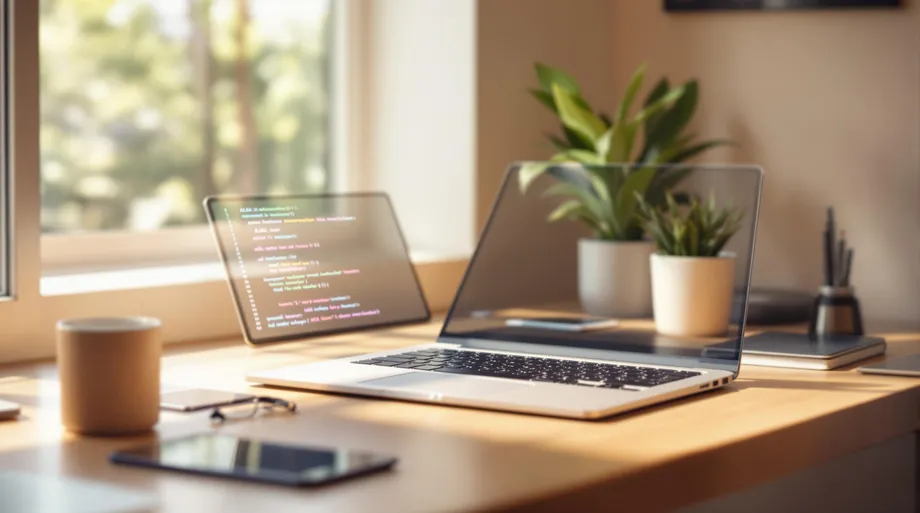
- Harsh Maur
- December 27, 2024
- 7 Mins read
- Scraping
Using Playwright to Intercept and Modify AJAX Requests
Playwright lets you take full control of AJAX requests. You can intercept, modify, or block them entirely. This is useful for testing, debugging, web scraping, and optimizing network behavior.
Here’s what you can do with Playwright:
-
Intercept requests: Use
page.route
to monitor or modify requests in real time. - Modify headers or payloads: Add custom headers or tweak request data.
- Simulate responses: Return mock data without needing server changes.
- Block unnecessary requests: Stop images, fonts, or analytics scripts to improve performance.
- Handle dynamic content: Wait for specific requests or elements to ensure data is fully loaded.
Example: Modify an API response easily:
page.route('**/api/data', (route) => {
route.fulfill({
status: 200,
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ data: 'Modified data' }),
});
});
With these tools, you can streamline workflows, test edge cases, and extract data efficiently.
Understanding AJAX Requests and Playwright
What Are AJAX Requests?
AJAX, short for Asynchronous JavaScript and XML, allows web applications to exchange data with servers in the background without reloading the page. This enables real-time interactions, like 'liking' a post or filtering search results, to happen seamlessly. Its ability to load dynamic content in the background makes it a key component in advanced web scraping and automation workflows.
With that in mind, let's look at how Playwright simplifies working with AJAX.
How Playwright Handles AJAX
Playwright offers several tools to manage AJAX requests effectively. Here are three main methods:
Method | What It Does |
---|---|
waitForRequest |
Observe outgoing requests, such as tracking API calls before they're sent. |
waitForResponse |
Monitors incoming responses, useful for validating server replies. |
page.route |
Intercepts and modifies requests, allowing custom behavior. |
These features enable Playwright to interact with AJAX-driven applications by monitoring and controlling network communications in real-time. For example, the page.route
method lets you intercept requests and even modify them. Here's how it works:
// Example: Modifying an API response
page.route('**/api/data', (route) => {
route.fulfill({
status: 200,
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ data: 'Modified data' }),
});
});
When dealing with dynamic content, Playwright's ability to wait for specific requests ensures your scripts capture every important update. This makes it especially useful for scenarios like extracting real-time data or testing applications with frequent server interactions.
Mocking, Intercepting, Monitoring and Waiting for network requests with Playwright
Intercepting AJAX Requests in Playwright
Intercepting AJAX requests is a useful technique, especially in tasks like web scraping where controlling network traffic is essential for accurate data collection.
Using page.route
Intercept Requests
Playwright's page.route
allows you to monitor and manage network requests. Here's a simple example:
await page.route('**/api/posts', async route => {
const request = route.request();
console.log(`Intercepted request to: ${request.url()}`);
await route.continue(); // Proceeds with the original request
});
With page.route
, you can define a URL pattern and a handler function for matching requests. Use wildcards (**
) for broader patterns, or specify exact URLs for more targeted interception.
Modifying Request Headers and Payloads
You can also tweak headers or payloads before sending requests to the server using route.continue
. Here's how:
await page.route('**/api/secure-endpoint', async route => {
const headers = route.request().headers();
headers['Authorization'] = 'Bearer your-custom-token';
headers['Custom-Header'] = 'test-value';
await route.continue({
headers: headers,
postData: JSON.stringify({
modified: true,
timestamp: Date.now()
})
});
});
Need to block specific requests? That’s possible too:
await page.route('**/*.{png,jpg,jpeg}', route => {
route.abort(); // Blocks image requests
});
Intercepting AJAX requests helps you:
- Simulate edge cases
- Test error handling
- Isolate tests from external dependencies
- Manage network behavior more effectively
With these basics covered, you can dive deeper into advanced techniques for handling dynamic content and fine-tuning network interactions.
sbb-itb-65bdb53
Advanced AJAX Manipulation Techniques
When using Playwright for web automation or data scraping, dealing with dynamic content and managing requests efficiently is key. Here’s a closer look at some advanced techniques to enhance your workflow.
Managing Dynamic Content
Dynamic content often requires precise coordination between request handling and page interactions. Here's an example of how to manage this using Playwright:
await page.route('**/api/dynamic-data', async route => {
const request = route.request();
await route.continue({
headers: {
...request.headers(),
'Cache-Control': 'no-cache'
}
});
// Wait for the dynamic content to load
await page.waitForSelector('.dynamic-content', {
state: 'attached',
timeout: 5000
});
});
// Wait for JavaScript execution to complete
await page.waitForFunction(() => {
return window.dataLoadingComplete === true;
});
In this example, window.dataLoadingComplete
Is a custom variable that signals when the page's data loading is finished. If the target site doesn’t use such a flag, you can implement your logic to track loading progress.
Key methods to manage dynamic content include:
-
page.waitForSelector()
: Ensures that specific elements are present on the page. -
page.waitForFunction()
: Checks if custom JavaScript conditions are met. -
Timeouts: Use options
timeout: 5000
to handle delays effectively. - Network monitoring: Observe requests to confirm when data has fully loaded.
These approaches are particularly helpful for AJAX-heavy websites where content is updated dynamically based on server responses.
Blocking Unnecessary Requests
To optimize performance, you can block certain types of requests or specific domains. Here’s an example:
await page.route('**/*', async route => {
const url = route.request().url();
const resourceType = route.request().resourceType();
// Block requests to analytics or tracking domains
if (url.includes('analytics.com') || url.includes('tracking.com')) {
await route.abort();
return;
}
// Block non-essential resource types
const blockedTypes = ['image', 'stylesheet', 'font'];
blockedTypes.includes(resourceType) ?
await route.abort() :
await route.continue();
});
This method helps you:
- Cut down on bandwidth usage by skipping unnecessary assets.
- Speed up page loading times.
- Focus on collecting only the data you need.
- Improve the overall efficiency of your scraping process.
Using Webscraping HQ for Managed Services
Running large-scale web scraping operations can be tough, especially when dealing with complex requirements. Managed services like those from Webscraping HQ offer a reliable way to handle these challenges, working alongside tools like Playwright to deliver results.
Services Offered by Webscraping HQ
Web Scraping HQ specializes in extracting and delivering structured data tailored to specific needs. Here's a quick look at what they offer:
Data Type | Use Cases | Delivery Format |
---|---|---|
Product Data | Tracking prices and inventory | JSON/CSV |
Company Data | Lead generation and research | Structured feeds |
Real Estate Data | Analyzing property details | Custom schema |
Job Posting Data | Recruitment insights | Automated feeds |
Vehicle Data | Market analysis | JSON/CSV |
Their double-layer QA process ensures high-quality data, even for sites with AJAX-driven content. They also tackle challenges like dynamic pages, authentication, and rate limits effectively.
When to Choose Managed Web Scraping
Managed services are a smart choice in situations like these:
Handling Technical Complexity
- Dealing with frequent changes in AJAX-based websites
- Managing multiple authentication layers
- Overcoming advanced rate-limiting systems
Scaling Up Operations
The Standard plan ($449/month) offers structured data with automated QA, while Custom solutions (starting at $999/month) provide enterprise-grade support with fast delivery - often within 24 hours.
Ensuring Compliance
Web Scraping HQ takes care of legal and ethical concerns, including:
- Respecting robots.txt rules
- Staying within rate limits
- Following data protection laws
- Addressing terms of service requirements
Conclusion
Learning how to intercept and modify AJAX requests with Playwright is a key skill for today's web developers and testers. With Playwright's page.route
method, you can take control of HTTP requests and responses, making it easier to create precise testing scenarios and streamline data extraction workflows.
Using Playwright, developers can simulate server responses without touching backend systems, optimize network traffic, and ensure consistent data collection in various scenarios. These features are particularly useful for testing, improving performance, and maintaining reliable data workflows.
For businesses needing scalable and compliant data extraction, managed services can be a strong alternative. Web Scraping HQ offers solutions that range from standard plans to custom enterprise support, ensuring businesses can meet their unique needs.
As web development grows more complex, tools like Playwright are becoming indispensable for handling AJAX-heavy websites. Whether you're testing, extracting data, or managing dynamic content, mastering these techniques is essential for building efficient, reliable web solutions.
To succeed, it's important to understand both the technical capabilities of Playwright and the broader context of web architecture. Combining these skills with the right tools and services allows developers to tackle modern web challenges with confidence.
FAQs
What is an intercept request?
Intercepting requests in Playwright is a handy way to manage network interactions. It lets developers track network traffic, tweak headers or payloads, return mock responses, or block unnecessary requests to improve performance. Here's a simple example using the page.route
method in Playwright:
await page.route('**/api/data', async route => {
await route.continue({
headers: {
...route.request().headers(),
'custom-header': 'test-value'
}
});
});
Some common uses include:
- Testing: Mimic server responses without changing the backend.
- Performance: Cut down on bandwidth by blocking unneeded requests.
- Web Scraping: Filter data for easier and faster extraction.
- Debugging: Keep track of API interactions in real time.
The playwright gives you control over both the request and response phases, expanding on the interception methods discussed earlier. For more examples, like modifying headers or handling various request types, check out the "Intercepting AJAX Requests in Playwright" section.