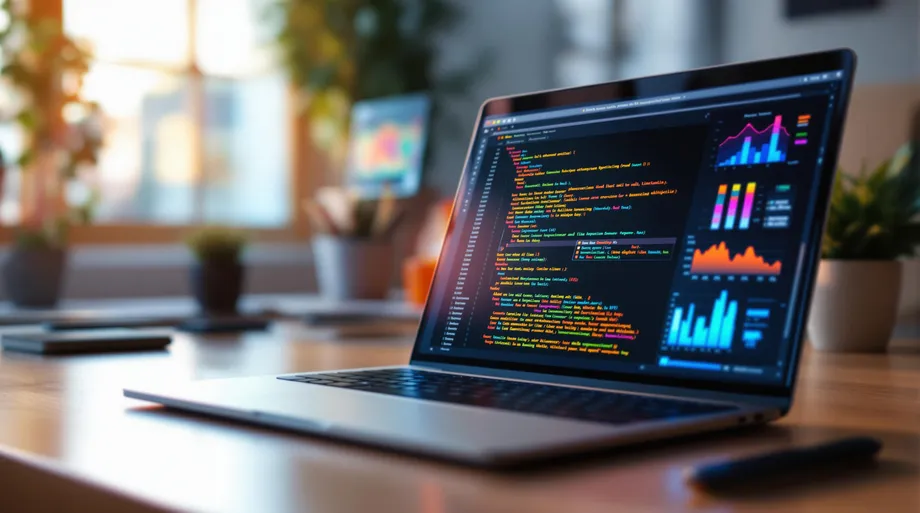
- Harsh Maur
- November 30, 2024
- 8 Mins read
- Scraping
Multi-Threading in Python Web Scraping
Want to scrape websites faster? Multi-threading in Python can help. It allows you to handle multiple web requests simultaneously, cutting down scraping time significantly. For example, 100 threads can reduce scraping 1,000 pages from 126 seconds to just 7 seconds. Here's what you'll learn:
- Why multi-threading works for web scraping: It’s ideal for I/O-bound tasks like HTTP requests.
- How to implement it: Use Python's
concurrent.futures
for clean and efficient multi-threading. - Best practices: Optimize thread count, handle errors, and respect website rules.
- Multi-threading vs. multiprocessing: Multi-threading is better for web requests, while multiprocessing suits CPU-heavy tasks.
Quick Comparison: Multi-Threading vs. Multiprocessing
Feature | Multi-Threading | Multiprocessing |
---|---|---|
Best For | Web requests (I/O) | Data processing (CPU) |
Memory Usage | Shared memory | Separate memory |
Performance with GIL | Great for I/O tasks | Better for CPU tasks |
Resource Overhead | Low | High |
Ready to make your scraping faster and more efficient? Learn how to set up multi-threading, handle errors, and stay compliant with website rules.
Basics of Multi-Threading in Web Scraping
What Multi-Threading Means
In Python, multi-threading lets a program handle multiple tasks at the same time within a single process. During I/O operations like making web requests, Python's Global Interpreter Lock (GIL) temporarily releases control, allowing other threads to execute while waiting for responses. This makes multi-threading especially useful for web scraping, where delays often happen due to network communication.
Benefits of Multi-Threading in Scraping
Multi-threading can drastically improve the performance of web scraping tasks. Here’s how:
- Cuts down wait time: Handles multiple web requests at once, reducing execution time significantly.
- Optimizes resource usage: Makes better use of system resources during network delays.
- Processes faster: Handles multiple responses simultaneously, speeding up data collection.
What to Know Before You Start
Python's concurrent.futures
module makes managing threads straightforward. Below is a simple example to get started:
from concurrent.futures import ThreadPoolExecutor
import requests
def scrape_page(url):
response = requests.get(url)
return response.text
urls = ["https://example1.com", "https://example2.com"]
with ThreadPoolExecutor(max_workers=4) as executor:
results = executor.map(scrape_page, urls)
To use multi-threading effectively, you’ll need:
- A basic understanding of Python and web scraping libraries like
requests
andBeautifulSoup
. - Familiarity with the
threading
orconcurrent.futures
modules. - Skills in debugging and handling errors to manage exceptions properly.
While multi-threading offers clear performance boosts, being prepared with the right tools and knowledge is crucial to make the most of it. With these basics in mind, you're ready to dive into implementing multi-threading for web scraping in Python.
Parallel and Concurrency in Python for Fast Web Scraping
How to Use Multi-Threading in Python for Web Scraping
Now that you know the basics of multi-threading, let’s look at how to use it effectively in Python for web scraping.
Setting Up Multi-Threading
To get started with multi-threading, you'll need a well-defined scraping function and Python's concurrent.futures
module. Here's a simple example to set up a multi-threaded scraper:
import concurrent.futures
import requests
from bs4 import BeautifulSoup
import logging
# Configure logging to monitor thread activity and troubleshoot issues
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
def scrape_page(url):
try:
headers = {'User-Agent': 'Mozilla/5.0 (compatible; MyScraperBot/1.0)'}
response = requests.get(url, headers=headers, timeout=10)
response.raise_for_status()
return BeautifulSoup(response.text, 'html.parser')
except requests.RequestException as e:
logger.error(f"Error scraping {url}: {str(e)}")
return None
Example Code for Multi-Threading
Here’s a practical example of scraping multiple pages concurrently:
def process_urls(urls, max_workers=4):
results = []
with concurrent.futures.ThreadPoolExecutor(max_workers=max_workers) as executor:
future_to_url = {executor.submit(scrape_page, url): url for url in urls}
for future in concurrent.futures.as_completed(future_to_url):
url = future_to_url[future]
try:
data = future.result()
if data:
results.append(data)
logger.info(f"Successfully scraped {url}")
except Exception as e:
logger.error(f"Error processing {url}: {str(e)}")
return results
# Usage example
urls = [
"https://example.com/page1",
"https://example.com/page2",
"https://example.com/page3"
]
scraped_data = process_urls(urls)
Error Handling Strategies
Error handling is crucial when working with web scraping. Here’s an approach to retry requests when they fail:
def scrape_with_retry(url, max_retries=3):
for attempt in range(max_retries):
try:
response = requests.get(url, timeout=(5, 15)) # Timeout: 5s (connect), 15s (read)
return response
except requests.Timeout:
logger.warning(f"Timeout on attempt {attempt + 1} for {url}")
if attempt == max_retries - 1:
raise
time.sleep(2 ** attempt) # Incremental backoff for retries
Common Error Types and How to Handle Them
Error Type | Likely Cause | Suggested Fix |
---|---|---|
ConnectionError | Network issues or blocked IP | Use retry logic with increasing delays |
HTTPError | Invalid status codes (403, 429) | Add headers and follow rate limits |
Timeout | Slow server response | Adjust timeout settings and retry requests |
sbb-itb-65bdb53
Tips for Effective Multi-Threaded Web Scraping
Optimizing Threads and Resources
Getting the thread count right can make or break your scraping efficiency. A good starting point is 5-10 threads, but you’ll need to tweak this based on how your system handles the load. Use tools like psutil
to keep an eye on CPU and memory usage, ensuring you don’t push your system too hard. While it's tempting to maximize performance, don’t forget to respect website rules to keep your scraping efforts sustainable.
Following Website Rules and Staying Legal
Abiding by website policies is non-negotiable if you want to scrape ethically. The table below outlines key practices to follow:
Requirement | Implementation | Purpose |
---|---|---|
Rate Limiting | Add delays between requests | Prevents server overload |
User Agent | Include custom headers | Ensures clear identification |
robots.txt | Follow directives | Honors site rules |
Error Handling | Retry failed requests | Handles failures gracefully |
If you're dealing with large-scale scraping, services like Web Scraping HQ can be a lifesaver. They handle resource allocation and compliance for you, making it easier to manage complex, multi-threaded operations without legal or technical headaches [1].
Multi-Threading vs. Multiprocessing in Web Scraping
Differences and When to Use Each
When it comes to web scraping, multi-threading often shines because most tasks involve waiting for server responses and managing network requests. On the other hand, multiprocessing can bring more overhead since each process operates in its own memory space and requires more resources [1].
Here’s how to decide which method to use:
Why Choose Multi-Threading?
- Handles many HTTP requests at the same time without using too many resources.
- Switches between threads quickly and efficiently.
- Shares memory space, which simplifies resource management.
When to Opt for Multiprocessing
- Ideal for CPU-heavy tasks like analyzing scraped data after collection.
- Works around the GIL (Global Interpreter Lock) for computational operations.
- Requires more memory and CPU power.
Quick Comparison
Here’s a side-by-side look at multi-threading and multiprocessing to help you decide:
Feature | Multi-Threading | Multiprocessing |
---|---|---|
Memory Usage | Shared memory, efficient | Separate memory for each process |
Best Use Case | Web requests (I/O-bound tasks) | Data processing (CPU-bound tasks) |
Complexity | Easier to implement and manage | More complex, needs careful planning |
Resource Overhead | Low | High |
Performance with GIL | Great for I/O tasks | Better for CPU-intensive tasks |
Scalability | Handles many requests well | Limited by CPU core availability |
In most web scraping situations, multi-threading is the go-to choice. However, multiprocessing can be a game-changer for tasks like processing massive datasets after scraping. For complex projects, you can even combine the two - multi-threading for scraping and multiprocessing for in-depth data analysis [2][3].
Now that you know when to use each, let’s dive into optimizing your web scraping scripts further.
Summary and Next Steps
Key Takeaways
Multi-threading can greatly improve Python web scraping by efficiently managing multiple HTTP requests. However, its success hinges on careful thread management and keeping an eye on system resources to avoid performance issues. The concurrent.futures
module is a helpful tool for implementing multi-threading while keeping your code clean and easy to follow [1].
Exploring Advanced Techniques
Once you're comfortable with the basics, you can level up your skills with these advanced methods:
- Asynchronous Programming: Use
aiohttp
for more efficient handling of network requests. - Hybrid Approaches: Combine multi-threading and multiprocessing for more complex scraping tasks.
- Performance Tuning: Take advantage of Python’s built-in profiling tools to optimize your scripts.
Why Consider Web Scraping HQ for Your Needs?
If your custom multi-threaded scripts aren't cutting it, Web Scraping HQ offers a reliable alternative. Their platform is designed to handle large-scale projects while staying compliant with legal requirements. Here's what they bring to the table:
Feature | What It Does |
---|---|
Automated QA | Ensures the data is accurate and complete. |
Legal Compliance | Keeps your scraping aligned with website terms of service. |
Scalable Systems | Manages even the most demanding projects. |
Custom Data Output | Provides data in the format you need. |
Whether you choose to build your own scraper or rely on a managed service, the goal is to strike a balance between efficiency and ethical practices. This ensures you can collect data effectively while respecting website policies.
FAQs
What is multithreading in web scraping?
Multithreading allows multiple tasks to run simultaneously, speeding up web scraping by processing several pages at the same time. This reduces waiting periods during network requests and boosts efficiency.
Here’s an example of how to use multithreading in web scraping with retry logic and rate limiting:
import concurrent.futures
import requests
from time import sleep
def scrape_page_with_retry(url, max_retries=3):
for attempt in range(max_retries):
try:
response = requests.get(url, timeout=10)
if response.status_code == 429: # Rate limit reached
sleep(2 ** attempt) # Exponential backoff
continue
return response.text
except requests.RequestException:
if attempt == max_retries - 1:
raise
sleep(2 ** attempt)
return None
# Implementation with error handling and rate limiting
with concurrent.futures.ThreadPoolExecutor(max_workers=5) as executor:
futures = {executor.submit(scrape_page_with_retry, url): url
for url in urls}
This setup includes error handling and rate limiting to ensure the process runs smoothly. Start with 5-10 threads and make adjustments based on how the website responds and your system's capacity [1].
For larger projects, tools like Web Scraping HQ can manage threads and monitor compliance automatically, simplifying the process.
While multithreading can significantly improve scraping speed, effective implementation also requires a balance between performance and ethical practices.