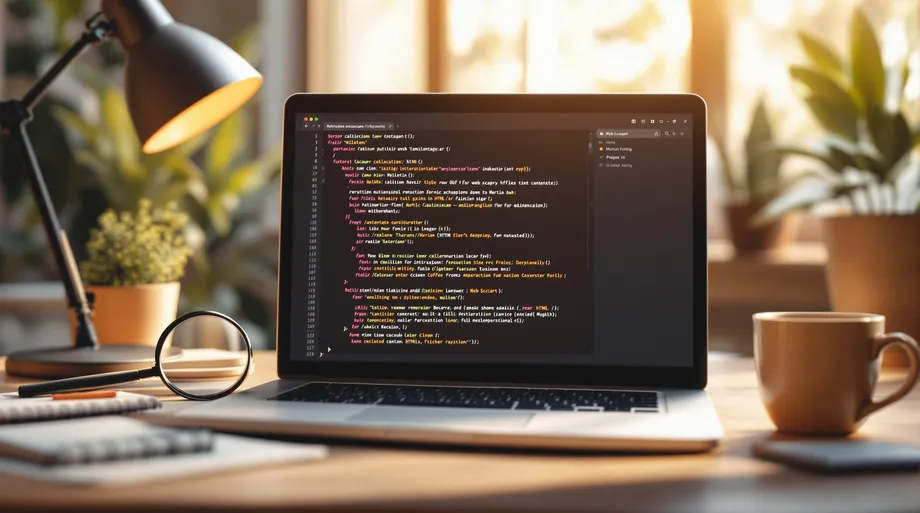
- Harsh Maur
- December 26, 2024
- 8 Mins read
- Scraping
Basics of HTML Web Scraping
Want to scrape data from websites? Understanding HTML is the first step. HTML organizes web pages using tags and attributes, making it possible to extract specific data.
Key Takeaways:
-
HTML Structure: Learn how tags (like
<div>
and<span>
), attributes (class
,id
), and nesting help locate data. -
Tools You’ll Use: Browser Inspect tools, Python libraries (
BeautifulSoup
,requests
), and advanced options like Selenium for dynamic content. - Scraping Techniques: Use CSS/XPath selectors to extract data, handle errors, and scrape multiple pages.
-
Legal & Ethical Practices: Respect
robots.txt
, avoid overloading servers, and follow site terms.
This guide covers everything from basic HTML concepts to advanced Python-based scraping techniques. Whether you’re extracting product prices or handling dynamic pages, this is your go-to resource.
Inspecting Web Pages with HTML
HTML Basics and Structure
Now that we've gone over what HTML is and why it's important for web scraping, let's break down its structure and key components that make data extraction possible.
HTML Tags and Elements Explained
An HTML document is made up of elements, defined by tags. These tags tell the browser how to organize and display content. Most elements consist of an opening tag, the content itself, and a closing tag:
<tag>Content goes here</tag>
For web scraping, knowing this structure is essential because it helps you pinpoint the data you want. Some common elements you'll often run into include:
<div>Container for other elements</div>
<p>Paragraph text</p>
<a href="url">Link text</a>
<table>Data in rows and columns</table>
Understanding Attributes in HTML
Attributes add extra details to elements and are key to locating data during scraping. These attributes are included in the opening tag and often serve as identifiers:
<div id="main-content" class="container">
<span class="price">$29.99</span>
</div>
Two attributes you’ll frequently rely on are id
and class
, as they make it easier to find specific elements on a page.
HTML Hierarchy and Nesting
HTML follows a hierarchical layout where elements are nested within each other. This parent-child relationship is critical for navigating web pages during scraping:
<article class="product-listing">
<h2>Product Title</h2>
<div class="details">
<span class="price">$99.99</span>
<span class="stock">In Stock</span>
</div>
</article>
This hierarchy allows you to create precise selectors that target specific pieces of data while keeping their context intact. For example, you can start at a parent element and work your way down to its children to ensure you're capturing the right information.
Features for HTML Web Scraping
Certain HTML features make web scraping more efficient and precise. Knowing how to use browser tools and selectors can help you extract data with accuracy.
Using IDs and Classes to Locate Elements
IDs and classes are essential for finding specific elements in HTML. An ID is unique to a page, making it perfect for targeting a single element. For example:
<div id="main-product-price">$299.99</div>
On the other hand, classes are often shared across multiple elements, making them useful for extracting repeated patterns:
<div class="product-card">...</div>
Understanding when to use IDs versus classes is key to effective scraping.
Inspecting HTML with Browser Tools
Browser developer tools, like Chrome Inspector, are invaluable for analyzing HTML and testing selectors before writing code. These tools let you:
- Explore element structures
- Test CSS or XPath selectors in real-time
- Understand how data is organized, even for responsive or dynamically loaded content [2]
For web scraping, this step can save time and ensure your selectors are accurate.
CSS and XPath Selectors for Scraping
Both CSS and XPath selectors help pinpoint elements in HTML. CSS selectors are straightforward and work well for class-based or nested elements:
-
.product-price
-
#main-content > div.item
XPath selectors, such as //div[@class="price"]/text()
, are more versatile for identifying elements based on their relationships within the HTML structure [1]. For example:
# Using CSS selector
prices = tree.cssselect('.item-price')
# Using XPath selector
prices = tree.xpath('//div[@class="item-price"]/text()')
XPath is particularly helpful for complex tasks, while CSS selectors are easier to use for simpler cases [1][2].
sbb-itb-65bdb53
Web Scraping with Python: Step-by-Step
Setting Up Python for Web Scraping
To get started with web scraping in Python, you'll need a few key libraries. These include:
import requests
from bs4 import BeautifulSoup
import lxml
-
BeautifulSoup
: Helps parse and navigate HTML documents. -
requests
: Handles HTTP requests to fetch web pages.
Install these libraries via pip:
pip install beautifulsoup4 requests lxml
Here’s a simple example to fetch and parse a webpage:
# Fetch webpage content
response = requests.get('http://example.com')
# Parse HTML with BeautifulSoup
soup = BeautifulSoup(response.content, 'lxml')
Once everything is set up, you can start pulling useful information from the HTML structure.
Extracting Data from HTML
When extracting data, it's important to handle errors gracefully. This ensures your script can adapt to missing elements or unexpected changes in the HTML.
# Extract data with error handling
try:
element = soup.find('div', class_='target')
text = element.text if element else 'Not found'
except AttributeError:
text = 'Error occurred'
# Extract multiple links
links = soup.find_all('a')
for link in links:
url = link.get('href')
text = link.text
# Extract images
images = soup.find_all('img')
for img in images:
src = img.get('src')
Working with Dynamic or Complex HTML
Some websites rely on JavaScript to load content, making static HTML parsing insufficient. In such cases, Selenium can help. Selenium automates browser actions, making it ideal for scraping JavaScript-heavy pages.
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# Initialize Chrome driver
driver = webdriver.Chrome()
driver.get('http://example.com')
# Wait for dynamic content to load
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.CLASS_NAME, "dynamic-content"))
)
# Find elements with specific conditions
elements = driver.find_elements(By.XPATH,
"//div[@class='item' and contains(@data-type, 'product')]")
To reduce the risk of detection, rotate user agents in your HTTP headers and add random delays between requests:
import time
from random import uniform
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36'
}
response = requests.get(url, headers=headers)
time.sleep(uniform(1, 3))
With these tools and techniques, you can handle both static and dynamic content, giving you the flexibility to scrape a wide range of websites effectively.
Advanced HTML Web Scraping Tips
Scraping Data from Multiple Pages
Handling pagination effectively is key to gathering all the data you need from multi-page websites. Here's a Python example using requests
and BeautifulSoup
:
def scrape_multiple_pages(base_url, max_pages):
all_data = []
for page in range(1, max_pages + 1):
url = f"{base_url}?page={page}"
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36'
}
time.sleep(2)
try:
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.content, 'lxml')
page_data = extract_page_data(soup)
all_data.extend(page_data)
except Exception as e:
print(f"Error on page {page}: {str(e)}")
continue
return all_data
This approach ensures you loop through pages without missing data. However, always make sure your scraping activities follow legal and ethical standards.
Legal and Ethical HTML Web Scraping
When scraping websites, it's crucial to act responsibly. Here are some best practices to follow:
-
Check the site's
robots.txt
file to understand allowed paths and crawl delays. - Introduce delays (1-3 seconds) between requests to avoid overloading servers.
- Use proper User-Agent headers to indicate your scraper's identity.
- Review and adhere to the website's terms of service.
- Ensure your activities don't negatively impact the site's performance.
By adhering to these principles, you can scrape data responsibly while minimizing risks.
Speeding Up Scraping with Multi-threading
If you're working with a large number of pages, multi-threading can boost efficiency. Here's a simple implementation:
from concurrent.futures import ThreadPoolExecutor
def scrape_with_threading(urls, max_workers=5):
with ThreadPoolExecutor(max_workers=max_workers) as executor:
results = list(executor.map(fetch_and_parse, urls))
return results
To use multi-threading effectively, keep these factors in mind:
- Monitor memory usage to avoid overloading your system.
- Handle errors in each thread to prevent crashes.
- Safely share data between threads to maintain consistency.
- Limit the number of requests sent simultaneously to avoid being blocked.
For large-scale projects, where multi-threading may become too complex, consider using managed services. These can simplify technical challenges while ensuring compliance and reliable data extraction [1][3].
Using Managed HTML Web Scraping Services
What Is Web Scraping HQ?
Scaling manual web scraping can be tricky, especially for large projects. That’s where Web Scraping HQ comes in. They offer data extraction services tailored to different industries, such as tracking product prices, analyzing real estate trends, monitoring job postings, and more. The goal? To help businesses make smarter decisions using reliable data.
Why Choose Managed Services?
Managed web scraping services tackle some of the biggest challenges in data extraction, especially when dealing with complex websites or large-scale projects. Here’s how they help:
- Streamlined Process: They manage the technical side, from handling complex HTML to scaling operations, so you don’t need extra infrastructure or custom coding.
- Reliable Data: You get consistent, accurate results without the hassle of fixing errors or inconsistencies.
- Legal Peace of Mind: Providers ensure compliance with web scraping laws, reducing the risk of legal issues for your business.
How Businesses Use Managed Scraping
Managed services take raw web data and turn it into actionable insights. With plans like the Standard option at $449/month or fully Custom solutions starting at $999/month, they offer enterprise-grade features with quick setup times.
Here are some ways businesses use these services:
- Market Research: Gather competitor pricing and product details to guide your strategy.
- Content Tracking: Monitor updates across multiple websites automatically.
- Lead Generation: Collect company data to support your sales efforts.
For businesses that need efficient, large-scale data solutions, managed web scraping services simplify the process and deliver results you can use right away. They’re a great addition to DIY efforts when projects get too big or complex.
Summary and Next Steps to HTML Web Scraping
Key Takeaways
Understanding HTML basics is crucial for effective web scraping. Success in web scraping relies on combining HTML expertise, Python tools, and following legal and ethical practices. This combination allows you to extract data efficiently and responsibly from any website.
Getting Started with HTML Web Scraping
Ready to dive into web scraping? Here's how to kick things off:
- Begin with simple projects to practice HTML inspection and using CSS selectors.
-
Set up Python tools like
requests
andlxml
to streamline data extraction. - For larger or more complex tasks, consider using managed services to handle scaling and maintain accuracy.
With these steps and the tools outlined in this guide, you're equipped to start exploring the world of web scraping.