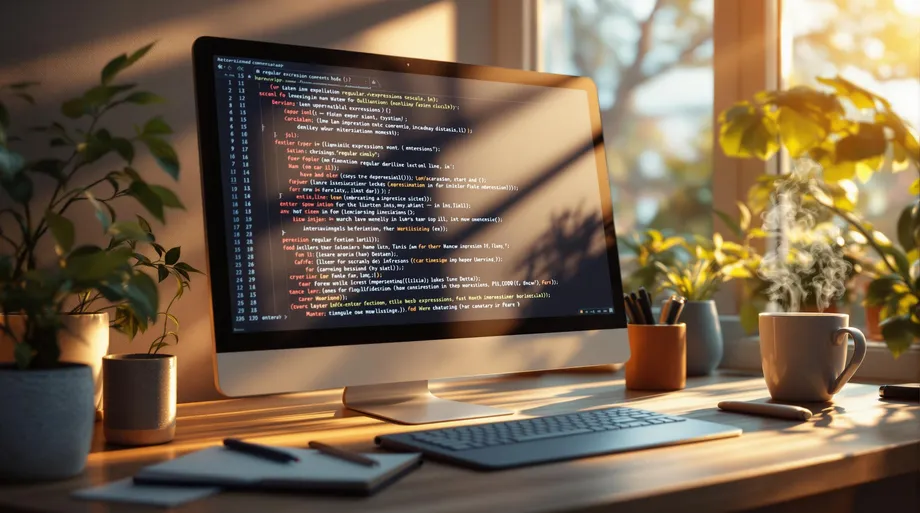
- Harsh Maur
- December 29, 2024
- 8 Mins read
- Scraping
Regular Expressions for Web Scraping: Basics and Examples
Regular expressions (regex) are essential for web scraping. They help you extract specific data, like emails, URLs, and phone numbers, from raw HTML efficiently. By combining regex with Python libraries like BeautifulSoup
and requests
, you can automate data extraction and clean messy web content.
Key Takeaways:
-
What You Need: Basic Python, HTML knowledge, and libraries like
requests
,BeautifulSoup
, andre
. -
Common Regex Patterns:
-
Emails:
r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b'
-
URLs:
r'http[s]?://(?:[a-zA-Z]|[0-9]|[$-_@.&+]|[!*\\(\\),]|(?:%[0-9a-fA-F][0-9a-fA-F]))+'
-
Phone Numbers:
r'\b\d{3}[-.]?\d{3}[-.]?\d{4}\b'
-
Emails:
-
Setup: Install
requests
andbeautifulsoup4
usingpip
, and use Python's built-inre
module for regex. -
Ethical Scraping: Add delays, respect
robots.txt
, and comply with data protection laws.
Regex is powerful but works best when paired with tools like BeautifulSoup for parsing HTML. By mastering this, you can extract data accurately while adhering to ethical practices.
Scraping Data using Regular Expression
Setting Up for Web Scraping
To get started with web scraping, you'll need two key libraries:
pip install requests beautifulsoup4
Python's re
module, used for regular expressions, is included by default, so no additional installation is required.
Using Libraries in Python
Once installed, you can import the libraries into your Python script like this:
import requests
from bs4 import BeautifulSoup
import re
url = 'https://example.com'
response = requests.get(url)
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
These tools work together to help you fetch, parse, and analyze web content:
Library | Purpose | Common Operations |
---|---|---|
requests |
Fetch web pages | Sending HTTP requests |
BeautifulSoup |
Parse HTML content | Selecting elements |
re |
Handle text patterns | Regex-based matching |
Here's an example of extracting email addresses from a webpage using regex:
paragraphs = soup.find_all('p')
email_pattern = r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b'
emails = re.findall(email_pattern, ' '.join([p.text for p in paragraphs]))
Before processing a response, always ensure the HTTP request is successful:
try:
response = requests.get(url)
response.raise_for_status()
except requests.RequestException as e:
print(f"Error: {e}")
With this setup, you're equipped to start working with regular expressions for data extraction. This foundation will prepare you to explore regex techniques and apply them effectively in web scraping projects.
Regular Expressions Basics for Web Scraping
Regex Syntax Basics
Regular expressions (regex) are a tool for matching and extracting specific data from text using special characters and rules. For instance, symbols like ^
and $
mark positions, while quantifiers such as *
and +
handle repetition. Character sets like [a-z]
or \d
help match specific types of characters. By combining these elements, you can craft patterns like this one for identifying prices:
price_pattern = r'\$\d+\.\d{2}' # Matches prices like $19.99
These components allow you to build precise patterns for extracting the exact data you need from web pages.
Common Web Scraping Patterns
Here are some regex patterns commonly used in web scraping:
# URL pattern
url_pattern = r'http[s]?://(?:[a-zA-Z]|[0-9]|[$-_@.&+]|[!*\\(\\),]|(?:%[0-9a-fA-F][0-9a-fA-F]))+'
# Date pattern (YYYY-MM-DD)
date_pattern = r'\b\d{4}-\d{2}-\d{2}\b'
# Phone number pattern
phone_pattern = r'\d{3}[-.]?\d{3}[-.]?\d{4}'
You can use these patterns with Python's re
library alongside BeautifulSoup for parsing HTML. For example, to extract dates from a webpage:
import re
from bs4 import BeautifulSoup
# Extract all dates from HTML content
dates = re.findall(date_pattern, html_content)
Testing Regex Patterns
Testing your regex patterns is crucial, especially when dealing with messy or unpredictable web data. Tools like Regex101.com are excellent for validating and refining patterns before using them. Start by breaking down complex patterns into smaller, testable parts, and then combine them once you're confident they work.
Capture groups, marked by parentheses ()
, are particularly useful for isolating specific parts of the matched text. For instance, when working with dynamic content, you can use BeautifulSoup to first narrow down the relevant HTML sections and then apply regex to extract the desired information.
After testing, these patterns can be smoothly integrated into your web scraping scripts for reliable and efficient data extraction.
sbb-itb-65bdb53
Regex in Web Scraping Examples
Data Extraction with Regex
Here's how you can use regex alongside BeautifulSoup to extract specific data from web pages:
import re
from bs4 import BeautifulSoup
import requests
# Extract both links and images
def extract_elements(html_content):
soup = BeautifulSoup(html_content, 'html.parser')
# Find links
links = [(a['href'], a.text) for a in soup.find_all('a', href=True)]
# Find images
images = [(img['src'], img.get('alt', '')) for img in soup.find_all('img')]
return links, images
For extracting product details like titles and prices, you can use regex for precise targeting:
# Extract product titles and prices
def extract_product_info(html_content):
soup = BeautifulSoup(html_content, 'html.parser')
# Find product titles
titles = soup.find_all('h2', class_='product-title')
title_text = [title.text.strip() for title in titles]
# Use regex to find prices
price_pattern = r'\$\d+\.?\d*'
prices = re.findall(price_pattern, html_content)
return title_text, prices
Cleaning Extracted Data
Scraped data often needs cleanup to make it ready for analysis or storage. Below are some examples of how to handle common cleanup tasks:
# Clean and convert prices to numerical format
def clean_price(price_str):
pattern = r'\$([0-9,]+\.?\d*)'
match = re.search(pattern, price_str)
return float(match.group(1).replace(',', '')) if match else None
# Standardize phone numbers from contact pages
def clean_phone(phone_str):
digits = re.sub(r'\D', '', phone_str)
pattern = r'(\d{3})(\d{3})(\d{4})'
return re.sub(pattern, r'(\1) \2-\3', digits)
# Clean and structure HTML table data
def clean_table_data(html_content):
soup = BeautifulSoup(html_content, 'html.parser')
rows = soup.find_all('tr')
return [[cell.text.strip() for cell in row.find_all(['td', 'th'])]
for row in rows]
These cleanup techniques help prepare data for various uses, such as tracking prices, building contact lists, or analyzing structured tables. When paired with web scraping tools, they make handling large datasets much easier and more efficient.
Mastering these basics sets the stage for diving into more advanced regex patterns and ensuring ethical practices in web scraping.
Advanced Regex and Ethical Web Scraping
Advanced Regex for Complex Data
Basic regex patterns might work for straightforward tasks, but extracting data from complex HTML structures often requires more advanced techniques. For instance, the re.DOTALL
flag is essential when dealing with multi-line matches, especially for nested HTML elements. Here's an example of how to extract product details from nested structures:
import re
# Extract product information from nested structures
def extract_nested_products(html_content):
pattern = r'<div class="product">.*?<h2>(.*?)</h2>.*?<span class="price">(?P<price>.*?)</span>.*?</div>'
matches = re.finditer(pattern, html_content, re.DOTALL)
products = []
for match in matches:
products.append({
'name': match.group(1).strip(),
'price': match.group('price').strip()
})
return products
When regex alone isn't enough - like with dynamic or deeply nested content - combining it with tools like BeautifulSoup can improve accuracy and efficiency.
Here’s a quick reference guide for handling different data structures with regex:
Data Structure Type | Recommended Approach | Best Practice |
---|---|---|
Nested Elements | Recursive patterns | Use alongside BeautifulSoup |
Multiple Groups | Labels for matched data | Test with varied inputs |
Dynamic Content | Recursive patterns | Cache results |
While refining your regex and scraping methods, always keep ethical considerations in mind.
Ethical Scraping Practices
Knowing advanced regex techniques is only part of the equation. Ethical web scraping is essential for responsible data collection and maintaining a good relationship with website owners. As Software Engineer Bonaventure Ogeto explains:
"Ethical web scraping is not just about avoiding legal issues, but also about respecting the intellectual property of website owners".
A recent study found that 70% of companies experience data quality issues due to web scraping, emphasizing the need for responsible practices.
Here are some key steps to ensure ethical scraping:
- Add delays between requests to avoid overloading servers.
-
Respect the website's
robots.txt
file and terms of service. - Comply with data protection laws, such as GDPR or CCPA.
Below is an example of how to implement rate limiting in your scraping scripts:
# Ethical scraping with rate limiting
import time
from random import uniform
for url in urls:
time.sleep(uniform(2, 5)) # Random delay
headers = {'User-Agent': 'Your Bot (email@example.com)'}
# Perform request and process data
Additionally, keep track of your activities through monitoring and logging to ensure compliance with legal and ethical standards. This level of transparency will help you avoid potential issues down the line.
Conclusion and Further Steps
Key Points Summary
Regular expressions play a crucial role in web scraping, making data extraction more precise and keeping your code organized.
Pattern Type | Common Uses |
---|---|
Basic | Extracting emails, URLs, phone numbers |
HTML Tags | Pulling content, targeting elements |
Complex Data | Handling nested structures, dynamic content |
The true power of regex lies in combining it with specialized libraries while understanding its limitations. As mentioned earlier, pairing regex skills with ethical practices ensures responsible and effective web scraping.
Additional Learning Resources
If you want to refine your skills and stay informed, check out these resources:
- Online Tools: Use platforms like Regex101.com for testing patterns or exploring interactive regex visualizations.
- Professional Services: Companies like Webscraping HQ offer managed data extraction solutions tailored for businesses.
- Community Resources: Dive into developer forums, open-source documentation, and guides on industry best practices.
Always prioritize ethical scraping practices when applying these tools and techniques. With a solid understanding of regex and web scraping principles, you'll be ready to handle both straightforward and more intricate data extraction tasks.
The field of web scraping is constantly changing - keep an eye on emerging tools and methods to stay ahead.
FAQs
Let's dive into some common questions about how regular expressions (regex) fit into web scraping.
What is the purpose of regular expressions in text scraping?
Regex is a powerful way to locate and extract specific types of data from text. It works by defining patterns that match the information you're looking for. For instance, you can use regex to pull dates from text like this:
date_pattern = r'\b\d{4}-\d{2}-\d{2}\b' # Matches dates in YYYY-MM-DD format
Is regex used in web scraping?
Absolutely. Regex plays a key role in web scraping. It's especially handy for:
- Extracting static content
- Cleaning up messy data
- Matching recurring patterns
- Handling dynamic data in certain scenarios
When using regex for web scraping, keep these tips in mind:
- Test your patterns thoroughly to ensure accuracy.
- Follow ethical scraping guidelines.
- Respect the website's terms of service.
- Use rate limiting to avoid overloading servers.
Mastering regex will make your data extraction process smoother and more efficient.