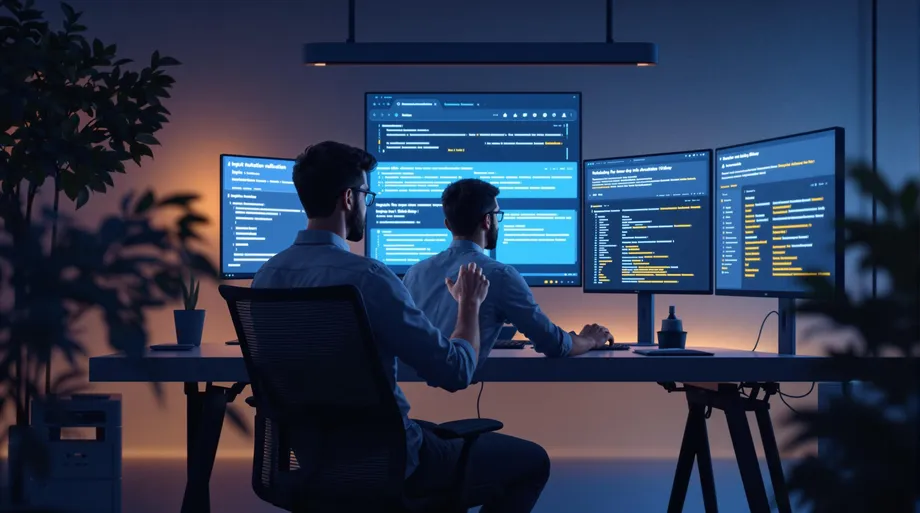
- Harsh Maur
- January 2, 2025
- 5 Mins read
- Scraping
MutationObserver API: Real-World Examples
The MutationObserver API lets you track changes in the DOM (like added elements, modified attributes, or text updates) in real-time with JavaScript. It’s widely used for tasks like detecting ad-blockers, scraping dynamic content, and validating form inputs instantly - all while keeping your website fast and responsive.
Key Features:
- Monitor multiple elements at once.
- Track specific changes (e.g., child nodes, attributes, or text content).
- Works asynchronously to avoid slowing down your app.
- Fully customizable settings to focus only on what matters.
Common Use Cases:
- Ad-blocker detection: Identify when ad elements are removed.
- Dynamic content handling: Scrape or process content updates on SPAs.
- Real-time input validation: Provide instant feedback on user input.
Example: Want to detect ad-blockers? Use the API to monitor ad containers and log changes. Need to validate form fields? Observe input changes and display feedback in real time.
This guide explains how to use MutationObserver effectively, with practical examples for modern web development.
Understanding MutationObserver
What Is MutationObserver?
The MutationObserver API is a JavaScript interface designed to track changes in the DOM (Document Object Model) in real-time. It can monitor updates like adding or removing elements, changing attributes, or modifying text content within the DOM [1][4].
How It Works and Key Features
MutationObserver runs efficiently and asynchronously, keeping the main JavaScript thread free for other tasks. This ensures your application stays responsive while observing multiple elements [1].
Here’s what you can configure with MutationObserver:
Configuration Option | What It Monitors | Example Use Case |
---|---|---|
childList | Changes to child nodes | Detecting when new elements are added to a container |
subtree | Changes in all descendant elements | Watching for updates in deeply nested components |
attributes | Modifications to attributes | Observing changes to styles or classes |
characterData | Updates to text content | Validating real-time user input |
To use MutationObserver, follow this basic setup:
let observer = new MutationObserver(callback);
observer.observe(node, config);
Pro Tip: Focus on specific elements instead of the entire document to avoid unnecessary overhead [1][4].
The callback function captures details about every change, including which attributes were modified, nodes that were added or removed, and the order of changes [1][2].
Thanks to its flexibility, MutationObserver is a go-to tool for tasks like detecting ad-blockers, handling dynamic content, or validating user input. We'll dive deeper into these applications in upcoming sections.
Understanding MutationObserver: From Basics to Advanced Implementation
sbb-itb-65bdb53
Applications of MutationObserver
The MutationObserver API is a handy tool in modern web development, solving a variety of practical challenges. Below are some of its main uses across different scenarios.
Ad-Blocker Detection
Websites often use MutationObserver to identify ad-blocking software by keeping an eye on ad-related DOM elements. For instance, by monitoring ad containers or placeholders (like div
elements with specific IDs or classes) using settings such as {childList: true, subtree: true}
, sites can detect when these elements are altered or removed. In response, they might display notifications or enforce paywalls to address the issue.
But the API isn’t just about ad detection - it’s also incredibly useful for managing dynamic content.
Extracting Dynamic Content
In web scraping, MutationObserver simplifies the process of handling dynamic content. For example, Web Scraping HQ uses it in their services to efficiently gather data from websites that frequently update, such as product catalogs, news feeds, or real-time pricing [3][4]. By monitoring changes in the DOM, MutationObserver makes it easier to collect data reliably from these constantly changing sources.
Its ability to track real-time updates also proves valuable in improving user experience, especially in web forms.
Real-Time Input Validation
MutationObserver is often employed to improve real-time input validation in web forms. By observing changes in input fields, it provides instant feedback on user errors without requiring the form to be submitted [1][2]. This approach ensures a smoother and more user-friendly validation process.
Examples of MutationObserver in Use
Here are some specific ways MutationObserver is used in practical scenarios. It helps developers address challenges like detecting ad-blockers, managing dynamic content, and providing real-time input validation.
Example: Detecting Ad-Blockers
const adContainer = document.getElementById('ad-container');
const callback = function (mutationsList, observer) {
for (let mutation of mutationsList) {
if (mutation.type === 'childList' && mutation.removedNodes.length > 0) {
console.log('Ad-blocker detected: Ad element was removed.');
// Take appropriate actions
}
}
};
const observer = new MutationObserver(callback);
observer.observe(adContainer, { childList: true });
This script monitors an ad container and logs a message if ad elements are removed. It’s a handy way to detect ad-blocker activity without interrupting the main thread [5].
Example: Handling Dynamic Web Content
const targetNode = document.querySelector('.dynamic-content');
const observer = new MutationObserver((mutations) => {
mutations.forEach((mutation) => {
if (mutation.type === 'childList') {
// Handle new content
const newElements = Array.from(mutation.addedNodes)
.filter(node => node.nodeType === 1);
if (newElements.length > 0) {
// Process the new elements
processNewContent(newElements);
}
}
});
});
observer.observe(targetNode, {
childList: true,
subtree: true,
attributes: true
});
This example tracks changes in dynamic content areas, such as single-page applications (SPAs). It ensures new elements, like items in a product catalog or news feed, are detected and processed accurately [1][4].
Example: Monitoring Input Fields
const formInput = document.querySelector('#email-input');
const feedbackElement = document.querySelector('#feedback');
const validateInput = new MutationObserver((mutations) => {
mutations.forEach((mutation) => {
if (mutation.type === 'attributes' &&
mutation.attributeName === 'value') {
const emailValue = formInput.value;
const isValid = /^[^\s@]+@[^\s@]+\.[^\s@]+$/.test(emailValue);
feedbackElement.textContent = isValid ?
'Valid email format' : 'Please enter a valid email';
feedbackElement.style.color = isValid ? 'green' : 'red';
}
});
});
validateInput.observe(formInput, {
attributes: true,
attributeFilter: ['value']
});
This setup provides instant feedback on email input validation. Users get immediate guidance, eliminating the need to submit the form to check for errors [1][2].
These examples demonstrate how MutationObserver can streamline tasks in web development, making it a powerful tool for modern applications.
Conclusion
MutationObserver provides practical tools for tackling various web development challenges. Whether it's detecting ad-blockers, monitoring dynamic content, or validating user input in real time, this API proves to be a handy resource for developers [2][5].
Its ability to monitor multiple elements efficiently makes it a strong choice for applications with complex requirements [1]. To make the most of MutationObserver, developers should focus on:
- Configuring observers to track only necessary changes for better performance.
- Using
disconnect
to stop observation when it's no longer needed. - Adding error handling to ensure stability in production environments.
The examples we've covered highlight how MutationObserver improves both user experience and app functionality. From detecting ad-blockers to offering instant feedback on form inputs, it addresses common development needs effectively [1][2].
As web applications continue to evolve, MutationObserver remains a reliable tool for managing DOM changes with precision [5].