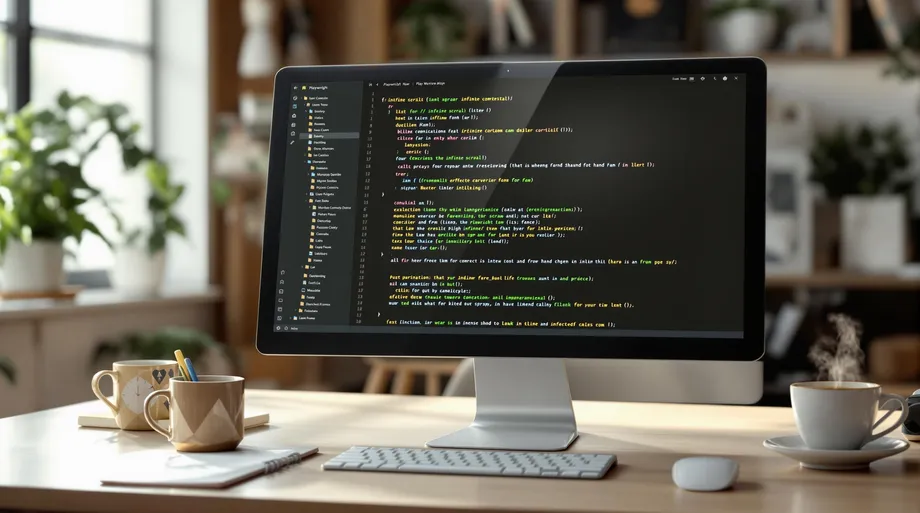
- Harsh Maur
- November 28, 2024
- 8 Mins read
- Scraping
Scraping Infinite Scroll with Playwright
Scraping infinite scroll pages can be tricky, but Playwright makes it manageable. Infinite scroll dynamically loads content as you scroll, which traditional scrapers struggle to handle. Playwright, a browser automation tool by Microsoft, helps you extract data by simulating user actions, handling JavaScript, and managing network requests.
Why Use Playwright for Infinite Scroll?
- Automates scrolling to load dynamic content.
- Works across Chromium, Firefox, and WebKit browsers.
- Executes JavaScript for seamless content extraction.
- Supports headless mode for faster performance.
- Handles proxies, user agents, and error retries to avoid detection.
Key Steps:
-
Install Playwright: Use
npm i -D playwright
. - Automate Scrolling: Use scripts to scroll and load all content.
- Extract Data: Target dynamic elements with selectors.
- Handle Anti-Scraping Tools: Rotate IPs, and user agents, and manage CAPTCHAs.
-
Stay Legal: Respect
robots.txt
and comply with data privacy laws.
Example Use Case:
Automate scrolling, extract product details and handle delays with retry mechanisms. For large-scale tasks, integrate Playwright into a cluster or pipeline for efficiency.
Quick Tip: Always test your script and adjust for specific site behaviors like loading delays or API requests.
Playwright simplifies the complexities of scraping infinite scroll, making it a go-to tool for dynamic content extraction.
Related video from YouTube
Setting Up Playwright for Scraping
To scrape infinite scroll pages with Playwright, you'll need to set up and configure your environment properly. Below are the steps to get you started.
How to Install Playwright
Installing Playwright is simple with Node Package Manager (npm). Run the following command in your terminal:
npm i -D playwright
Writing Your First Script
Here’s an example script to handle infinite scrolling:
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch({ headless: false });
const page = await browser.newPage();
// Navigate to the target page
await page.goto('https://example.com', {
waitUntil: 'networkidle'
});
// Simulate scrolling to load dynamic content
await page.evaluate(() => window.scrollTo(0, document.body.scrollHeight));
await browser.close();
})();
This script opens a browser, goes to a webpage, and scrolls down to trigger the loading of dynamic content. The waitUntil: 'networkidle'
option ensures the page is fully loaded by waiting for all network activity to stop.
Once this basic script is working, you can expand its functionality by setting up browser contexts for more control.
Setting Up Browser Contexts
Browser contexts allow you to create independent browsing sessions within a single browser instance. This is useful for running multiple scraping tasks simultaneously. Below are some configurations you can apply:
Configuration Type | Example Code |
---|---|
Run in Headless Mode: Speeds up execution by hiding the browser UI (some sites may block headless browsers) | chromium.launch({ headless: true }) |
Set a Custom User Agent: Helps avoid detection by mimicking a real browser | context.setUserAgent('Mozilla/5.0...') |
Use a Proxy: Rotates IPs to bypass rate limits or restrictions | launch({ proxy: { server: 'http://myproxy.com:8080' }}) |
Here’s how to set up a browser context with error handling and timeout management:
const browser = await chromium.launch({
headless: true,
timeout: 30000,
proxy: {
server: 'http://myproxy.com:8080',
username: 'user',
password: 'pass'
}
});
const context = await browser.newContext({
userAgent: 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36'
});
try {
const page = await context.newPage();
// Add your scraping logic here
} catch (error) {
console.error('Scraping error:', error);
} finally {
await browser.close();
}
For infinite scroll pages, you may need to implement retries and adjust timeouts to handle delays caused by slow-loading content. The default timeout of 30 seconds can be increased for websites that take longer to load.
Scraping Pages with Infinite Scroll
How Infinite Scroll Works
Infinite scroll loads content dynamically as users scroll down the page, replacing traditional pagination. This is achieved through JavaScript-triggered API requests. By checking the Network tab in developer tools, you can see how scrolling initiates XHR or fetches API requests to load new chunks of content into the DOM. Identifying these API patterns allows you to retrieve data directly, skipping the need for manual scrolling.
Now that we understand the mechanics, let's see how Playwright can automate this process.
Automating Scrolling with Playwright
Here’s a script that handles infinite scrolling, ensuring all dynamic content is loaded:
const { chromium } = require('playwright');
async function scrapeInfiniteScroll(url) {
const browser = await chromium.launch({ headless: true });
const page = await browser.newPage();
await page.goto(url, { waitUntil: 'networkidle' });
let previousHeight = 0;
let retries = 0;
const maxRetries = 3;
while (retries < maxRetries) {
// Get current scroll height
const currentHeight = await page.evaluate('document.body.scrollHeight');
// Stop if no new content loads after multiple attempts
if (currentHeight === previousHeight) {
retries++;
continue;
}
// Reset retries if new content found
retries = 0;
previousHeight = currentHeight;
// Scroll and wait for network activity
await page.evaluate('window.scrollTo(0, document.body.scrollHeight)');
await page.waitForTimeout(1000);
await page.waitForLoadState('networkidle');
}
return page;
}
This script uses a retry mechanism to ensure it continues scrolling until all content is loaded, even if there are delays.
Once the scrolling process completes, you can move on to extracting the data you need.
Extracting Data from Loaded Content
Playwright’s selector engine makes it easy to target dynamic elements, even if their structure changes. Here’s an example of extracting product details from an e-commerce site:
const items = await page.$eval('.product-card', elements =>
elements.map(el => ({
title: el.querySelector('.product-title')?.textContent?.trim(),
price: el.querySelector('.product-price')?.textContent?.trim(),
rating: el.querySelector('.rating')?.getAttribute('data-rating')
}))
);
To ensure accurate data extraction, follow these practices:
Aspect | Implementation | Purpose |
---|---|---|
Selector Strategy | Use stable attributes like IDs or data attributes | Ensures consistent targeting of elements |
Error Handling | Wrap selectors in try-catch blocks | Prevents crashes if elements are missing |
Rate Limiting | Add small delays between scroll actions | Reduces server load and avoids detection |
For better reliability, use Playwright's waiting features:
// Wait for specific elements to load
await page.waitForSelector('.product-card', { timeout: 5000 });
// Wait for network requests to complete
await page.waitForLoadState('networkidle');
This ensures all dynamic content is fully loaded before you start extracting data, making your scraping process more dependable.
sbb-itb-65bdb53
Tips for Better Scraping
Improving Script Performance
To keep your script running smoothly and avoid issues like crashes, it's essential to manage memory usage and set a scroll depth limit. This is particularly important when working with infinite scroll pages and large datasets.
Here's a refined example of scroll handling code:
async function controlledScroll(page, maxScrolls = 50) {
let scrollCount = 0;
let previousHeight = 0;
while (scrollCount < maxScrolls) {
const currentHeight = await page.evaluate('document.body.scrollHeight');
if (currentHeight === previousHeight) break;
previousHeight = currentHeight;
await page.evaluate('window.scrollBy(0, 1000)');
await page.waitForTimeout(1500); // Adds a delay between scrolls
scrollCount++;
}
}
Efficient performance is key, but you also need strategies to handle anti-scraping mechanisms for successful data extraction.
Dealing with Anti-Scraping Tools
Websites often employ anti-scraping measures to block bots. Here's how you can address some of the most common challenges:
const context = await browser.newContext({
userAgent: 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) Chrome/119.0.0.0',
proxy: {
server: 'proxy.example.com:8080',
username: 'user',
password: 'pass'
}
});
Challenge | Solution | Implementation |
---|---|---|
IP Blocking | Rotate IP addresses | Use proxy rotation services |
User Agent Detection | Rotate user agents | Use a pool of browser signatures |
CAPTCHAs | Solve CAPTCHAs | Services like 2captcha can help |
While technical solutions are vital, it's equally important to follow legal and ethical guidelines when scraping.
Staying Legally Compliant
Web scraping must align with terms of service and data privacy regulations. Follow these practices to ensure compliance:
1. Respect robots.txt
Always check and adhere to the website's robots.txt file before scraping:
async function checkRobotsTxt(domain) {
const response = await fetch(`${domain}/robots.txt`);
const robotsTxt = await response.text();
return robotsTxt.includes('Disallow: /api/');
}
2. Rate Limiting
Avoid overloading the server by setting appropriate rate limits:
const rateLimiter = {
requestDelay: 2000,
async wait() {
await new Promise(resolve => setTimeout(resolve, this.requestDelay));
}
};
3. Data Privacy
When dealing with personal data, ensure compliance with GDPR, CCPA, and similar regulations by:
- Encrypting stored data
- Setting clear data retention policies
- Offering mechanisms for data deletion
- Keeping records of data processing activities
For enterprise-level scraping needs, platforms like Webscraping HQ provide tools and infrastructure designed with compliance in mind.
Advanced Techniques and Scaling
Customizing Scripts for Specific Data
Extracting data from infinite scroll pages can be tricky, especially when targeting specific fields. To handle this effectively, you need precise scripts that manage dynamic content. Here's an example of how to extract product details using Playwright:
async function extractProductData(page) {
const products = await page.$eval('.product-card', elements =>
elements.map(el => ({
name: el.querySelector('.product-name')?.textContent?.trim(),
price: el.querySelector('.product-price')?.textContent?.trim(),
rating: el.querySelector('.rating')?.getAttribute('data-score'),
availability: el.querySelector('.stock')?.textContent?.trim()
}))
);
return products.filter(product => product.name && product.price);
}
Accuracy matters, especially when dealing with large datasets. Validating the extracted data ensures you only work with reliable information. Here's a validation function:
function validateData(products) {
return products.filter(product => {
return product.price && product.rating &&
isValidPrice(product.price) && isValidRating(product.rating);
});
}
Once you've extracted and validated the data, you can move on to integrating these scripts into a scalable workflow.
Using Playwright with Data Pipelines
To handle large-scale data scraping, integrating your scripts into a robust data pipeline is key. Managing errors and ensuring efficient processing helps maintain reliability. Below is an example of using Playwright with a cluster setup:
const { Cluster } = require('playwright-cluster');
const cluster = new Cluster({
concurrency: 5,
maxCrawlingTime: 180000,
puppeteerOptions: {
headless: true,
timeout: 30000
}
});
await cluster.task(async ({ page, data: url }) => {
await page.goto(url);
await controlledScroll(page);
const products = await extractProductData(page);
return validateData(products);
});
Leveraging Services Like Web Scraping HQ
For large-scale operations, managed services like Web Scraping HQ can be a game-changer. They handle infrastructure, compliance, and scalability, allowing you to focus on analyzing the data rather than managing the scraping process.
"Playwright is a powerful tool developed by Microsoft, it allows developers to write reliable end-to-end tests and perform browser automation tasks with ease." - DevLoker, DEV Community
These services are especially helpful when scaling beyond what custom scripts can manage, offering ready-made solutions for handling challenges like infinite scroll efficiently.
Conclusion
Key Takeaways
Playwright stands out for its ability to handle cross-browser compatibility and manage dynamic content, making it a strong choice for scraping infinite scroll pages. Tools like page.waitForFunction
and page.evaluate
empower developers to tackle even the most intricate infinite scroll setups.
Next Steps to Consider
If you're working on smaller projects, you can use Playwright's core features to build custom scripts. Take advantage of its debugging tools, such as --debug
mode, to troubleshoot infinite scroll challenges during development. Consistent testing and monitoring are essential to ensure reliable data extraction.
For larger-scale or enterprise projects, platforms like Web Scraping HQ provide managed web scraping services tailored to handle complex tasks. They excel at navigating issues like rate limits and legal compliance while maintaining high data quality.
To keep your scraping efforts effective, stay updated on Playwright's latest features and updates. Whether you're writing custom scripts or using managed solutions, ongoing maintenance, and optimization are key to achieving consistent results over time.