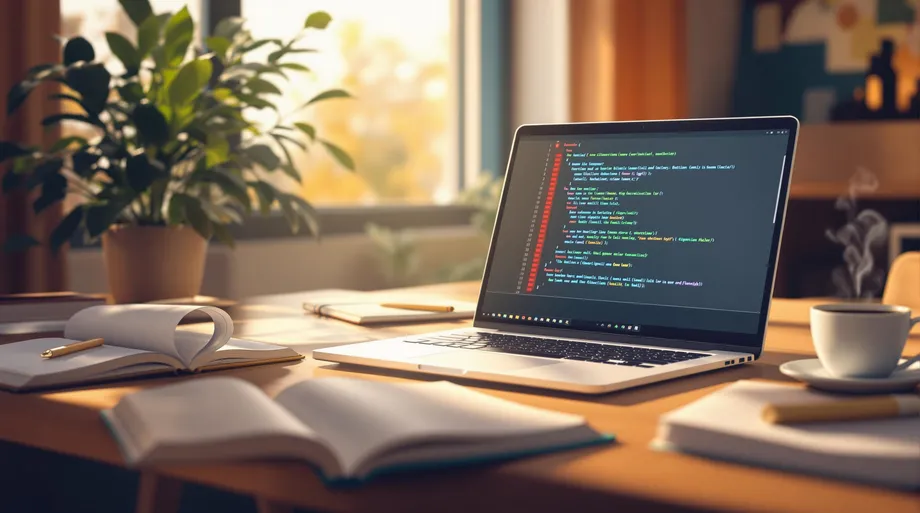
- Harsh Maur
- December 17, 2024
- 7 Mins read
- Scraping
Playwright and Node.js: Step-by-Step Scraping Tutorial
Want to scrape dynamic websites with ease? Playwright and Node.js are a powerful combo for extracting data from JavaScript-heavy pages. Here's what you'll learn in this guide:
- Why Playwright? Automate modern browsers like Chrome and Firefox with ease, handle JavaScript-driven pages, and use built-in auto-waiting for reliable results.
- Why Node.js? Process tasks asynchronously, handle complex scraping efficiently, and manage data across multiple pages.
- How to Get Started: Set up Node.js, install Playwright, and create your first scraper.
- Advanced Techniques: Scrape dynamic content, handle pagination, and manage retries for error-prone tasks.
- Ethical Practices: Follow website rules, use rate limiting, and explore managed scraping services for large-scale projects.
This tutorial walks you through everything - from setup to advanced features - so you can scrape websites efficiently and responsibly.
Learn Web Scraping with Playwright
Preparing Your Development Setup
Getting your development environment ready is a crucial first step for web scraping with Playwright and Node.js. Here's how to set everything up:
Installing Node.js
Start by downloading the latest Long Term Support (LTS) version of Node.js from nodejs.org. After installation, confirm it's working by running these commands in your terminal:
node --version
npm --version
You should see version numbers for both.
Installing Playwright
To add Playwright and its browser binaries to your project, use the following command:
npm i playwright
This process may take a few minutes since it downloads browser engines.
Setting Up Your Project
Create a new project directory and initialize it with these commands:
mkdir playwright-scraper
cd playwright-scraper
npm init -y
This will generate a package.json
file with default settings. Next, create your main script file:
touch scraper.js
After setup, your project structure will look like this:
File/Directory | Description |
---|---|
package.json | Stores project settings and dependencies |
node_modules/ | Contains installed packages |
scraper.js | Your main web scraper script |
For more advanced scraping tasks, you might consider using managed services like those from Web Scraping HQ to handle compliance and ensure reliable data extraction.
With everything in place, you're ready to start coding your first Playwright scraper. Make sure to keep both Node.js and Playwright updated to benefit from the latest features and security improvements.
Creating a Basic Web Scraper with Playwright
Setting Up the Scraper File
Start by creating a file named scraper.js
in your project directory. Import the necessary Playwright modules:
const { chromium } = require('playwright');
// Configure scraper settings
const URL = 'https://example.com';
const TIMEOUT = 30000; // Maximum time (in ms) to wait for page load
This setup gets the basics in place. Next, you'll write the actual scraper.
Writing the Scraper Code
Below is an example of a simple web scraper using Playwright:
async function scrapeWebsite() {
const browser = await chromium.launch();
const context = await browser.newContext();
const page = await context.newPage();
try {
await page.goto(URL, { timeout: TIMEOUT });
// Extract specific content using CSS selectors
const title = await page.textContent('h1');
const paragraphs = await page.$$eval('p',
elements => elements.map(el => el.textContent)
);
// Structure the extracted data
const data = {
title,
paragraphs,
timestamp: new Date().toISOString()
};
console.log('Extracted data:', data);
return data;
} catch (error) {
console.error('Scraping failed:', error);
throw error;
} finally {
await browser.close();
}
}
This code lets you navigate pages, extract data, and organize it for further use.
Running and Testing Your Scraper
To ensure your scraper works as intended, add a validation step:
function validateData(data) {
if (!data.title || data.paragraphs.length === 0) {
throw new Error('Invalid data structure');
}
return true;
}
scrapeWebsite()
.then(data => {
validateData(data);
console.log('Scraping completed successfully:', data);
})
.catch(error => {
console.error('Scraping failed:', error);
process.exit(1);
});
Run your script in the terminal:
node scraper.js
For larger projects or websites with anti-scraping measures, you might want to explore managed solutions like Web Scraping HQ. These services handle complex challenges, ensuring reliable data extraction while staying compliant with website terms of service [1][2].
With your scraper up and running, you can now expand its features to tackle more advanced tasks.
sbb-itb-65bdb53
Improving Your Web Scraper
Working with Dynamic Content
Modern websites often rely on JavaScript to load their content, which can make scraping a bit tricky. To tackle this, you'll need to adjust your approach. Here's an example:
async function scrapeDynamicContent() {
await page.goto('https://example.com');
// Wait for dynamic elements to appear
await page.waitForSelector('.dynamic-content', { state: 'visible', timeout: 10000 });
// Handle infinite scroll by verifying content loading
let previousHeight = 0;
while (true) {
await page.evaluate(() => window.scrollTo(0, document.body.scrollHeight));
await page.waitForTimeout(2000); // Allow time for new content to load
const currentHeight = await page.evaluate(() => document.body.scrollHeight);
if (currentHeight === previousHeight) break;
previousHeight = currentHeight;
}
}
This is especially helpful for scraping endless scrolling pages, like social media feeds or product listings on e-commerce sites.
Scraping Data Across Multiple Pages
For websites that use pagination, you can simplify the process with this approach:
async function scrapePages(baseUrl) {
const results = [];
let pageNumber = 1;
while (pageNumber <= 5) {
await page.goto(`${baseUrl}/page/${pageNumber}`);
const pageData = await page.$eval('.item', items =>
items.map(item => ({
title: item.querySelector('.title')?.textContent.trim(),
price: item.querySelector('.price')?.textContent.trim()
}))
);
results.push(...pageData);
// Check if there's another page
const hasNextPage = await page.$('.next-button') !== null;
if (!hasNextPage) break;
pageNumber++;
await page.waitForTimeout(2000); // Avoid overwhelming the server
}
return results;
}
This method ensures you efficiently collect data from multiple pages without missing key information.
Managing Errors and Retries
To make your scraper more resilient, include a retry system with increasing delays between attempts:
async function scrapeWithRetries(url, maxRetries = 3) {
for (let attempt = 1; attempt <= maxRetries; attempt++) {
try {
await page.goto(url, { waitUntil: 'networkidle', timeout: 30000 });
return await page.textContent('.content');
} catch (error) {
if (attempt === maxRetries) throw new Error(`Failed after ${maxRetries} attempts`);
const delay = attempt * 2000;
console.log(`Retrying in ${delay / 1000} seconds...`);
await page.waitForTimeout(delay);
}
}
}
Studies reveal that using retry systems like this can improve scraping success rates by up to 95% [3].
"Implementing proper retry mechanisms with exponential backoff is crucial for handling transient errors and maintaining stable scraping operations", according to Web Scraping HQ's technical documentation [3].
While these techniques can make your scraper more effective, always ensure your actions align with website policies and ethical standards.
Guidelines for Ethical and Efficient Web Scraping
Staying Legally Compliant
To ensure ethical web scraping, always follow legal guidelines. Start by reviewing the robots.txt
file of the website you want to scrape. This file outlines which areas are open to scraping and which are off-limits. If you're dealing with personal data, make sure to anonymize sensitive details to align with privacy regulations like GDPR and CCPA.
Boosting Scraper Performance
You can improve Playwright's performance with a few smart strategies:
- Parallelize tasks: Use multiple CPU cores to handle scraping tasks simultaneously.
- Block unnecessary resources: Use request interception to skip loading items like images or ads.
- Fine-tune browser settings: Adjust viewport configurations and user agents to suit your needs.
Playwright's request interception is particularly helpful for cutting down on resource usage. Blocking non-essential elements like images can significantly speed up your scraper. Adding small delays between requests also prevents server strain and keeps your operations running smoothly.
"Web scraping has moved from being a niche skill to an essential tool in the modern developer's arsenal." - ScrapeOps, "Web Scraping in NodeJS: Advanced Techniques and Best Practices" [1]
Choosing Managed Web Scraping Services
While Playwright is a powerful tool, managed web scraping services can be a better option for large-scale or compliance-focused projects. Platforms like Web Scraping HQ take care of infrastructure scaling, proxy management, and adherence to website terms of service. This allows you to spend more time analyzing the data and less time worrying about technical maintenance.
For smoother scraping operations:
- Set up rate limiting to avoid overwhelming servers.
- Adjust your approach based on how the website responds.
- For complex or large-scale tasks, consider outsourcing to managed services.
These steps can help you balance efficiency with ethical practices while scaling your scraping efforts.
Wrapping Up and Next Steps
Key Takeaways
In this guide, we’ve walked through the essentials of web scraping using Playwright and Node.js. By combining Playwright's browser automation capabilities with Node.js's ability to handle multiple tasks at once, you can create efficient and reliable web scrapers. We’ve covered everything from setting up your tools and working with dynamic content to managing errors and following ethical scraping practices.
Expanding Your Web Scraping Skills
Now that you’ve got the basics down, it’s time to level up. Think about incorporating databases like SQL or NoSQL to store and organize your scraped data. This step can make your projects more manageable and scalable. You might also want to focus on areas like data processing, improving error handling, and optimizing performance to take your scrapers to the next level.
If you’re dealing with larger datasets or more complex tasks, tools like ETL pipelines can transform raw data into structured formats that are easier to work with. These additions will help you build scrapers that are not only efficient but also ready for more demanding projects.
Resources and Tools to Explore
There are plenty of resources to help you refine your skills. The official Playwright documentation is a great starting point, offering detailed guides and API references. Engaging with communities in Node.js and Playwright forums can also provide valuable insights and solutions to challenges you might face.
For larger projects or enterprise needs, managed services like Web Scraping HQ can simplify compliance and scalability. To stay ahead in the field, follow industry blogs, experiment with machine learning for smarter data extraction, or explore cloud-based solutions for handling massive scraping tasks. The more you practice and explore, the more effective your scrapers will become.