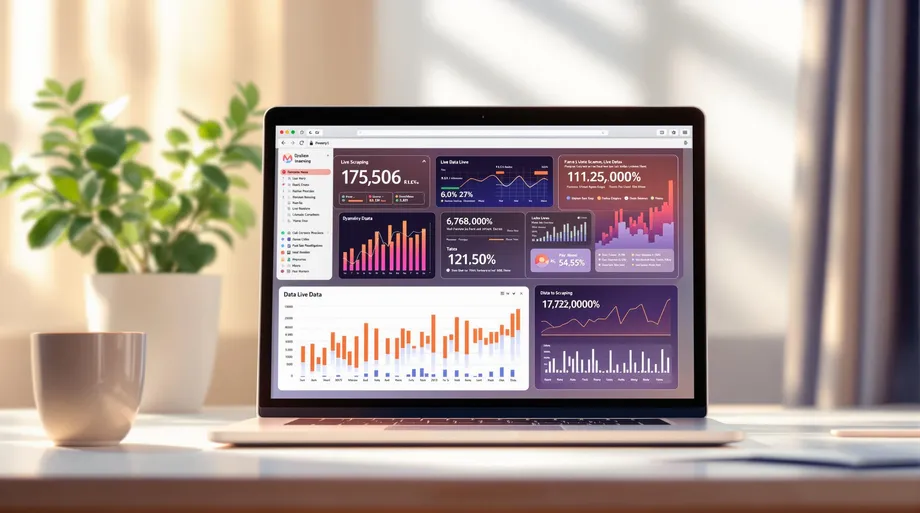
- Harsh Maur
- February 25, 2025
- 9 Mins read
- Scraping
Ultimate Guide to Real-Time Data Scraping with Playwright
Real-time data scraping is essential for businesses to make quick, informed decisions. Playwright is a powerful tool for scraping dynamic, JavaScript-heavy websites efficiently. Here's why Playwright stands out:
- Cross-Browser Support: Works with Chromium, Firefox, and WebKit.
- Auto-Wait Mechanism: Avoids errors by waiting for elements to load.
- Handles Dynamic Content: Extracts data from AJAX-heavy or SPA websites.
- Customizable: Offers advanced browser settings for better control.
Key Features and Benefits:
- E-commerce: Track inventory in real-time to avoid overselling.
- Finance: Monitor stock prices and execute trades without delays.
- Performance Optimization: Block unnecessary resources and use caching.
- Error Management: Retry failed tasks with exponential backoff.
Quick Setup:
-
Install Playwright:
npm init playwright@latest
-
Launch a browser:
chromium.launch({ headless: false })
- Write your first script to scrape data dynamically.
Comparison Table:
Feature | Playwright | Puppeteer | Selenium |
---|---|---|---|
Speed | Fast | Fastest | Slower |
Browser Support | Multiple | Chromium only | All major |
Language Support | Multiple | JavaScript only | Multiple |
Development Experience | Excellent | Excellent | Good |
Playwright is ideal for businesses that need accurate, real-time data scraping solutions. From handling infinite scrolls to monitoring API responses, it ensures reliable data collection for critical operations.
Playwright Setup Guide
Setting up Playwright involves specific steps to ensure everything runs smoothly. From installation to writing your first scraping script, this guide will walk you through the process.
Installation Steps
Before starting, make sure you have Node.js v14 or higher installed. Then, install Playwright with the following commands:
npm init playwright@latest
npx playwright install
For a better coding experience, install the Playwright Test extension in VS Code. It provides features like auto-completion, debugging tools, and handy code snippets.
Browser Configuration
Fine-tuning Playwright's browser settings can improve your scraping experience. Here's a quick overview of some important configuration options:
Configuration Option | Purpose | Example Setting |
---|---|---|
Headless Mode | Show or hide the browser during execution | headless: false |
JavaScript | Enable or disable JavaScript | javaScriptEnabled: false |
Timeout | Set time limits for actions | actionTimeout: 30000 |
Browser Selection | Pick a browser engine | browserName: 'chromium' |
Performance Mode | Reduce resource usage | args: ['--disable-gpu'] |
To further refine your setup, customize the browser launch settings like this:
const browser = await chromium.launch({
args: ['--no-sandbox', '--disable-setuid-sandbox'],
channel: 'chrome',
headless: false
});
Basic Script Structure
Here’s a simple script to get started with web scraping:
const { chromium } = require("playwright");
async function scrapeData() {
const browser = await chromium.launch();
const context = await browser.newContext();
const page = await context.newPage();
try {
await page.goto("https://example.com");
const data = await page.waitForSelector(".target-element");
const extractedContent = await data.textContent();
console.log(extractedContent);
} catch (error) {
console.error("Scraping failed:", error);
} finally {
await browser.close();
}
}
scrapeData();
For handling more dynamic content, adjust the browser context settings:
const context = await browser.newContext({
bypassCSP: true,
userAgent: 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36',
viewport: { width: 1920, height: 1080 }
});
These tweaks can improve reliability, boost performance, and reduce the chances of detection. Keep your Playwright and browser binaries up to date by running:
npm install -D @playwright/test@latest
npx playwright install
This ensures you have access to the latest features and security updates. Next, dive into techniques for managing dynamic content efficiently.
Dynamic Content Management
Modern websites often use JavaScript to load dynamic content, requiring careful techniques to extract data effectively.
JavaScript Page Handling
Instead of relying on fixed delays, use smarter, context-aware waiting methods:
// Wait for a specific element to appear
await page.waitForSelector('.dynamic-content', { state: 'visible' });
// Wait until network activity slows down
await page.waitForLoadState('networkidle');
// Run custom JavaScript after the page finishes loading
await page.evaluate(() => {
const dynamicData = document.querySelector('.data-container').textContent;
return dynamicData;
});
For Single-Page Applications (SPAs), combine multiple waiting strategies to ensure accurate data retrieval:
async function waitForContent(page) {
await Promise.all([
page.waitForLoadState('domcontentloaded'),
page.waitForSelector('.content-loaded'),
page.waitForResponse(response =>
response.url().includes('/api/data')
)
]);
}
Once you’ve handled JavaScript loading, the next step is to improve performance by managing network requests.
Network Request Control
Speed up scraping by filtering out unnecessary network requests:
await page.route('**/*', route => {
const resourceType = route.request().resourceType();
if (['image', 'stylesheet', 'font', 'media'].includes(resourceType)) {
route.abort();
} else {
route.continue();
}
});
Excluding non-essential resources can drastically reduce scraping time, especially for SPAs. If you need to monitor specific API calls, you can intercept them:
await page.route('**/api/data', route => {
const request = route.request();
console.log(`Intercepted API call: ${request.url()}`);
route.continue();
});
With network traffic optimized, you can move on to handling infinite scrolling and pagination.
Scroll and Pagination Handling
For infinite scroll pages, use a script to scroll until all content is loaded:
async function scrollToBottom(page) {
let previousHeight = 0;
let currentHeight = await page.evaluate(() => document.body.scrollHeight);
while (previousHeight !== currentHeight) {
previousHeight = currentHeight;
await page.evaluate(() => window.scrollTo(0, document.body.scrollHeight));
await page.waitForTimeout(1000);
currentHeight = await page.evaluate(() => document.body.scrollHeight);
}
}
For pagination where the URL doesn’t change, you can loop through pages until the end:
async function handlePagination(page) {
while (true) {
await page.waitForSelector('.content-loaded');
const data = await extractPageData(page);
const nextButton = await page.$('.next-page:not([disabled])');
if (!nextButton) break;
await nextButton.click();
await page.waitForSelector('.loading', { state: 'hidden' });
}
}
"Playwright, with its comprehensive browser support, auto-wait features, and ability to execute JavaScript, represents a leap forward in the field of web scraping." - yahia almarafi, Data Analyst
Real-Time Data Collection Methods
Playwright's dynamic content controls make it possible to monitor and act on real-time data instantly.
Data Monitoring Systems
Playwright can be paired with monitoring tools like Checkly to turn scripts into powerful monitoring solutions. Here's an example of setting up periodic checks:
// Schedule periodic checks
const monitor = async (page, interval) => {
while (true) {
await page.waitForLoadState('networkidle');
const data = await page.evaluate(() => {
return {
price: document.querySelector('.price')?.textContent,
timestamp: new Date().toISOString()
};
});
await processData(data);
await new Promise(resolve => setTimeout(resolve, interval));
}
};
You can also add retry patterns to handle errors more effectively:
async function resilientMonitor(page, selector, maxRetries = 3) {
for (let attempt = 1; attempt <= maxRetries; attempt++) {
try {
await page.waitForSelector(selector, { timeout: 5000 });
return await page.evaluate(sel => document.querySelector(sel).textContent, selector);
} catch (error) {
if (attempt === maxRetries) throw error;
await page.reload();
}
}
}
These methods can be extended with change detection for immediate updates.
Change Detection Methods
To track changes in the DOM, you can use MutationObserver
:
async function detectChanges(page) {
await page.evaluate(() => {
window._changes = [];
const observer = new MutationObserver(mutations => {
window._changes.push(...mutations);
});
observer.observe(document.body, {
childList: true,
subtree: true,
attributes: true
});
});
await page.waitForFunction(() =>
window._changes.some(m => m.target.matches('.target-element'))
);
}
For API-driven updates, intercept and monitor network responses:
page.on('response', async response => {
if (response.url().includes('/api/data')) {
const newData = await response.json();
if (hasChanged(newData, previousData)) {
notifyChange(newData);
}
}
});
Combining these techniques ensures you can react to updates efficiently.
Tool Integration
By integrating services like Web Scraping HQ, you can enhance your scraping workflows. Here's an example setup:
const { chromium } = require('playwright');
const WebScrapingHQ = require('webscrapinghq-sdk');
async function integratedScraper() {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.setExtraHTTPHeaders({
'User-Agent': 'Custom/1.0',
'Accept': 'text/html,application/json'
});
const rateLimiter = new RateLimiter({
requestsPerMinute: 60,
concurrent: 5
});
}
"We've saved a lot of time by using Playwright with Checkly. It only took our team a day or two to evaluate Checkly and determine if it was the right fit for us. It only took me a few minutes to get a login check working and determine if we could login and validate that the CDN was up." - Keegan Donley, Senior Front-End Engineer, Kizen
When using multiple tools, comparing their features can help you choose the best fit for your needs:
Feature | Playwright | Puppeteer | Selenium |
---|---|---|---|
Speed | Fast | Fastest | Slower |
Browser Support | Multiple | Chromium only | All major |
Language Support | Multiple | JavaScript only | Multiple |
Development Experience | Excellent | Excellent | Good |
sbb-itb-65bdb53
Best Practices and Guidelines
When managing dynamic content, these tips can help you fine-tune your real-time scraping efforts.
Performance Optimization
Speed up your Playwright scripts by running tasks in parallel and reducing unnecessary overhead.
// Example: Reusing browser context
const browser = await chromium.launch();
const context = await browser.newContext({
userAgent: 'Custom/1.0',
viewport: { width: 1920, height: 1080 },
ignoreHTTPSErrors: true
});
// Save authentication state for reuse
await context.storageState({ path: 'auth.json' });
You can also improve efficiency by blocking unneeded resources and caching API responses:
// Example: Caching API responses
const responseCache = new Map();
await page.route('**/api/**', async route => {
const cacheKey = route.request().url();
if (responseCache.has(cacheKey)) {
await route.fulfill({ body: responseCache.get(cacheKey) });
return;
}
const response = await route.fetch();
const body = await response.text();
responseCache.set(cacheKey, body);
await route.fulfill({ body });
});
Error Management
Handle temporary issues by implementing retries with exponential backoff:
async function resilientScrape(page, selector, options = {}) {
const {
maxRetries = 3,
baseDelay = 1000,
maxDelay = 10000
} = options;
for (let attempt = 0; attempt < maxRetries; attempt++) {
try {
await page.waitForSelector(selector, { state: 'visible' });
return await page.evaluate(sel => document.querySelector(sel).textContent, selector);
} catch (error) {
if (attempt === maxRetries - 1) throw error;
const delay = Math.min(baseDelay * Math.pow(2, attempt), maxDelay);
console.warn(`Attempt ${attempt + 1} failed. Retrying in ${delay}ms`);
await page.waitForTimeout(delay);
await page.reload();
}
}
}
Legal Requirements
Scraping must comply with legal standards. Here's a quick checklist to guide you:
Requirement | Implementation | Risk Mitigation |
---|---|---|
Rate Limiting | Use dynamic delays | Avoids server overload |
Data Privacy | Remove PII before storage | Ensures GDPR/CCPA compliance |
Terms of Service | Review robots.txt regularly | Maintains lawful access |
Access Methods | Use public APIs when possible | Reduces legal risks |
For large-scale operations, tools like Web Scraping HQ can help you stay compliant. Keep in mind that violations, such as breaching GDPR, can lead to fines of up to €20 million or 4% of global revenue - so staying on the right side of the law is crucial.
Playwright Benefits
Playwright stands out as a robust option for real-time data scraping, offering a modern alternative to older tools. With its asynchronous design, support for multiple browsers, and compatibility with various programming languages, it enables efficient data extraction - especially for dynamic web applications. It also executes tasks faster than Selenium while maintaining full functionality. Its multi-language support makes it easy to integrate into different tech ecosystems.
"Playwright's ability to handle multiple browser contexts and its support for multiple programming languages make it versatile and user-friendly." - Data Journal
These features open up various ways to use Playwright, making it scalable and adaptable to production environments.
Implementation Options
Playwright's strengths allow for different deployment strategies, tailored to fit unique business needs.
Implementation Approach | Best For | Considerations |
---|---|---|
Self-Managed Solution | Teams with strong technical expertise | Requires dedicated resources and infrastructure management |
Hybrid Implementation | Organizations with mixed scraping demands | Combines in-house capabilities with external assistance |
Managed Services | Businesses seeking quick deployment | Offers ready-to-go solutions with built-in compliance and maintenance |
For larger operations, tools like Web Scraping HQ can boost Playwright's capabilities by adding advanced proxy management and anti-bot features - key for ensuring reliability at scale.
With detailed documentation and a growing community, Playwright is a reliable option for long-term projects. Whether you're building a custom setup or leveraging managed services, its flexible architecture supports everything from simple to complex data extraction tasks, making it a go-to choice for real-time data solutions.