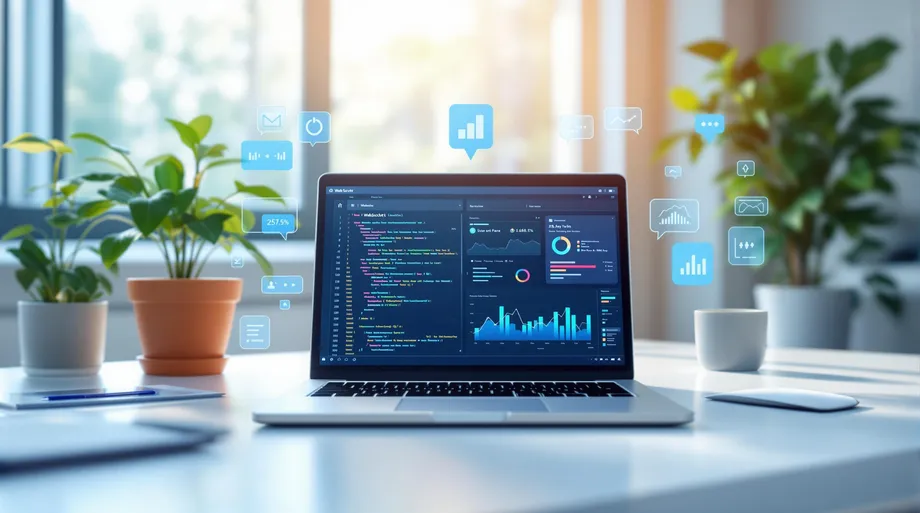
- Harsh Maur
- December 1, 2024
- 5 Mins read
- Scraping
WebSocket Data Extraction with Playwright
Want to extract real-time WebSocket data effortlessly? The playwright makes it simple and efficient. Here's what you'll learn:
- What WebSockets are: A technology enabling continuous, two-way communication for live updates like chat apps or financial data.
- Challenges in WebSocket scraping: Handling persistent connections, encrypted data, and dynamic updates.
- Why Playwright is ideal: It automates WebSocket monitoring, stabilizes connections, and processes live data efficiently.
- How to set it up: Step-by-step guide on installing Playwright, launching browsers, and capturing WebSocket messages.
- Advanced techniques: Routing WebSocket requests, handling dynamic content, and managing large-scale data streams.
This guide provides everything you need to extract WebSocket data effectively using Playwright, from setup to advanced performance tips.
How to check WebSocket requests in Browser
Setting Up Playwright for WebSocket Data
To use Playwright for extracting WebSocket data, you'll need to install and configure it correctly. Follow these steps to prepare for monitoring WebSocket connections.
Installing Playwright
First, install Playwright via npm:
npm i -D playwright
Then, create a JavaScript file and import Playwright with:
const playwright = require('playwright');
Launching a Browser with Playwright
Playwright supports several browser engines, including Chromium, Firefox, and WebKit, making it adaptable to different needs. Below is a basic setup to launch a browser:
const browser = await playwright.chromium.launch({
headless: true
});
Running the browser in headless mode is ideal for WebSocket data extraction. It allows automated real-time monitoring without the overhead of a visible browser interface.
Configuring WebSocket Monitoring
To monitor WebSocket activity, use the following setup:
page.on('websocket', (ws) => {
ws.on('framereceived', (frame) => {
console.log(frame.payloadData);
});
});
To prevent missing any initial WebSocket activity, use the waitForEvent('websocket')
method:
await page.waitForEvent('websocket');
For more control, you can intercept specific WebSocket requests using Playwright's routing feature:
await page.route('**/ws-endpoint', (route) => {
// Custom handling logic
route.continue();
});
This setup ensures stable connections and efficient processing, laying the groundwork for extracting real-time WebSocket data.
Once everything is configured, you can start navigating web pages and capturing live data from WebSocket connections.
How to Extract WebSocket Data with Playwright
Navigating to the Target Web Page
To get started, open the target webpage using Playwright:
const { chromium } = require("playwright");
async function setupWebSocketExtraction() {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.goto("https://example.com/websocket-page");
}
Add error handling to manage potential navigation problems:
try {
await page.goto("https://example.com/websocket-page", {
waitUntil: 'networkidle',
timeout: 30000
});
} catch (error) {
console.error("Navigation failed:", error);
}
Listening to WebSocket Messages
Use Playwright's event listeners to track WebSocket activity in real-time:
page.on('websocket', (ws) => {
console.log(`WebSocket opened: ${ws.url()}`);
ws.on('framereceived', (frame) => {
console.log(`Received WebSocket message: ${frame.payload}`);
});
ws.on('close', () => {
console.log('WebSocket closed');
});
});
After capturing messages, organizing and storing them is key for analysis.
Handling and Storing WebSocket Data
To process and save WebSocket messages, use this approach:
const fs = require('fs');
page.on('websocket', (ws) => {
ws.on('framereceived', async (frame) => {
try {
const data = JSON.parse(frame.payload);
// Generate a unique filename using a timestamp
const filename = `websocket_data_${Date.now()}.json`;
// Save to file
await fs.promises.writeFile(
filename,
JSON.stringify(data, null, 2)
);
} catch (error) {
console.error("Error processing message:", error);
}
});
});
For high-frequency data streams, use buffering to avoid memory overload:
let messageBuffer = [];
const BUFFER_SIZE = 100;
page.on('websocket', (ws) => {
ws.on('framereceived', (frame) => {
messageBuffer.push(JSON.parse(frame.payload));
if (messageBuffer.length >= BUFFER_SIZE) {
saveBufferedData();
messageBuffer = [];
}
});
});
This method ensures smooth performance even during intense data streams.
sbb-itb-65bdb53
Advanced WebSocket Data Extraction Techniques
Using WebSocket Routing in Playwright
Playwright's WebSocket routing lets you filter and tweak WebSocket messages with precision. Here's an example of how to set up targeted routing:
await page.route('**/*', async (route, request) => {
if (request.resourceType() === 'websocket') {
const ws = await route.continue();
ws.on('framereceived', (frame) => {
if (frame.payload.includes('target_data')) {
processWebSocketData(frame.payload);
}
});
}
});
This approach allows you to intercept specific WebSocket messages and process the data you need.
Handling Content That Changes Dynamically
Dynamic content requires careful handling to ensure accurate data collection. Use waiting strategies like these:
await page.waitForSelector('#dynamic-content', {
state: 'visible',
timeout: 30000
});
page.waitForEvent('websocket').then(async (ws) => {
await page.waitForFunction(() => {
return document.querySelector('#content')?.dataset.updated === 'true';
});
});
By combining WebSocket routing with dynamic content handling, you can maintain data reliability even in rapidly changing environments.
Improving Performance for Large-Scale Data
When dealing with large data volumes, efficiency is key. Use batching, retries, and memory management to streamline the process:
const BATCH_SIZE = 1000;
let messageQueue = [];
page.on('websocket', (ws) => {
ws.on('framereceived', async (frame) => {
try {
messageQueue.push(JSON.parse(frame.payload));
if (messageQueue.length >= BATCH_SIZE) {
await processBatch(messageQueue);
messageQueue = [];
if (global.gc) {
global.gc(); // Trigger garbage collection to free memory
}
}
} catch (error) {
console.error('Processing error:', error);
}
});
});
async function processBatch(batch, retries = 3) {
for (let attempt = 1; attempt <= retries; attempt++) {
try {
await saveBatchToDatabase(batch);
break;
} catch (error) {
if (attempt === retries) throw error;
await new Promise(resolve => setTimeout(resolve, 1000 * attempt));
}
}
}
Batching messages minimizes memory usage, retries ensure data is saved even if errors occur, and garbage collection helps manage memory during intensive operations. These techniques make Playwright a reliable tool for handling large-scale WebSocket data extraction.
Conclusion
Key Takeaways
Playwright stands out when it comes to extracting WebSocket data. It handles real-time data collection and dynamic content with ease, all while ensuring stable performance. Its automation features simplify even the most challenging data extraction tasks, making it a solid choice for maintaining both efficiency and reliability.
Practical Tips for Better Data Extraction
For dependable WebSocket data extraction, focus on these essentials: validate your data regularly, set up robust error-handling mechanisms, and batch process high-frequency streams. These steps are especially useful for large-scale operations, helping to keep data accurate and workflows efficient. While Playwright offers a strong foundation, external tools can complement its functionality for more advanced needs.
Helpful Resources and Tools
If you're tackling complex WebSocket data extraction projects, consider using services like Webscraping HQ. They provide managed solutions that work alongside Playwright, helping ensure compliance and maintaining data quality. Combining Playwright's automation with professional expertise allows you to handle challenging tasks, especially those involving real-time data and dynamic content, with greater ease.
FAQs
Does the Playwright use WebSocket?
Yes, Playwright supports WebSocket functionality through its event listeners and routing tools. When working with WebSockets in Playwright, you have two main options:
- Direct Server Interaction: Intercept and modify WebSocket messages while connected to servers. This is useful for collecting and analyzing real-time data.
-
Message Monitoring and Control: Use Playwright's WebSocket API to track and manipulate WebSocket communications. This can help with tasks like:
- Monitoring live data streams
- Debugging server-client interactions
- Dynamically altering messages
For examples of methods like waitForEvent()
and route()
, check earlier sections on WebSocket monitoring and routing.