multiple Elements | querySelectorAll() with caching |
Medium - Balanced approach |
Dynamic Content | MutationObserver with selective monitoring |
Low - Resource-intensive |
Nested Structures | Combination of parent/child methods with caching | Medium - Context-dependent |
Tips for Efficient DOM Traversal
When working with complex DOM structures, efficiency is key. These strategies can help improve performance:
- Cache Nodes: Store frequently accessed elements in variables to avoid repeated lookups.
-
Be Specific: Use precise selectors instead of broad ones. For example, replace
document.getElementsByTagName('div')
withdocument.querySelector('#specific-id > .specific-class')
for better accuracy and performance. - Validate Nodes: Always check that a node is valid before accessing its properties to avoid errors. Here's a simple example:
function safeNodeAccess(node) {
return node?.nodeType === Node.ELEMENT_NODE
? node
: null;
}
- Monitor Performance: Use tools like the Performance API to identify bottlenecks and optimize your code.
For larger projects, specialized tools can make DOM traversal and data extraction faster and more efficient.
How Webscraping HQ Can Help
If you're dealing with challenges like dynamic content or deeply nested structures, Webscraping HQ provides solutions that go beyond manual DOM traversal. Their tools are designed to handle tasks such as:
- Dynamic content loading
- Navigating complex nested structures
- Collecting data across different domains
- Managing rate limits and access restrictions
Webscraping HQ also ensures high data quality and compliance with legal standards, delivering structured outputs in formats like JSON or CSV. Their services save time and effort, making them a great option for handling complex web scraping needs.
FAQs
What happens here in the first DOM structure?
DOM traversal starts with the document
object, which acts as the entry point to all nodes in the DOM tree. This is the starting point for navigating both basic and complex DOM structures.
// Starting from document root
const rootElement = document.documentElement;
// Accessing first child in DOM hierarchy
const firstChild = rootElement.firstElementChild;
// Checking node type before operations
if (firstChild?.nodeType === Node.ELEMENT_NODE) {
console.log("Valid element node found");
}
This method is especially useful when you need to work with key root elements like <html>
or <body>
to kick off DOM manipulation tasks.
Understanding node types is key when traversing the DOM. Here's a quick breakdown of commonly used methods:
Method | Returns | Use Case |
---|---|---|
firstChild |
Any node type, including text nodes | General node traversal |
firstElementChild |
Only element nodes | Focusing on element nodes |
querySelector |
The first matching element | Directly selecting specific elements |
If you're only interested in element nodes and want to skip over text nodes, go with firstElementChild
. Always make sure to validate nodes before accessing their properties to avoid errors during traversal.
These basic techniques form the foundation for working with more intricate structures, including nested or dynamic content. They are essential tools for tackling advanced DOM traversal tasks discussed further in this guide.